- Prerequisites
- Step 1: Install Stripe's PHP SDK
- Step 2: Initialize Stripe
- Step 3: Create a Charge
- Step 4: Handle Exceptions
- Profit
Stripe is a renowned online payment processing platform that offers a wide range of services and solutions for businesses. In this tutorial, we'll delve into how to leverage Stripe's PHP SDK to take payments.
Prerequisites
Before we begin, you'll need the following:
- A Stripe account: If you don't have one, sign up here.
- Your Stripe API Keys: These are available in your Stripe Dashboard.
- Composer: You'll need this to install Stripe's PHP SDK.
Step 1: Install Stripe's PHP SDK
Let's begin by installing Stripe's PHP SDK using Composer. Open your terminal and run:
composer require stripe/stripe-php
This command installs the Stripe PHP SDK into your project.
Step 2: Initialize Stripe
Next, initialize Stripe in your PHP file. Include the following code:
require_once('vendor/autoload.php');
\Stripe\Stripe::setApiKey('your-stripe-secret-key');
Replace 'your-stripe-secret-key'
with your actual Stripe secret key. We always recommend using .env
variables to store these values so you can use different Stripe environments in your different development environments. Stripe offers Test credentials for development and testing/QA/staging, and live credentials for production environments.
Step 3: Create a Charge
Now that Stripe is set up, we can create a charge. A charge represents a payment in Stripe.
Here's how you can create a charge:
try {
$charge = \Stripe\Charge::create(array(
"amount" => 2000, // Amount in cents
"currency" => "usd",
"source" => "tok_mastercard", // Replace with your test token
"description" => "Test payment."
));
echo 'Charge successful, charge ID: ' . $charge->id;
} catch(\Stripe\Error\Card $e) {
// The card was declined
echo 'Error, payment failed.';
}
In this code, we're creating a charge of $20.00 USD. The "source" field should be replaced with your test token. You can get a list of test tokens from the Stripe documentation on testing.
Attaining a token in production is going to be a little more complex than copying a test token from Stripe's docs. The way to attain a token differs depending on what you are trying to do, and isn't done in PHP. Instead, this is usually done in JavaScript, and Stripe offer a number of products to make this easier. Stripe Elements is one such product, which is an embeddable UI component that takes a customer's card details, sends them to Stripe's server and returns a token for you to create a charge. Stripe Elements is great because it looks like it's part of your website, but it's actually a number of iframes that load content from Stripe's servers, saving you the security headache that comes with handling card details.
A common gotcha is that Stripe charges everything in the lowest denomination of that currency. In the case of USD that is cents. This often doesn't match up with how currency is stored in a database (you may store it as a DECIMAL
, for example), so ensure you convert it if necessary.
Step 4: Handle Exceptions
You'll notice that we wrapped our charge code in a try-catch
block. This is to handle cases where the card is declined. There are several other exceptions that you may need to handle, such as RateLimit
, InvalidRequest
, Authentication
, ApiConnection
, ApiErrorException
, and Base
. You can find more about these exceptions in the Stripe API documentation.
Profit
That's it, that's all you need to do to charge a card in Stripe.
One thing this tutorial doesn't cover is the ability to attain a token. Entering card details on a browser is a complex topic due to obvious security concerns. Stripe has a number of solutions to handle this and return a token, one of which is Stripe Elements.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
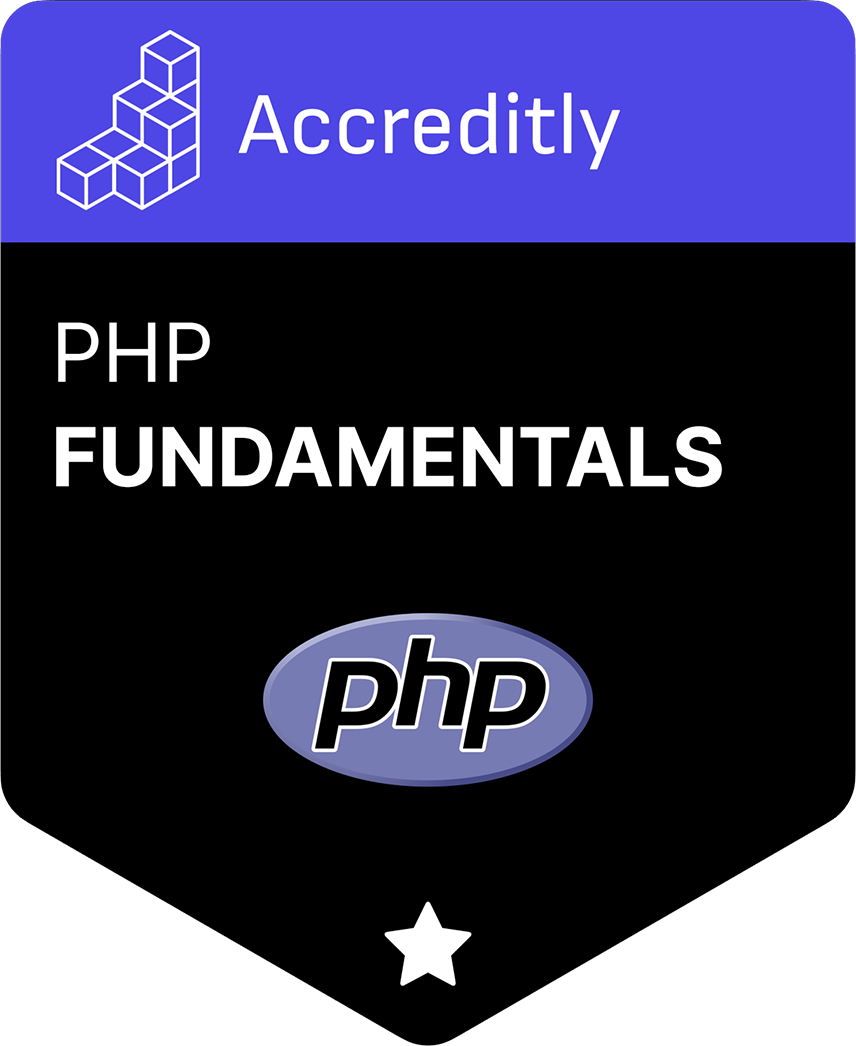