- Step 1: Set Up Your Laravel Project
- Step 2: Create a Route for the Sitemap
- Step 3: Create the Sitemap Controller
- Step 4: Define the URLs for Your Sitemap
- Step 5: Generate the Sitemap XML
- Step 6: Return the Sitemap
- Step 7: Test Your Sitemap
A sitemap XML file is a critical component for improving your website's SEO by helping search engines crawl and index your content efficiently. While there are numerous packages available to generate sitemaps in Laravel, creating one manually gives you full control over its structure and content. This tutorial will guide you through the process of manually generating a sitemap XML for your Laravel site.
Step 1: Set Up Your Laravel Project
Ensure your Laravel project is set up and running. If not, you can create a new Laravel project using Composer:
composer create-project --prefer-dist laravel/laravel sitemap-example
Step 2: Create a Route for the Sitemap
First, create a route in routes/web.php
that will handle the sitemap request:
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\SitemapController;
Route::get('/sitemap.xml', [SitemapController::class, 'index']);
Step 3: Create the Sitemap Controller
Next, create a controller that will generate the sitemap XML. Run the following Artisan command:
php artisan make:controller SitemapController
In the newly created SitemapController.php
, add the following code:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\URL;
use Carbon\Carbon;
class SitemapController extends Controller
{
public function index()
{
// Array to store URLs
$urls = [];
// Add static pages
$urls[] = [
'loc' => URL::to('/'),
'lastmod' => Carbon::now()->toAtomString(),
'changefreq' => 'daily',
'priority' => '1.0'
];
$urls[] = [
'loc' => URL::to('/about'),
'lastmod' => Carbon::now()->toAtomString(),
'changefreq' => 'monthly',
'priority' => '0.8'
];
// Add dynamic pages
$posts = \App\Models\Post::all();
foreach ($posts as $post) {
$urls[] = [
'loc' => URL::to('/posts/' . $post->slug),
'lastmod' => $post->updated_at->toAtomString(),
'changefreq' => 'weekly',
'priority' => '0.9'
];
}
// Generate XML
$xml = $this->generateSitemap($urls);
return response($xml, 200)
->header('Content-Type', 'application/xml');
}
private function generateSitemap($urls)
{
$xml = new \SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><urlset/>');
$xml->addAttribute('xmlns', 'http://www.sitemaps.org/schemas/sitemap/0.9');
foreach ($urls as $url) {
$urlTag = $xml->addChild('url');
$urlTag->addChild('loc', $url['loc']);
$urlTag->addChild('lastmod', $url['lastmod']);
$urlTag->addChild('changefreq', $url['changefreq']);
$urlTag->addChild('priority', $url['priority']);
}
return $xml->asXML();
}
}
Step 4: Define the URLs for Your Sitemap
In the SitemapController
, we define both static and dynamic URLs. For static URLs, we manually add them to the $urls
array. For dynamic URLs, such as blog posts, we retrieve the entries from the database and append them to the $urls
array.
Step 5: Generate the Sitemap XML
The generateSitemap
method converts the array of URLs into an XML structure using PHP's SimpleXMLElement
. This method iterates over the URLs and adds them to the XML document with appropriate tags (<loc>
, <lastmod>
, <changefreq>
, and <priority>
).
Step 6: Return the Sitemap
The index
method in the SitemapController
prepares the URLs, calls the generateSitemap
method to create the XML, and returns the XML response with the correct Content-Type
.
Step 7: Test Your Sitemap
To test your sitemap, navigate to http://yourdomain.com/sitemap.xml
in your browser. You should see an XML document listing your site's URLs.
Manually generating a sitemap in Laravel without using packages gives you full control over its content and structure. By following this guide, you can ensure that your sitemap accurately reflects the content on your site, thereby improving your SEO and helping search engines crawl your site more efficiently. This approach is flexible, allowing you to customize the sitemap generation process to fit your specific needs.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
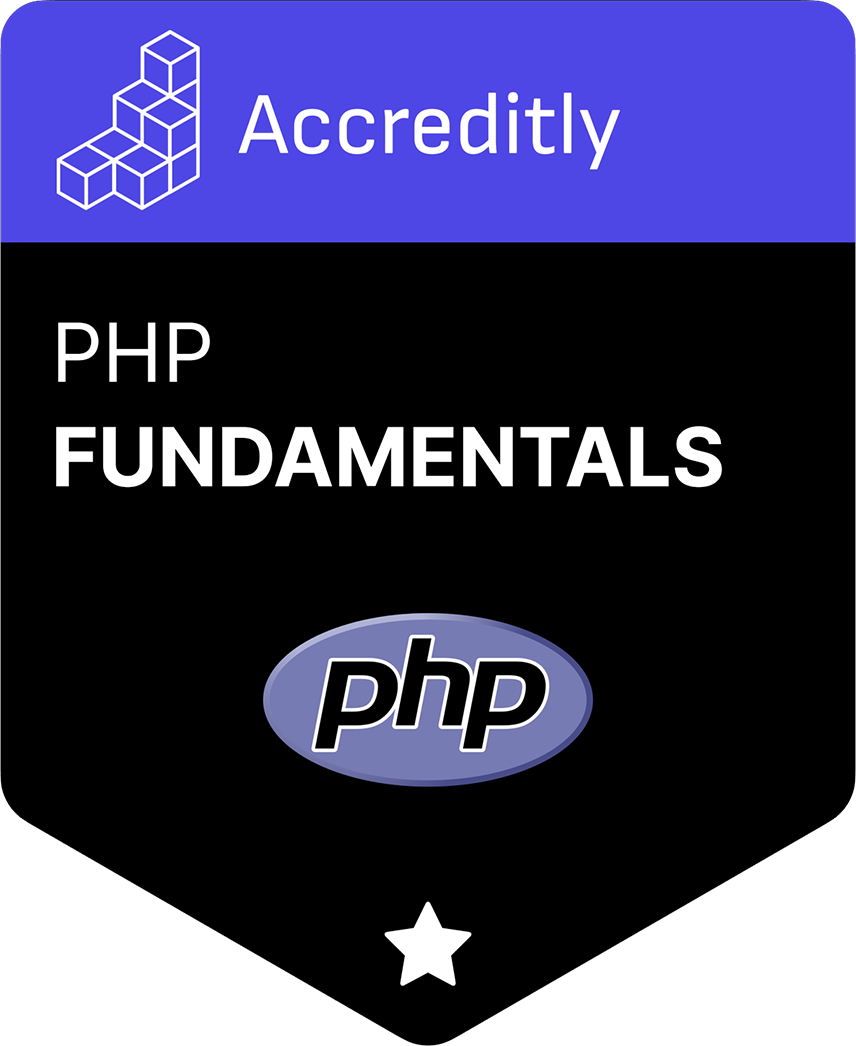