- Step 1: Creating a Custom Post Type for Events
- Step 2: Adding Custom Fields for Event Management
- Step 3: Building the Admin Interface for Event Management
- Step 4: Integrating FullCalendar for Frontend Display
- Step 5: Handling Recurring Events
- Conclusion
Creating a robust event calendar in WordPress involves several key components: an intuitive admin interface for managing events, handling complex scenarios like recurring events, and elegantly displaying these events on the frontend. This guide delves deep into each aspect, ensuring you can build a feature-rich calendar tailored to your WordPress site’s needs.
Step 1: Creating a Custom Post Type for Events
-
Define the Custom Post Type: Add a custom post type for events in your theme’s
functions.php
file:
function create_event_post_type() {
register_post_type('event',
array(
'labels' => array(
'name' => __('Events'),
'singular_name' => __('Event')
),
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'custom-fields'),
)
);
}
add_action('init', 'create_event_post_type');
Step 2: Adding Custom Fields for Event Management
-
Install Advanced Custom Fields (ACF): Use ACF to add custom fields for managing event details. Install and activate the ACF plugin.
-
Create Custom Fields: In your WordPress admin, go to Custom Fields and create a new field group for your events. Add fields for:
- Event Start Date & Time
- Event End Date & Time
- Recurring Event (Checkbox)
- Recurring Frequency (Dropdown with options like daily, weekly, monthly)
- Recurring End Date
Step 3: Building the Admin Interface for Event Management
- Event Creation: Utilize the WordPress editor for adding events. The custom fields created with ACF will be available for inputting event details.
- Managing Recurring Events: When the 'Recurring Event' checkbox is selected, show additional fields to specify the frequency and end date of the recurrence.
Step 4: Integrating FullCalendar for Frontend Display
- Enqueue FullCalendar Scripts and Styles: Add FullCalendar’s resources to your theme:
function enqueue_fullcalendar_scripts() {
wp_enqueue_style('fullcalendar-css', 'https://path-to-fullcalendar/fullcalendar.css');
wp_enqueue_script('fullcalendar-js', 'https://path-to-fullcalendar/fullcalendar.js', array('jquery'), null, true);
}
add_action('wp_enqueue_scripts', 'enqueue_fullcalendar_scripts');
- Displaying the Calendar: Create a shortcode for the calendar:
function render_calendar() {
return '<div id="calendar"></div>';
}
add_shortcode('my_custom_calendar', 'render_calendar');
- Populating the Calendar with Event Data:
AJAX Endpoint: Create a function to fetch event data from your 'event' post type and return it in JSON format suitable for FullCalendar.
function fetch_calendar_events() {
$events_query = new WP_Query(array(
'post_type' => 'event',
'posts_per_page' => -1, // fetch all events
// Additional query parameters if needed
));
$events = array();
if ($events_query->have_posts()) {
while ($events_query->have_posts()) {
$events_query->the_post();
$event_meta = get_post_meta(get_the_ID());
$event_start = $event_meta['event_start_date'][0];
$event_end = $event_meta['event_end_date'][0];
// Handle recurring events
if (!empty($event_meta['recurring_event'][0]) && $event_meta['recurring_event'][0] == 'yes') {
$frequency = $event_meta['recurring_frequency'][0];
$recurring_end = $event_meta['recurring_end_date'][0];
// Logic to calculate recurring event instances
// Add each instance to the $events array
} else {
// Add single event to the $events array
$events[] = array(
'title' => get_the_title(),
'start' => $event_start,
'end' => $event_end
);
}
}
}
wp_send_json($events);
wp_die();
}
add_action('wp_ajax_nopriv_fetch_calendar_events', 'fetch_calendar_events');
add_action('wp_ajax_fetch_calendar_events', 'fetch_calendar_events');
JavaScript to Fetch and Render Events: In your JavaScript file, use AJAX to fetch the events and render them with FullCalendar:
document.addEventListener('DOMContentLoaded', function() {
var calendarEl = document.getElementById('calendar');
var calendar = new FullCalendar.Calendar(calendarEl, {
initialView: 'dayGridMonth',
events: function(fetchInfo, successCallback, failureCallback) {
jQuery.ajax({
url: '/wp-admin/admin-ajax.php',
type: 'POST',
data: {
action: 'fetch_calendar_events'
},
success: function(events) {
successCallback(events);
}
});
}
});
calendar.render();
});
Step 5: Handling Recurring Events
Handling recurring events involves generating multiple event instances based on the specified frequency and adding them to the $events
array in the fetch_calendar_events
function. This logic will depend on the recurrence patterns (daily, weekly, monthly) and the duration of recurrence.
Conclusion
Creating a custom calendar with a comprehensive admin interface and a dynamic frontend display in WordPress is a valuable addition to any website that requires event management. By integrating FullCalendar and leveraging WordPress custom post types and ACF, you can build a fully functional, easily manageable calendar system.
This custom calendar solution not only enriches the functionality of your WordPress site but also provides a seamless, integrated experience for both website administrators managing events and users engaging with the calendar.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
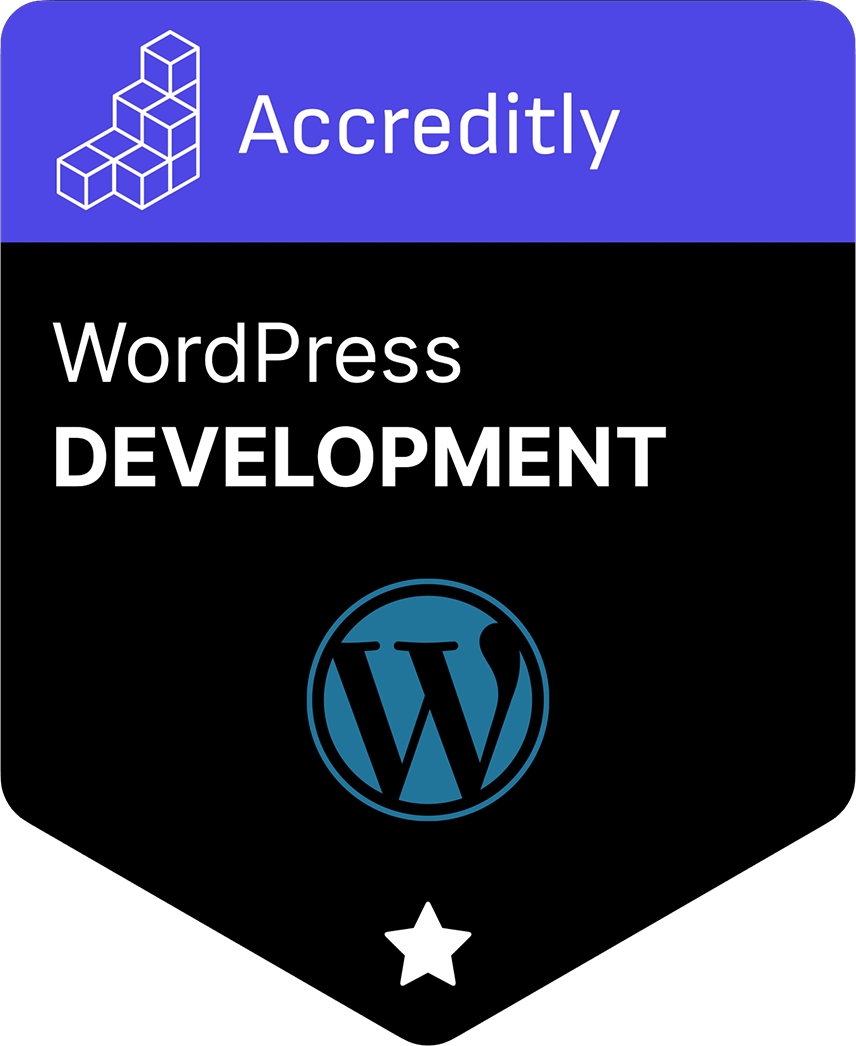