- Prerequisites
- Step 1: Set Up Google Analytics API
- Step 2: Install the Google API Client Library for PHP
- Step 3: Authenticate and Retrieve Data
- Step 4: Display Data on a Custom Dashboard
Google Analytics is a powerful tool for tracking and analyzing website traffic. Integrating Google Analytics with your PHP application allows you to pull data from Analytics and display it on a custom dashboard. This article will guide you through the process of setting up the Google Analytics API, retrieving data, and displaying it on a PHP dashboard.
Prerequisites
Before we begin, ensure you have the following:
- A Google Analytics account and property set up. If you need help setting up Google Analytics, refer to the Google Analytics setup guide.
- A PHP development environment. If you need to set up PHP, refer to the PHP installation guide.
Step 1: Set Up Google Analytics API
First, you need to set up the Google Analytics API and obtain the necessary credentials.
Enable the Google Analytics API
- Go to the Google Cloud Console and create a new project.
- Enable the Google Analytics API for your project by navigating to API & Services > Library and searching for "Google Analytics API."
- Enable the API by clicking the "Enable" button.
Create Service Account Credentials
- Navigate to API & Services > Credentials.
- Create credentials by selecting "Create Credentials" and choosing "Service account."
- Configure the service account and provide it with necessary roles, such as "Viewer."
- Create a key for the service account. Choose JSON as the key type and download the JSON file.
Grant Access to Your Google Analytics Account
- Open your Google Analytics account.
- Navigate to Admin > Account/User Management.
- Add the service account email (found in the JSON key file) as a user with the necessary permissions.
For more detailed instructions, refer to the Google Analytics API documentation.
Step 2: Install the Google API Client Library for PHP
Next, you need to install the Google API Client Library for PHP. This library provides the necessary functions to interact with the Google Analytics API.
Using Composer
If you don't have Composer installed, follow the Composer installation guide.
Install the Google API Client Library:
composer require google/apiclient
Step 3: Authenticate and Retrieve Data
Create a PHP script to authenticate with the Google Analytics API and retrieve data.
Authenticate with the API
require_once 'vendor/autoload.php';
use Google\Client;
use Google\Service\AnalyticsReporting;
// Initialize the Google Client
$client = new Client();
$client->setAuthConfig('path/to/your-service-account-file.json');
$client->addScope(AnalyticsReporting::ANALYTICS_READONLY);
// Initialize the Analytics Reporting service
$analytics = new AnalyticsReporting($client);
Replace 'path/to/your-service-account-file.json'
with the path to your JSON key file.
Retrieve Data from Google Analytics
Define a function to retrieve data from the Google Analytics API:
function getReport($analytics) {
// Create the DateRange object
$dateRange = new Google_Service_AnalyticsReporting_DateRange();
$dateRange->setStartDate("30daysAgo");
$dateRange->setEndDate("today");
// Create the Metrics object
$sessions = new Google_Service_AnalyticsReporting_Metric();
$sessions->setExpression("ga:sessions");
$sessions->setAlias("sessions");
// Create the ReportRequest object
$request = new Google_Service_AnalyticsReporting_ReportRequest();
$request->setViewId("YOUR_VIEW_ID");
$request->setDateRanges($dateRange);
$request->setMetrics(array($sessions));
$body = new Google_Service_AnalyticsReporting_GetReportsRequest();
$body->setReportRequests(array($request));
return $analytics->reports->batchGet($body);
}
$reports = getReport($analytics);
Replace "YOUR_VIEW_ID"
with your Google Analytics view ID. You can find the view ID in your Google Analytics account under Admin > View Settings.
For more information, refer to the Google Analytics Reporting API documentation.
Step 4: Display Data on a Custom Dashboard
Process the retrieved data and display it on a custom PHP dashboard.
Process the Data
Extract the data from the response:
function printResults($reports) {
for ($reportIndex = 0; $reportIndex < count($reports); $reportIndex++) {
$report = $reports[$reportIndex];
$rows = $report->getData()->getRows();
for ($rowIndex = 0; $rowIndex < count($rows); $rowIndex++) {
$row = $rows[$rowIndex];
$metrics = $row->getMetrics();
for ($metricIndex = 0; $metricIndex < count($metrics); $metricIndex++) {
$metricValues = $metrics[$metricIndex]->getValues();
foreach ($metricValues as $value) {
echo "Sessions: $value <br>";
}
}
}
}
}
printResults($reports);
Create the Dashboard
Create an HTML page to display the data:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Google Analytics Dashboard</title>
</head>
<body>
<h1>Google Analytics Dashboard</h1>
<div id="analytics-data">
<?php printResults($reports); ?>
</div>
</body>
</html>
Integrating Google Analytics with PHP allows you to create custom dashboards and gain insights from your website's data. By following the steps outlined in this article—setting up the Google Analytics API, authenticating, retrieving data, and displaying it—you can build a personalized analytics dashboard tailored to your needs.
For more detailed information, refer to the Google Analytics API documentation and the Google API Client Library for PHP documentation.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
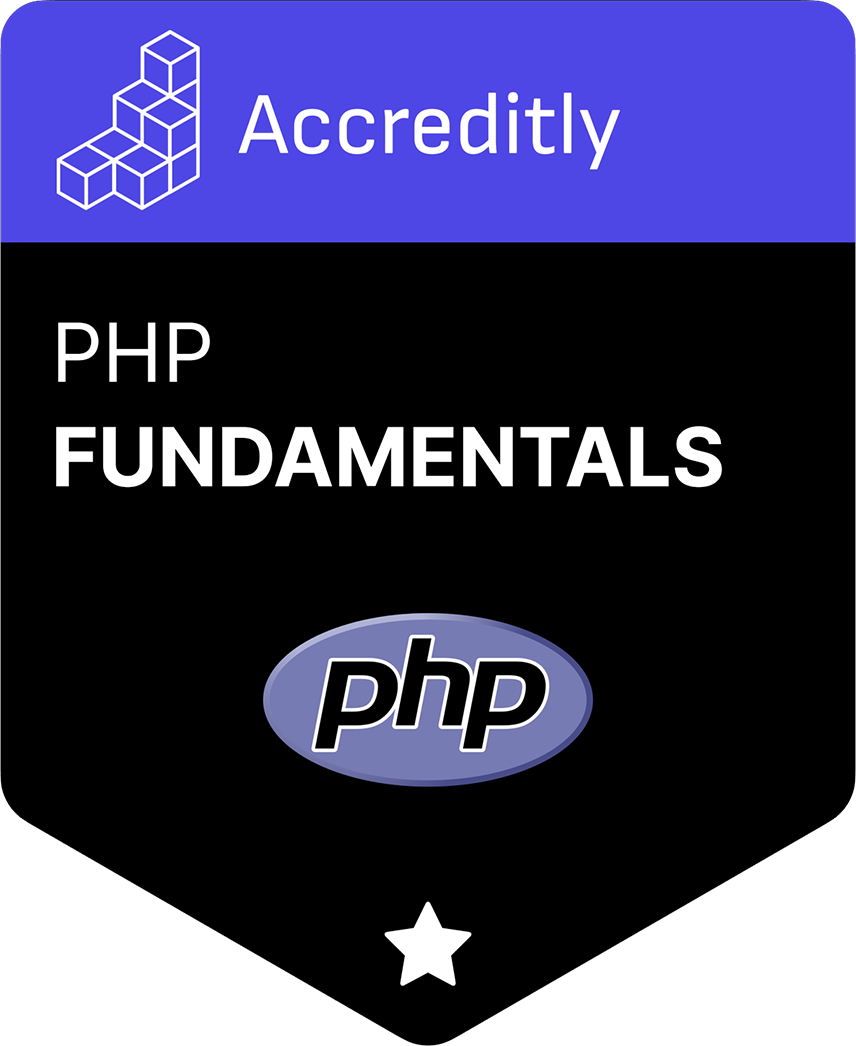