- What is optional in Laravel?
- Basic Usage of optional
- Advanced Usage
- Using optional with Closure
- Chainability
- When to Use optional
In Laravel, dealing with null values is a common challenge, especially when you're trying to access properties or call methods on an object that might not exist. Laravel's optional
helper function provides a clean, fluent way to handle these situations gracefully. Let's explore how to use optional
to enhance the robustness and readability of your Laravel application.
optional
in Laravel?
What is The optional
helper function is a convenience provided by Laravel for handling cases where a variable might be null
. It allows you to access properties or call methods on an object without first checking if the object is null. If the object is indeed null, optional
will return null instead of causing an error.
optional
Basic Usage of The most common use case for optional
is accessing a property or method on an Eloquent relationship that might return null.
Example Without optional
:
return view('profile', [
'phone' => $user->phone ? $user->phone->number : null,
]);
In this example, if $user->phone
is null, trying to access the number
property would result in an error.
Example With optional
:
return view('profile', [
'phone' => optional($user->phone)->number,
]);
Here, if $user->phone
is null, optional
will return null instead of trying to access the number
property, preventing an error.
Advanced Usage
optional
is also useful when you want to call a method on a potentially null object.
Example:
$profilePhotoUrl = optional($user->profilePhoto())->getUrl();
If $user->profilePhoto()
returns null, optional
will return null instead of calling getUrl()
on it.
optional
with Closure
Using For more complex conditional operations, optional
can take a closure as its second argument. The closure will only be executed if the object is not null.
Example:
$greeting = optional($user, function ($user) {
return 'Welcome, ' . $user->name;
});
If $user
is not null, the closure will execute and return the greeting. Otherwise, optional
will return null.
Chainability
One of the elegant features of optional
is its chainability. You can chain method calls or property accesses without worrying about encountering a null object in the middle of the chain.
Example:
$country = optional($user->address)->country->name;
This line will not throw an error even if $user->address
is null.
optional
When to Use optional
is particularly useful when:
- Dealing with nested relationships where an intermediate relationship might be null.
- Accessing data from an API response that may not always contain certain fields.
- Working with complex data structures where checking each property or method call for null would be cumbersome.
Laravel's optional
helper function is a simple yet powerful tool for handling null objects gracefully. It helps in writing cleaner and more maintainable code, reducing the need for repetitive null checks. By understanding and utilizing optional
, you can avoid common pitfalls associated with null values and enhance the overall quality of your Laravel applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
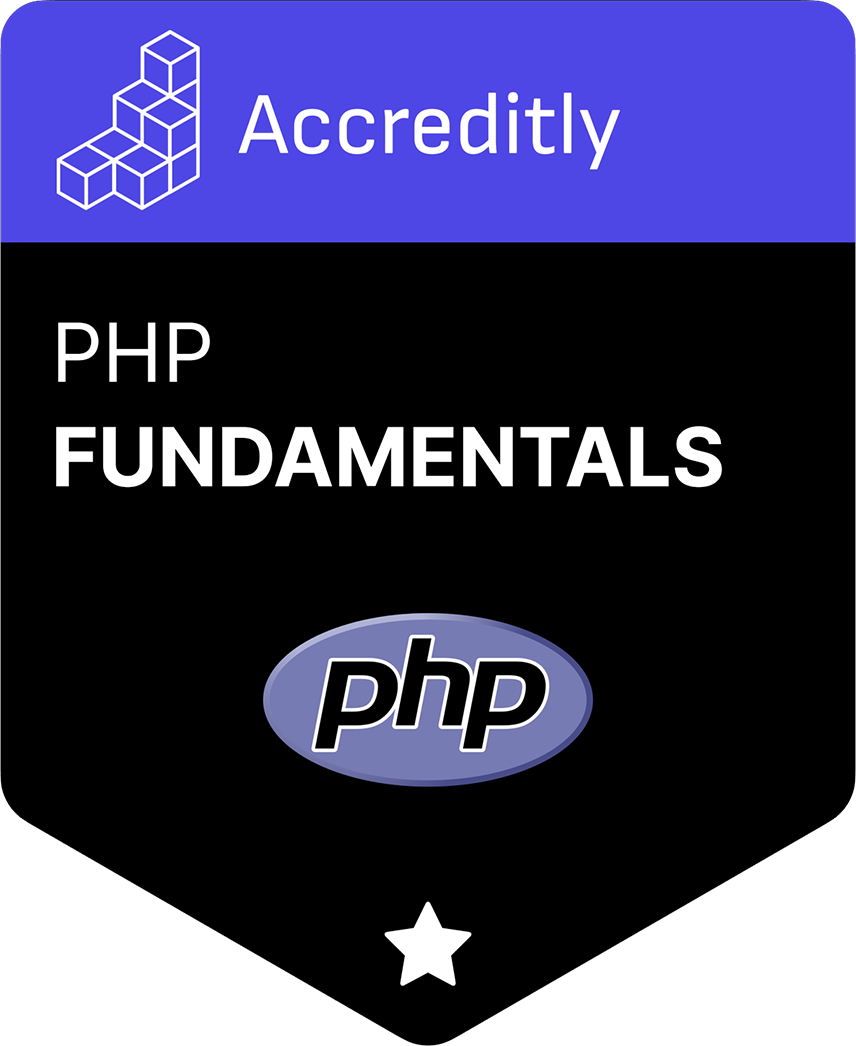