- Getting Started
- Prerequisites
- Installation
- Making API Requests
- Setting Up the Client
- Text Generation
- Example: Generating Code Snippets
- Text Summarization
- Language Translation
- Question Answering
- Code Examples and Error Handling
- Handling API Errors
- Advanced Code Example: Chatbot
The OpenAI API provides powerful tools for natural language processing, including text generation, translation, summarization, and more. PHP developers can leverage these capabilities by integrating the OpenAI API into their applications. This article will guide you through using the OpenAI API in PHP, with plenty of examples for each API endpoint.
Getting Started
Before diving into the code, make sure you have an API key from OpenAI. You can obtain one by signing up on the OpenAI website.
Prerequisites
- PHP 7.4 or higher. (8.3 or above recommended)
- Composer installed for managing dependencies.
- A web server or a local development environment like XAMPP or MAMP.
Installation
First, you need to install the Guzzle HTTP client, which we will use to make requests to the OpenAI API. Run the following command in your project directory:
composer require guzzlehttp/guzzle
Making API Requests
Setting Up the Client
Let's start by setting up a simple PHP script to handle API requests. Create a file named openai.php
and include the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client([
'base_uri' => 'https://api.openai.com/v1/',
'headers' => [
'Authorization' => 'Bearer YOUR_API_KEY',
'Content-Type' => 'application/json'
]
]);
function callOpenAI($endpoint, $data) {
global $client;
$response = $client->post($endpoint, [
'json' => $data
]);
return json_decode($response->getBody(), true);
}
?>
Replace YOUR_API_KEY
with your actual OpenAI API key. The callOpenAI
function will handle the API requests.
Text Generation
One of the most common uses of the OpenAI API is text generation. Here's how to generate text using the davinci
model:
<?php
// Include the previous setup code
$data = [
'model' => 'text-davinci-003',
'prompt' => 'Write a short story about a robot learning to love.',
'max_tokens' => 150
];
$response = callOpenAI('completions', $data);
echo $response['choices'][0]['text'];
?>
Example: Generating Code Snippets
You can also use the API to generate code snippets. Here's an example of generating a Python function to sort a list:
<?php
// Include the previous setup code
$data = [
'model' => 'code-davinci-002',
'prompt' => 'Write a Python function to sort a list of integers.',
'max_tokens' => 100
];
$response = callOpenAI('completions', $data);
echo $response['choices'][0]['text'];
?>
Text Summarization
To summarize a piece of text, you can use the same davinci
model with a different prompt. Here's an example:
<?php
// Include the previous setup code
$text = "OpenAI provides powerful AI tools that help developers build applications with natural language understanding, text generation, and more.";
$data = [
'model' => 'text-davinci-003',
'prompt' => 'Summarize the following text: ' . $text,
'max_tokens' => 50
];
$response = callOpenAI('completions', $data);
echo $response['choices'][0]['text'];
?>
Language Translation
The OpenAI API can also be used for language translation. Here's an example of translating English text to French:
<?php
// Include the previous setup code
$data = [
'model' => 'text-davinci-003',
'prompt' => 'Translate the following English text to French: "Hello, how are you?"',
'max_tokens' => 60
];
$response = callOpenAI('completions', $data);
echo $response['choices'][0]['text'];
?>
Question Answering
You can use the API to answer questions based on a provided context. Here's how:
<?php
// Include the previous setup code
$context = "The Eiffel Tower is one of the most famous landmarks in Paris, France. It was constructed between 1887 and 1889.";
$data = [
'model' => 'text-davinci-003',
'prompt' => 'Answer the following question based on the context: ' . $context . ' Question: When was the Eiffel Tower constructed?',
'max_tokens' => 50
];
$response = callOpenAI('completions', $data);
echo $response['choices'][0]['text'];
?>
Code Examples and Error Handling
Handling API Errors
It's important to handle potential errors when making API requests. Here's an updated version of the callOpenAI
function with error handling:
<?php
function callOpenAI($endpoint, $data) {
global $client;
try {
$response = $client->post($endpoint, [
'json' => $data
]);
return json_decode($response->getBody(), true);
} catch (Exception $e) {
return ['error' => $e->getMessage()];
}
}
?>
Advanced Code Example: Chatbot
Let's create a simple chatbot using the OpenAI API. This example demonstrates a more complex interaction with the API:
<?php
// Include the previous setup code
$messages = [
["role" => "system", "content" => "You are a helpful assistant."],
["role" => "user", "content" => "Who won the world series in 2020?"],
["role" => "assistant", "content" => "The Los Angeles Dodgers won the World Series in 2020."],
["role" => "user", "content" => "Where was it played?"]
];
$data = [
'model' => 'gpt-3.5-turbo',
'messages' => $messages,
'max_tokens' => 150
];
$response = callOpenAI('chat/completions', $data);
echo $response['choices'][0]['message']['content'];
?>
In this example, the chatbot is designed to continue the conversation based on previous messages. This approach is useful for creating interactive applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
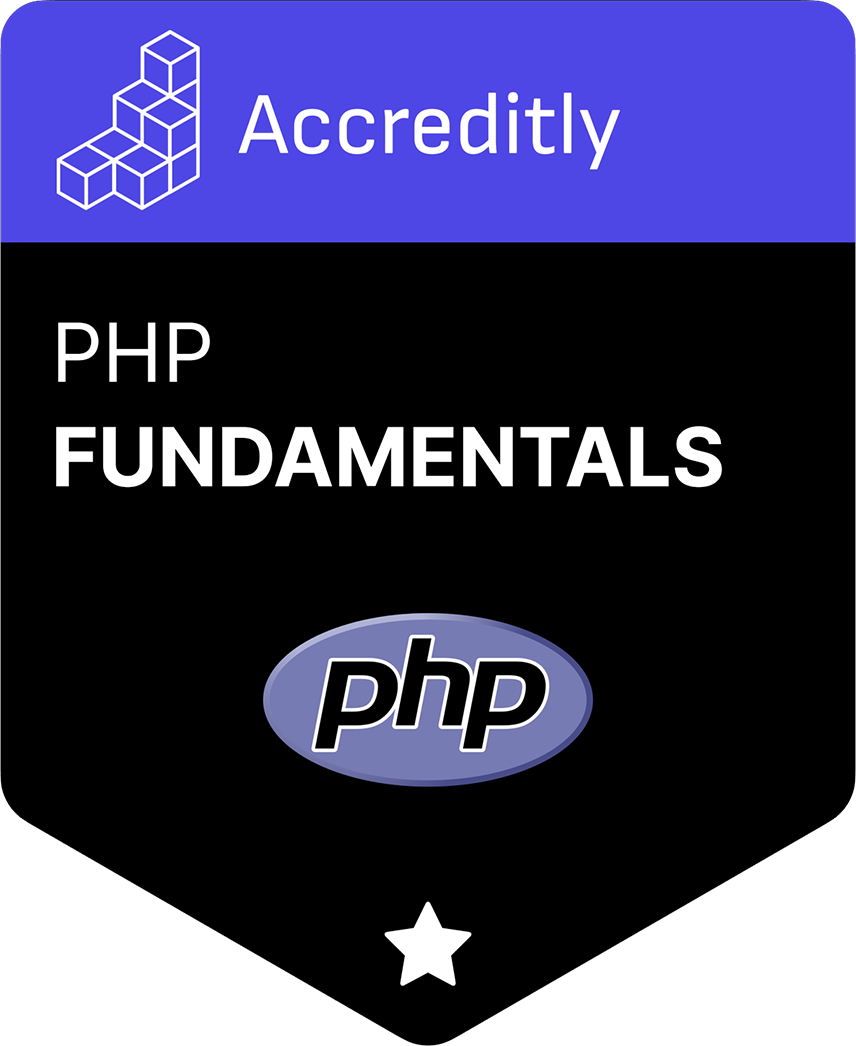
Related articles
Tutorials WordPress PHP Tooling
Optimizing WordPress YouTube Embeds with Lite YouTube Embed
Learn how to enhance your WordPress site's performance by replacing the native YouTube embed with Lite YouTube Embed. This guide takes you through the steps to override the default oEmbed behaviour for YouTube videos.