- Understanding Stateless Nature of APIs
- Leveraging Laravel’s Built-in Token Authentication
- Maintaining State Across Requests
- Refreshing and Revoking Tokens
- Best Practices
APIs play a crucial role in facilitating communication between client-side applications and server-side logic. Laravel, a powerful PHP framework, offers robust support for building APIs. One challenge developers often face with API development is maintaining user state in an inherently stateless HTTP protocol. This article delves into strategies for preserving user state across API routes in Laravel, enhancing both security and user experience.
Understanding Stateless Nature of APIs
APIs, particularly RESTful APIs, are designed to be stateless. This means each request from a client to a server must contain all the information the server needs to understand the request, independent of any previous requests. While this design promotes simplicity and scalability, it poses challenges for maintaining user sessions or states across requests.
Leveraging Laravel’s Built-in Token Authentication
Laravel provides several ways to manage user authentication and state in API routes, with token-based authentication being one of the most common and secure methods.
API Tokens with Laravel Sanctum
Laravel Sanctum offers a simple package for API token authentication, allowing you to maintain user states in SPA (Single Page Application), mobile applications, and simple token-based APIs.
Setting Up Sanctum:
- Install Sanctum via Composer:
composer require laravel/sanctum
- Publish the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
- Run the migrations to create the necessary database tables:
php artisan migrate
Using Sanctum for API Tokens:
- To use token authentication, first, ensure your
api
routes are using theauth:sanctum
middleware inapi.php
:
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
- Then, generate tokens for authenticated users. You can do this during login or user registration. Here's an example of token creation upon user login:
public function login(Request $request) {
$request->validate([
'email' => 'required|email',
'password' => 'required',
]);
$user = User::where('email', $request->email)->first();
if (!$user || !Hash::check($request->password, $user->password)) {
return response()->json(['message' => 'Unauthorized'], 401);
}
$token = $user->createToken('apiToken')->plainTextToken;
return response()->json(['token' => $token]);
}
Maintaining State Across Requests
With the token generated, the client must include this token in the Authorization
header of subsequent requests, allowing Laravel to authenticate the user and maintain their state across API routes.
fetch('api/user', {
headers: {
'Authorization': `Bearer ${userToken}`,
},
})
.then(response => response.json())
.then(user => console.log(user));
Refreshing and Revoking Tokens
- Refreshing Tokens: For added security, you might want to implement token expiration and refreshing. Sanctum does not come with built-in token refreshing since each token is stateless. You can manage token expiration manually by adding custom logic to expire tokens and issue new ones as needed.
- Revoking Tokens: You can revoke tokens when they are no longer needed (e.g., user logout) to ensure that the token cannot be used for further requests:
$request->user()->currentAccessToken()->delete();
Best Practices
- Always use HTTPS to encrypt API requests and protect token integrity.
- Advise clients on secure token storage strategies to prevent leakage or theft.
- Embrace the stateless design of APIs. Use tokens to authenticate requests without relying on server-side session storage.
Maintaining user state in Laravel API routes effectively hinges on secure token-based authentication. By leveraging Laravel Sanctum, developers can implement a robust system that authenticates users and carries their state across stateless API requests. This not only enhances the security of Laravel applications but also ensures a consistent and seamless user experience across client-server interactions. Remember, the goal is to authenticate and authorize users efficiently while adhering to the stateless nature of RESTful APIs.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
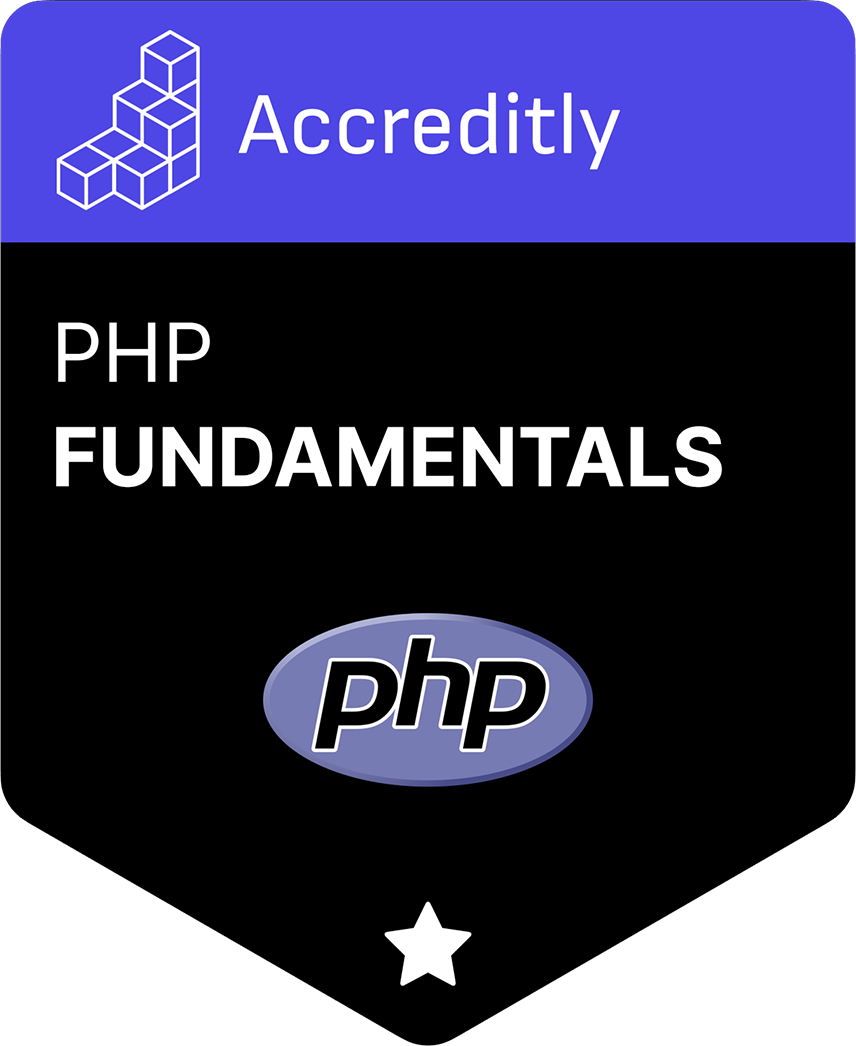