- Introduction
- 1. Keep MySQL Updated
- 2. Use Strong Passwords
- 3. Manage User Privileges
- 4. Use SSL/TLS for Connections
- 5. Secure the MySQL Configuration
- 6. Regular Backups
- 7. Monitor and Audit
- 8. Use Firewall and Network Security
- 9. Protect Against SQL Injection
- 10. Remove Unnecessary Users and Databases
- Remember
MySQL is one of the most widely used relational database management systems in the world, powering a significant portion of web applications. Ensuring its security is crucial to protect sensitive data from unauthorized access and potential breaches. This article explores various best practices to secure MySQL databases effectively.
Introduction
Securing your MySQL database is not just about setting a strong password. It encompasses a wide range of practices from user management to network security. Let’s delve into these practices to ensure your MySQL instances are secure.
1. Keep MySQL Updated
Why Update?
Keeping MySQL up-to-date is the first line of defense against potential security vulnerabilities. Updates often include patches for known security flaws.
How to Update
On a Linux system, you can update MySQL using the package manager. For example, on Ubuntu:
sudo apt-get update
sudo apt-get upgrade mysql-server
For Windows, download the latest version from the MySQL website.
2. Use Strong Passwords
Password Complexity
Ensure all MySQL user accounts have strong, complex passwords. Avoid using simple or common passwords.
Changing Passwords
To change a user's password in MySQL, use the following command:
ALTER USER 'username'@'host' IDENTIFIED BY 'new_password';
3. Manage User Privileges
Principle of Least Privilege
Only grant users the minimum privileges they need to perform their tasks. This minimizes the damage in case of a compromised account.
Grant and Revoke Privileges
Use the GRANT
and REVOKE
statements to manage user privileges:
GRANT SELECT, INSERT ON database_name.* TO 'username'@'host';
REVOKE DELETE ON database_name.* FROM 'username'@'host';
Example: Creating a Read-Only User
CREATE USER 'readonly_user'@'localhost' IDENTIFIED BY 'password';
GRANT SELECT ON your_database.* TO 'readonly_user'@'localhost';
4. Use SSL/TLS for Connections
Encrypt Data in Transit
Ensure that all connections to the MySQL server are encrypted using SSL/TLS. This prevents data from being intercepted during transmission.
Configuring SSL
- Generate SSL certificates.
- Configure the MySQL server to use SSL.
[mysqld]
ssl-ca=/path/to/ca-cert.pem
ssl-cert=/path/to/server-cert.pem
ssl-key=/path/to/server-key.pem
- Restart the MySQL server and require SSL for users:
CREATE USER 'secure_user'@'host' IDENTIFIED BY 'password' REQUIRE SSL;
GRANT ALL PRIVILEGES ON database_name.* TO 'secure_user'@'host';
5. Secure the MySQL Configuration
my.cnf File
Restrict access to the MySQL configuration file (my.cnf
or my.ini
) to prevent unauthorized modifications.
chmod 600 /etc/mysql/my.cnf
Disable Remote Root Login
Prevent remote root access to reduce the risk of unauthorized access:
[mysqld]
skip-networking
bind-address = 127.0.0.1
Example: Binding MySQL to a Specific IP Address
[mysqld]
bind-address = 192.168.1.100
6. Regular Backups
Backup Strategy
Regularly back up your MySQL databases to ensure data can be recovered in case of an incident.
mysqldump
Using mysqldump -u username -p database_name > backup.sql
Automating Backups with Cron Jobs
crontab -e
Add the following line to schedule a daily backup at 2 AM:
0 2 * * * mysqldump -u username -p'password' database_name > /path/to/backup/backup.sql
7. Monitor and Audit
Enable Logging
Enable MySQL logging to keep track of queries and activities. This helps in detecting suspicious behavior.
[mysqld]
general_log = 1
general_log_file = /var/log/mysql/general.log
Use MySQL Enterprise Audit
Consider using MySQL Enterprise Audit for more advanced auditing features.
8. Use Firewall and Network Security
Restrict Access
Use firewall rules to restrict access to the MySQL server.
sudo ufw allow from 192.168.1.0/24 to any port 3306
Example: IPTables Rule
iptables -A INPUT -p tcp --dport 3306 -s 192.168.1.0/24 -j ACCEPT
9. Protect Against SQL Injection
Use Prepared Statements
Always use prepared statements to protect against SQL injection attacks.
<?php
$mysqli = new mysqli("localhost", "user", "password", "database");
$stmt = $mysqli->prepare("SELECT * FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
$result = $stmt->get_result();
?>
Example: PHP Prepared Statement
<?php
$conn = new PDO("mysql:host=localhost;dbname=database", "username", "password");
$stmt = $conn->prepare("SELECT * FROM users WHERE email = :email");
$stmt->bindParam(':email', $email);
$stmt->execute();
?>
10. Remove Unnecessary Users and Databases
List Users
Regularly review and remove any unnecessary user accounts.
SELECT User, Host FROM mysql.user;
Drop Unused Databases
DROP DATABASE IF EXISTS old_database;
Remember
Securing your MySQL database requires a multi-faceted approach that includes updating software, managing user privileges, encrypting connections, and regular monitoring. By implementing these best practices, you can significantly enhance the security of your MySQL databases and protect your sensitive data from potential threats.
For further reading and advanced security configurations, refer to the official MySQL documentation.
Interested in proving your knowledge of this topic? Take the Database Fundamentals certification.
Database Fundamentals
Focusing on SQL and database design, this exam will test your knowledge of database fundamentals.
$99
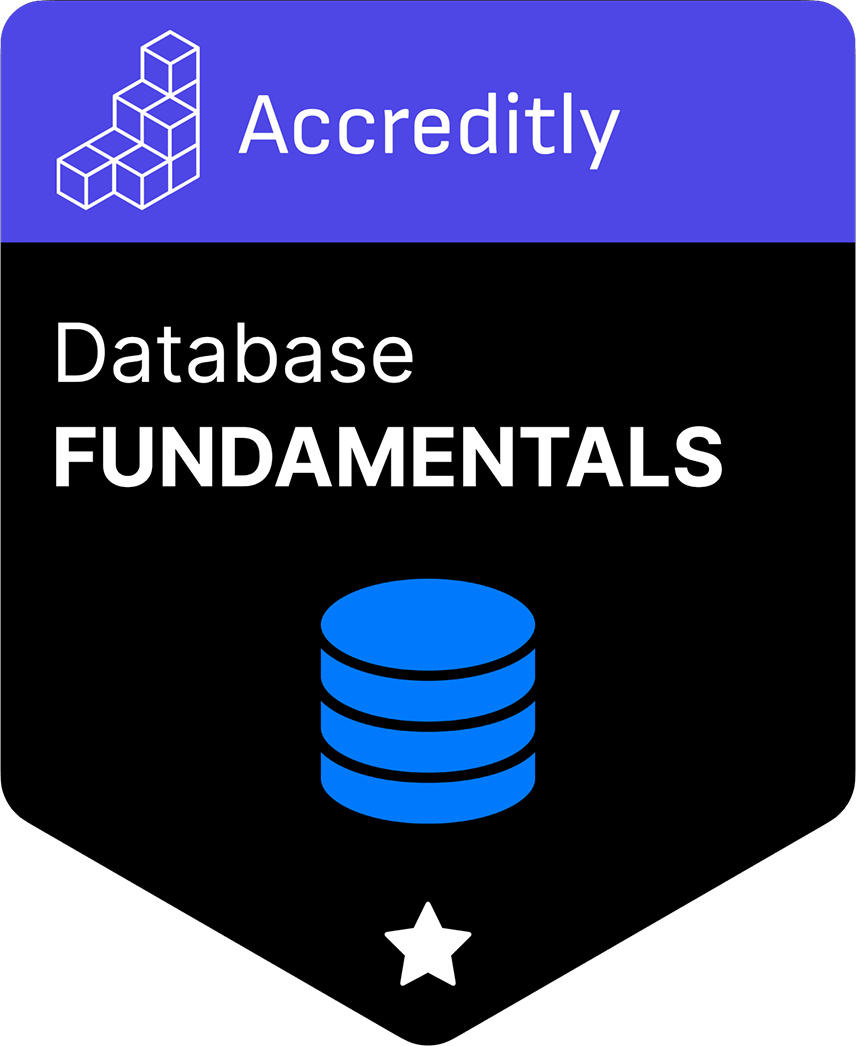