- 1. The if Statement
- 2. The switch Statement
- 3. The match Expression (as of PHP 8.0)
- Comparative Analysis
In PHP, making decisions based on different conditions is a fundamental part of programming. The language offers several control structures for this purpose, notably if
, switch
, and the recently introduced match
. Each has its unique syntax and use case scenarios. Understanding when and how to use these structures is key to writing clear, efficient, and maintainable code.
if
Statement
1. The The if
statement is the most basic way to perform condition-based actions. It's incredibly flexible and ideal for various scenarios, especially when you have a limited number of conditions or more complex logic.
Syntax:
if ($condition) {
// code to execute if condition is true
} elseif ($anotherCondition) {
// code to execute if another condition is true
} else {
// code to execute if no conditions are true
}
When to Use:
- When you have a small number of conditions.
- When conditions are not just based on different values of a single variable.
- When the logic for each condition is more complex.
switch
Statement
2. The switch
is best suited for comparing the same variable or expression with many different values. It's more readable than multiple if
statements when dealing with a single variable.
Syntax:
switch ($variable) {
case 'value1':
// code to execute for value1
break;
case 'value2':
// code to execute for value2
break;
default:
// code to execute if no case is matched
}
When to Use:
- When you have several conditions that use the same variable.
- When you need to compare a variable to distinct constant values.
- When the actions for each case are relatively straightforward.
match
Expression (as of PHP 8.0)
3. The The match
expression, introduced in PHP 8.0, is similar to switch
, but it offers more power and flexibility. It can return values and doesn't require a break
statement to prevent fall-through.
Syntax:
$result = match ($variable) {
'value1' => 'Result for value1',
'value2' => 'Result for value2',
default => 'Default result',
};
When to Use:
- When you need a concise way to return different values based on a single variable.
- When you want to avoid the verbosity of
switch
and the need forbreak
statements. - When you are using PHP 8.0 or higher.
Comparative Analysis
-
Flexibility:
if
is the most flexible, as it can handle various conditions.switch
andmatch
are more limited to specific values of a single variable. -
Readability: For multiple conditions based on the same variable,
switch
andmatch
are more readable than multipleif-else
blocks. -
Performance: While performance differences are usually negligible,
switch
andmatch
can be slightly faster in scenarios with many conditions. -
Return Values: Only
match
can directly return a value, making it suitable for assigning values based on a condition.
Choosing between if
, switch
, and match
depends on the specific requirements of your code. if
is ideal for complex conditions and a smaller number of checks. switch
is great for readability when comparing one variable against many values. match
brings the advantages of switch
with additional features like value returning and concise syntax, provided you're using PHP 8.0 or higher.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
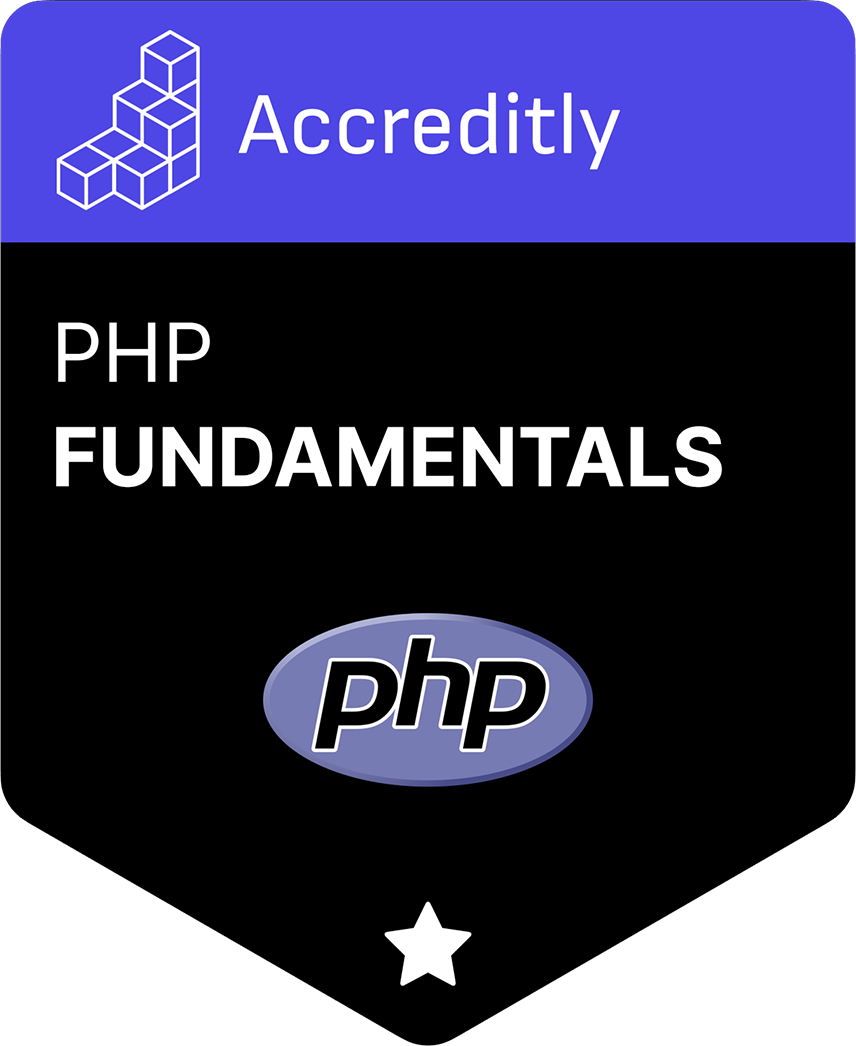