Personalization can significantly enhance the user experience on your WordPress site by delivering content tailored to individual visitors. This article explores various methods to implement personalization in WordPress, including using plugins and custom code. By the end of this guide, you’ll be equipped to create a more engaging and user-centric website.
Why Personalization?
Personalization involves displaying content based on user preferences, behavior, and demographics. It helps in:
- Improving user engagement.
- Increasing conversions.
- Enhancing the overall user experience.
Method 1: Using Plugins
Plugins offer a straightforward way to add personalization to your WordPress site. Below are some recommended plugins for different personalization needs.
1. OptinMonster
OptinMonster is a powerful lead generation plugin that offers advanced targeting rules to personalize content.
Installation and Setup:
-
Install the Plugin: Go to Plugins > Add New in your WordPress dashboard, search for "OptinMonster," and install it.
-
Activate the Plugin: Once installed, activate the plugin.
-
Create a Campaign: Go to OptinMonster > Campaigns and create a new campaign. Use the targeting rules to display personalized content based on user behavior, location, and other factors.
For detailed instructions, refer to the OptinMonster documentation.
2. If-So Dynamic Content
If-So Dynamic Content allows you to display dynamic content based on a variety of conditions.
Installation and Setup:
-
Install the Plugin: Go to Plugins > Add New, search for "If-So Dynamic Content," and install it.
-
Activate the Plugin: Once installed, activate the plugin.
-
Create a Trigger: Go to If-So > Add New Trigger and configure the conditions under which the content should change. For example, you can display different content based on the user's location or referral source.
For more details, refer to the If-So documentation.
Method 2: Using Custom Code
For more control and customization, you can use custom code to personalize content. This involves using hooks and filters provided by WordPress.
Example: Personalizing Content Based on User Role
You can personalize content based on the user role by adding custom code to your theme’s functions.php
file.
Step 1: Check User Role
Use the current_user_can()
function to check the user’s role.
function display_content_based_on_user_role($content) {
if (current_user_can('administrator')) {
$content .= '<p>Welcome, Administrator!</p>';
} elseif (current_user_can('editor')) {
$content .= '<p>Welcome, Editor!</p>';
} else {
$content .= '<p>Welcome, valued user!</p>';
}
return $content;
}
add_filter('the_content', 'display_content_based_on_user_role');
For more information on current_user_can()
, refer to the WordPress Codex.
Example: Personalizing Content Based on User Meta
You can also personalize content based on custom user meta fields.
Step 1: Add User Meta Fields
Add custom fields to user profiles using the show_user_profile
and edit_user_profile
hooks.
function extra_user_profile_fields($user) {
?>
<h3>Extra Profile Information</h3>
<table class="form-table">
<tr>
<th><label for="favorite_color">Favorite Color</label></th>
<td>
<input type="text" name="favorite_color" id="favorite_color" value="<?php echo esc_attr(get_the_author_meta('favorite_color', $user->ID)); ?>" class="regular-text" /><br />
<span class="description">Please enter your favorite color.</span>
</td>
</tr>
</table>
<?php
}
add_action('show_user_profile', 'extra_user_profile_fields');
add_action('edit_user_profile', 'extra_user_profile_fields');
Step 2: Save User Meta Fields
Save the custom fields using the personal_options_update
and edit_user_profile_update
hooks.
function save_extra_user_profile_fields($user_id) {
if (!current_user_can('edit_user', $user_id)) {
return false;
}
update_user_meta($user_id, 'favorite_color', $_POST['favorite_color']);
}
add_action('personal_options_update', 'save_extra_user_profile_fields');
add_action('edit_user_profile_update', 'save_extra_user_profile_fields');
Step 3: Display Personalized Content
Use the get_user_meta()
function to retrieve the custom field value and display personalized content.
function personalized_content_based_on_user_meta($content) {
$user_id = get_current_user_id();
$favorite_color = get_user_meta($user_id, 'favorite_color', true);
if ($favorite_color) {
$content .= '<p>Your favorite color is ' . esc_html($favorite_color) . '.</p>';
}
return $content;
}
add_filter('the_content', 'personalized_content_based_on_user_meta');
For more information on get_user_meta()
, refer to the WordPress Codex.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
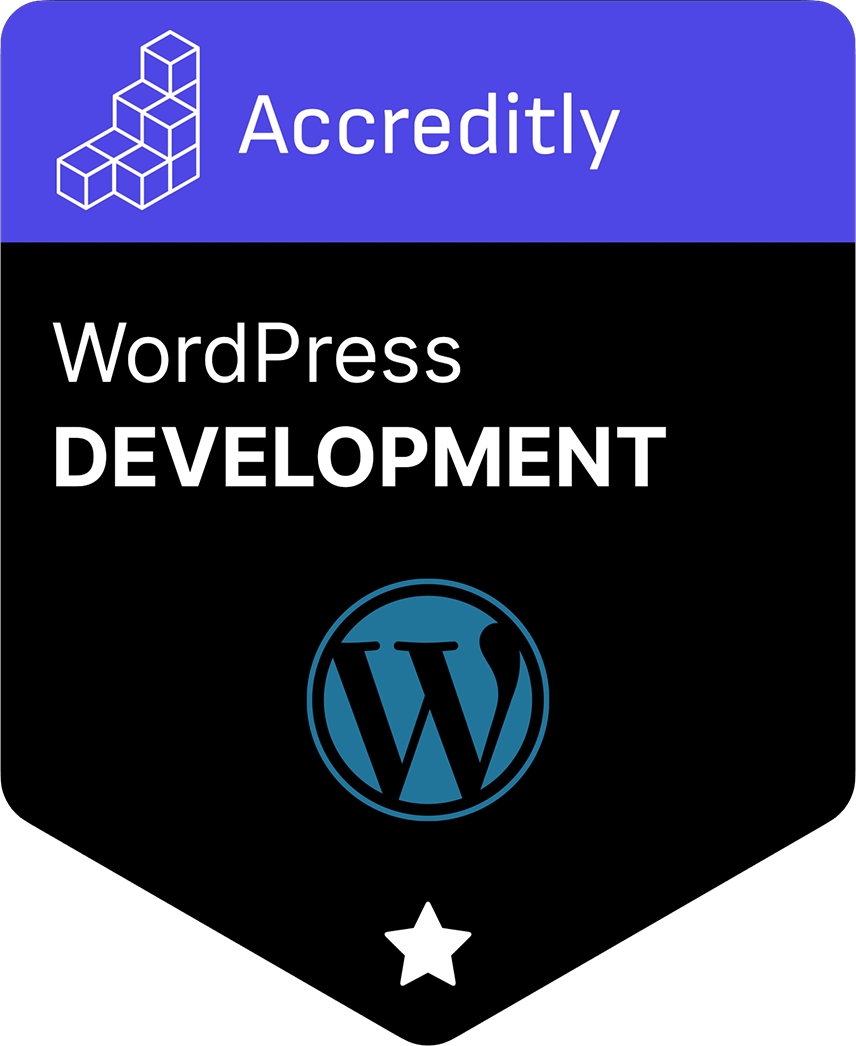