- Prerequisites
- Step 1: Setting Up Localization Configuration
- Step 2: Creating Translation Files
- Step 3: Defining Localized Routes
- Step 4: Using Localized Routes in Views
- Step 5: Middleware for Language Detection
- Final take
Creating a multilingual website involves more than just translating text. To fully support multiple languages, you also need to localise your routes. Laravel, a popular PHP framework, provides robust support for localization, including route localization. This article will guide you through the process of localising routes in Laravel, ensuring your application can serve users in their preferred languages.
Prerequisites
Before we begin, ensure you have the following:
- A Laravel application set up. If you haven't set up a Laravel project yet, you can follow the Laravel installation guide.
- Basic understanding of Laravel routing and middleware.
Step 1: Setting Up Localization Configuration
Laravel’s localization configuration is stored in the config/app.php
file. Here, you can define the default locale and available locales for your application.
Configuring Locales
Open config/app.php
and set the locale
and fallback_locale
options:
'locale' => 'en',
'fallback_locale' => 'en',
Next, define the supported locales. You can add this configuration at the end of the config/app.php
file:
'supported_locales' => ['en', 'fr', 'de'],
Step 2: Creating Translation Files
Laravel uses translation files stored in the resources/lang
directory. Each language should have its own subdirectory with translation files.
Example Translation Files
Create directories for each language you want to support:
mkdir -p resources/lang/en
mkdir -p resources/lang/fr
mkdir -p resources/lang/de
Inside each language directory, create a routes.php
file for route translations:
// resources/lang/en/routes.php
return [
'welcome' => 'welcome',
];
// resources/lang/fr/routes.php
return [
'welcome' => 'bienvenue',
];
// resources/lang/de/routes.php
return [
'welcome' => 'willkommen',
];
Step 3: Defining Localized Routes
Now, let’s define the localized routes in the routes/web.php
file. Laravel does not provide native support for route localization, so you need to implement it manually or use a package like mcamara/laravel-localization
.
Installing the Laravel Localization Package
To simplify the localization process, you can use the mcamara/laravel-localization
package:
composer require mcamara/laravel-localization
Publish the configuration file:
php artisan vendor:publish --provider="Mcamara\LaravelLocalization\LaravelLocalizationServiceProvider"
Configuring the Package
Open config/laravellocalization.php
and set the supported locales:
'supportedLocales' => [
'en' => ['name' => 'English'],
'fr' => ['name' => 'French'],
'de' => ['name' => 'German'],
],
Defining Routes with Localization
In routes/web.php
, wrap your routes with the LaravelLocalization
middleware:
use Mcamara\LaravelLocalization\Facades\LaravelLocalization;
Route::group(['prefix' => LaravelLocalization::setLocale(), 'middleware' => ['localeSessionRedirect', 'localizationRedirect', 'localeViewPath']], function () {
Route::get(LaravelLocalization::transRoute('routes.welcome'), function () {
return view('welcome');
})->name('welcome');
});
Step 4: Using Localized Routes in Views
To generate URLs for localized routes in your views, use the localize_url
helper function provided by the laravel-localization
package.
<a href="{{ LaravelLocalization::localizeUrl(route('welcome')) }}">
@lang('routes.welcome')
</a>
This ensures that the URLs are correctly localized based on the current language.
Step 5: Middleware for Language Detection
To automatically detect and set the user's preferred language, you can create middleware that checks the Accept-Language
header or a query parameter.
Creating Middleware
Generate middleware using Artisan:
php artisan make:middleware SetLocale
Implementing Middleware Logic
Open app/Http/Middleware/SetLocale.php
and implement the logic to set the locale:
namespace App\Http\Middleware;
use Closure;
use Illuminate\Support\Facades\App;
use Illuminate\Support\Facades\Config;
use Illuminate\Support\Facades\Session;
class SetLocale
{
public function handle($request, Closure $next)
{
if (Session::has('locale')) {
$locale = Session::get('locale');
} else {
$locale = $request->getPreferredLanguage(Config::get('app.supported_locales'));
Session::put('locale', $locale);
}
App::setLocale($locale);
return $next($request);
}
}
Register the middleware in app/Http/Kernel.php
:
protected $middlewareGroups = [
'web' => [
// other middlewares...
\App\Http\Middleware\SetLocale::class,
],
];
Final take
Localizing routes in Laravel enhances the user experience by providing content in the user’s preferred language. By configuring localization settings, creating translation files, defining localized routes, and using middleware to detect the user's language, you can build a multilingual Laravel application efficiently.
For more details, refer to the official documentation:
By following these steps, you can ensure that your Laravel application is fully localized, providing a seamless experience for users around the world.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
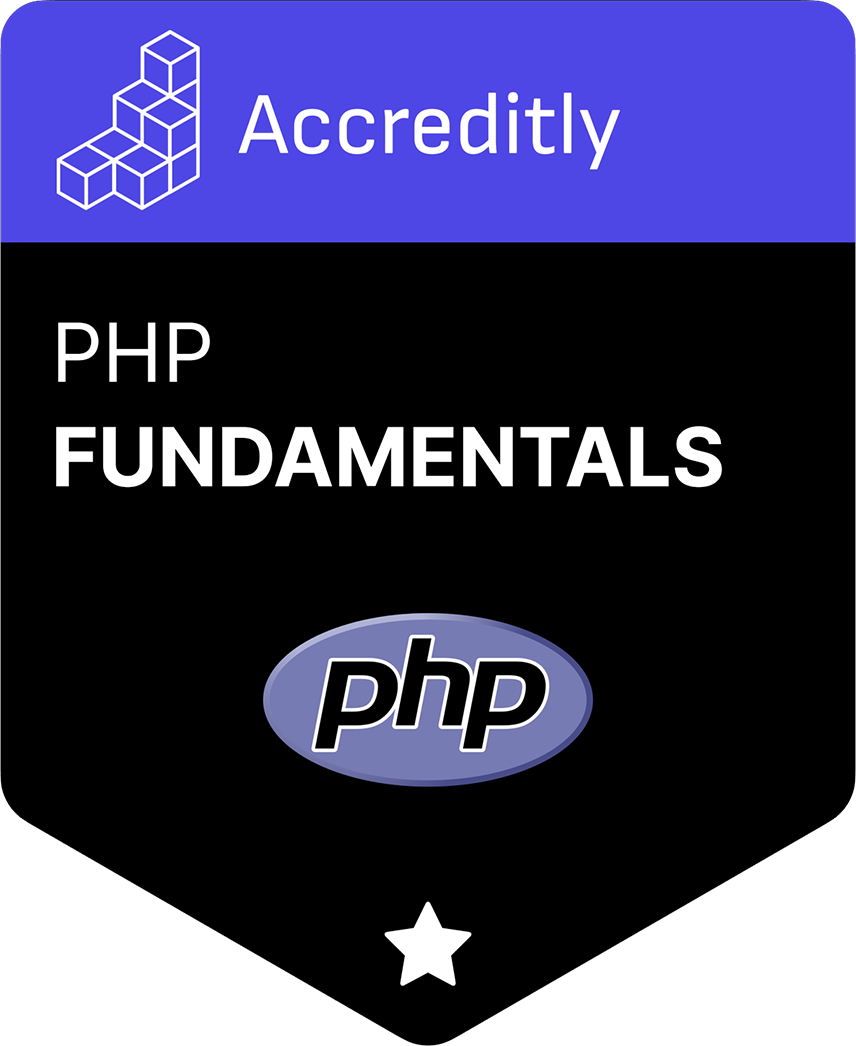