- Step 1: Register Your Application
- Step 2: Implement OAuth for Authentication
- Step 3: Create a Pin
- Step 4: Best Practices and Error Handling
Pinterest is a powerful platform for sharing and discovering ideas through images, known as pins. For developers looking to automate or integrate Pinterest functionalities directly within their PHP applications, understanding how to interact with the Pinterest API is essential. This article provides a detailed walkthrough on how to post to Pinterest using its API with PHP, covering everything from obtaining access credentials to making API calls.
Step 1: Register Your Application
Before you can use the Pinterest API, you must register your application with Pinterest to obtain the necessary credentials.
-
Create a Pinterest Developer Account:
- Visit the Pinterest Developers site and sign in with your Pinterest account.
- Navigate to the Apps section and create a new app.
-
Obtain API Credentials:
- Once your app is set up, you'll receive an API key (Client ID) and an API secret. Keep these credentials secure as they are essential for authenticating API requests.
Step 2: Implement OAuth for Authentication
Pinterest uses OAuth 2.0 for authentication. You will need to implement an OAuth flow to obtain an access token that allows your application to make authorized API calls.
2.1 Request Authorization:
- Direct the user to Pinterest's OAuth endpoint where they can authorize your application. Construct the URL with your client ID and the required permissions:
$client_id = 'YOUR_CLIENT_ID'; $redirect_uri = 'YOUR_REDIRECT_URI'; $scopes = 'read_public,write_public'; $response_type = 'code'; $url = "https://www.pinterest.com/oauth/?" . http_build_query([ 'response_type' => $response_type, 'redirect_uri' => $redirect_uri, 'client_id' => $client_id, 'scope' => $scopes, 'state' => 'optional_csrf_token' ]); echo "<a href='$url'>Login with Pinterest</a>";
2.2 Handle the Callback:
- After the user authorizes your app, they will be redirected to the
redirect_uri
you specified, along with acode
parameter. - Exchange this code for an access token:
if (isset($_GET['code'])) { $code = $_GET['code']; $token_url = "https://api.pinterest.com/v1/oauth/token"; $response = file_get_contents($token_url, false, stream_context_create([ 'http' => [ 'method' => 'POST', 'header' => "Content-Type: application/x-www-form-urlencoded\r\n", 'content' => http_build_query([ 'grant_type' => 'authorization_code', 'client_id' => $client_id, 'client_secret' => $client_secret, 'code' => $code ]) ] ])); $access_token = json_decode($response)->access_token; }
Step 3: Create a Pin
With the access token, you can now post a pin to a specific board on Pinterest.
$data = [
'board' => 'user/board', // Specify the board identifier
'note' => 'This is a test pin from my PHP application', // Note or description of the pin
'image_url' => 'http://example.com/path/to/image.jpg', // Direct URL to the image
'link' => 'http://example.com' // Link for the pin when clicked
];
$api_url = "https://api.pinterest.com/v1/pins/";
$options = [
'http' => [
'header' => "Authorization: Bearer $access_token\r\n" .
"Content-Type: application/json\r\n",
'method' => 'POST',
'content' => json_encode($data)
]
];
$context = stream_context_create($options);
$result = file_get_contents($api_url, false, $context);
$response = json_decode($result);
if ($response && isset($response->data) && isset($response->data->id)) {
echo "Pin created successfully. Pin ID: " . $response->data->id;
} else {
echo "Failed to create pin. Error: " . $response->message;
}
Step 4: Best Practices and Error Handling
- Error Handling: Always check API responses for errors. Handle exceptions and errors gracefully to improve user experience.
- Rate Limits: Be aware of Pinterest's API rate limits to avoid hitting the cap and getting your application blocked.
- Data Privacy: Ensure you respect user data privacy and adhere to all legal requirements, including GDPR where applicable.
Integrating Pinterest API into your PHP application allows you to automate pinning operations and enrich your social media interaction directly from your website. While this guide provides a basic overview, Pinterest's API offers extensive functionalities that you can explore further to maximize your application's social media capabilities. Always ensure to keep up with changes to the API and maintain security best practices to protect your application and users.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
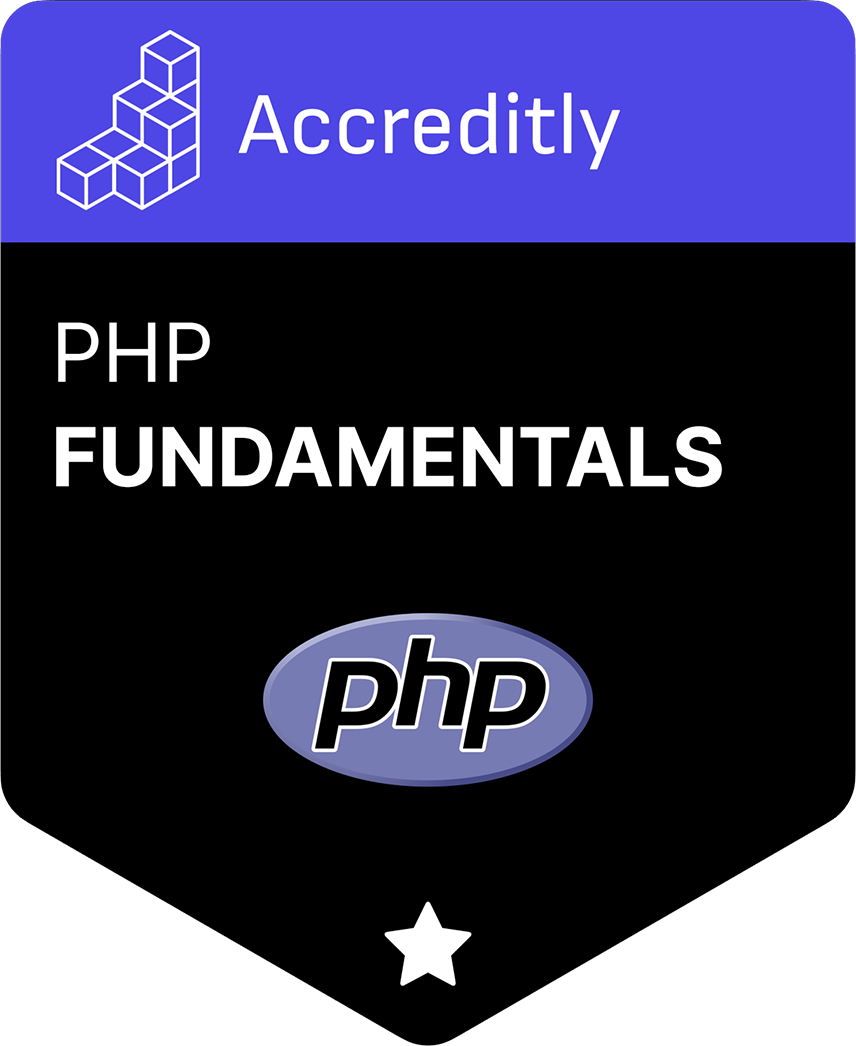