- What is Lazy Loading?
- The Problem with Lazy Loading
- Understanding preventLazyLoading
- Enabling preventLazyLoading
- Enabling preventLazyLoading in Specific Environments
- Handling Lazy Loading Exceptions
- Using Eager Loading to Avoid Lazy Loading
- Using load for Conditional Eager Loading
- Disabling preventLazyLoading for Specific Models
- Benefits of Using preventLazyLoading
As Laravel applications grow in complexity, managing database interactions efficiently becomes crucial. One common pitfall that developers encounter is lazy loading, a feature that can lead to unintended and potentially expensive database queries. To help developers avoid this, Laravel introduced the preventLazyLoading
method, which allows you to catch and prevent lazy loading when it happens in your application. This article will dive into what lazy loading is, why it can be problematic, and how to effectively use the preventLazyLoading
method in Laravel.
What is Lazy Loading?
Lazy loading is a design pattern used in many ORMs (Object-Relational Mappers), including Laravel's Eloquent, where related data is loaded only when it is accessed for the first time. For example, consider the following code:
$user = User::find(1);
$posts = $user->posts;
In this example, when you fetch a User
model, the related posts
are not immediately loaded from the database. Instead, the posts are only retrieved when you explicitly access the $user->posts
property. This is lazy loading in action.
The Problem with Lazy Loading
While lazy loading can be convenient, it can also lead to the "N+1 query problem." This occurs when your code unintentionally triggers additional queries in a loop, which can drastically affect the performance of your application.
For instance:
$users = User::all();
foreach ($users as $user) {
echo $user->posts->count();
}
In this case, if you have 100 users, Laravel will execute 101 queries: one to retrieve all users and 100 more to retrieve the posts for each user. This is highly inefficient, especially as the dataset grows.
preventLazyLoading
Understanding Laravel's preventLazyLoading
method was introduced to help developers avoid the unintended consequences of lazy loading by throwing an exception whenever lazy loading occurs. This feature is particularly useful during development, allowing you to catch potential performance issues early.
preventLazyLoading
Enabling To enable the prevention of lazy loading, you can call the preventLazyLoading
method on the Model
class in your application's AppServiceProvider
or any specific model where you want to enforce this behavior.
Here’s how to enable it globally:
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use Illuminate\Database\Eloquent\Model;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
Model::preventLazyLoading();
}
}
preventLazyLoading
in Specific Environments
Enabling You may not want to prevent lazy loading in all environments (e.g., production). To restrict this behavior to specific environments like development or testing, you can conditionally enable it:
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use Illuminate\Database\Eloquent\Model;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
if ($this->app->isLocal()) {
Model::preventLazyLoading();
}
}
}
Handling Lazy Loading Exceptions
When preventLazyLoading
is enabled, Laravel will throw an Illuminate\Database\LazyLoadingViolationException
whenever it detects lazy loading. This exception provides useful information, such as the model and relationship where lazy loading was attempted.
You can handle this exception by either fixing the code to use eager loading or by explicitly allowing lazy loading where necessary.
Using Eager Loading to Avoid Lazy Loading
Eager loading is the recommended way to avoid the N+1 query problem. Eager loading retrieves related models as part of the initial query, significantly reducing the number of queries executed.
Here’s how you can use eager loading to resolve the earlier example:
$users = User::with('posts')->get();
foreach ($users as $user) {
echo $user->posts->count();
}
By using with('posts')
, you instruct Laravel to load the related posts for all users in a single query, reducing the total number of queries to just two: one for the users and one for the posts.
load
for Conditional Eager Loading
Using Sometimes, you may not know in advance whether a relationship will be accessed. In such cases, you can use the load
method to conditionally eager load relationships:
$user = User::find(1);
// Conditionally load posts if they will be accessed
if ($needsPosts) {
$user->load('posts');
}
$posts = $user->posts;
preventLazyLoading
for Specific Models
Disabling If you want to disable the preventLazyLoading
feature for specific models, you can override it at the model level:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $guarded = [];
protected $preventLazyLoading = false;
}
This approach allows you to selectively allow lazy loading on certain models while keeping it disabled globally.
preventLazyLoading
Benefits of Using - Performance Optimization: By catching unintended lazy loading, you can optimize your queries and improve the overall performance of your application.
- Early Detection: During development, catching lazy loading issues early prevents them from becoming bigger problems in production.
- Encourages Best Practices: Enforcing eager loading by default encourages developers to write more efficient and maintainable code.
Laravel's preventLazyLoading
feature is a powerful tool for improving the performance and reliability of your applications by preventing unintended database queries. By enabling this feature during development, you can catch potential performance bottlenecks early and ensure that your application scales efficiently.
By combining preventLazyLoading
with best practices like eager loading, you can avoid the pitfalls of the N+1 query problem and build applications that are both robust and performant. As with all tools, the key is to use it judiciously, applying it in environments where it will provide the most value without disrupting your development workflow.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
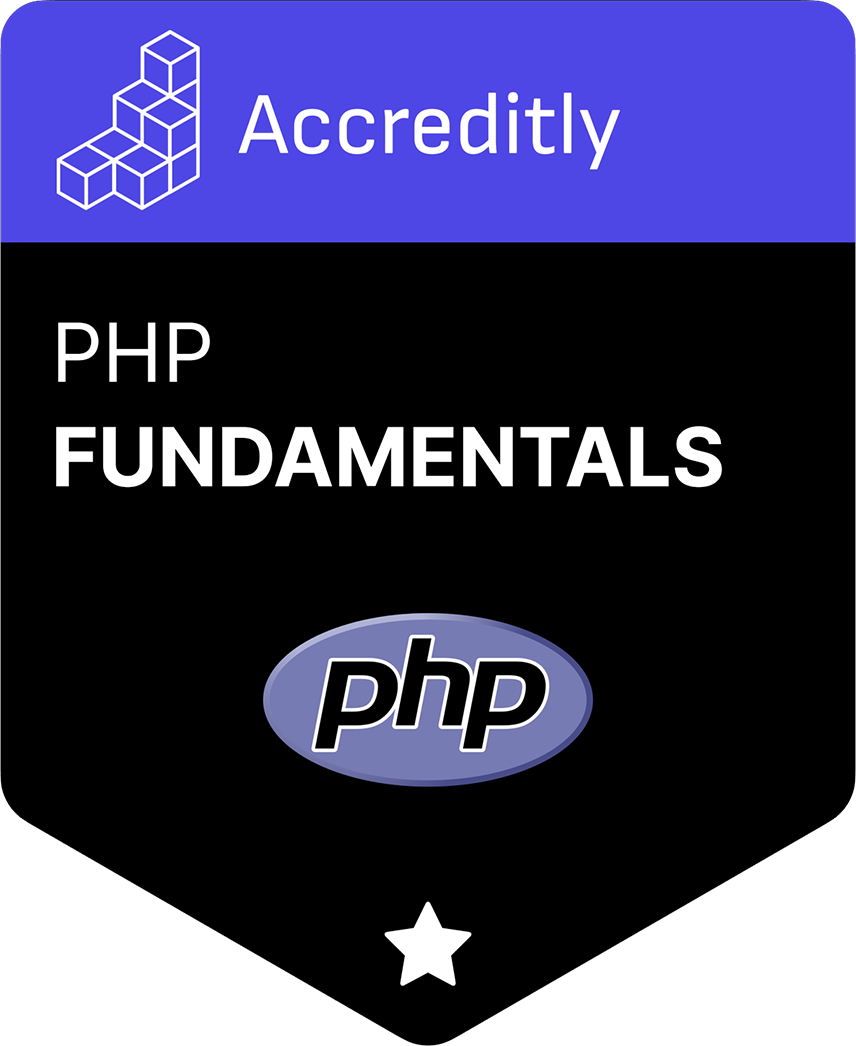