- What is Loose Typing?
- Loose Typing in Action
- Understanding Type Coercion
- Common Pitfalls and Solutions
- Loose Typing in Arrays
- Handling Loose Typing in Functions
- Loose Typing and Object Properties
- Best Practices and Guidelines
- Summing up
Loose typing in JavaScript is both a feature and a challenge, offering flexibility and ease of development while presenting potential pitfalls. It allows variables to hold values of any type and to change types over time. This article provides an in-depth look at loose typing, enriched with examples to illustrate its nuances.
What is Loose Typing?
JavaScript is a dynamically typed language, meaning variables can hold values of any type and their types can change. This is different from statically typed languages where the type of a variable is known at compile time and typically doesn't change.
Loose Typing in Action
Example 1: Changing Variable Types
let myVar = 42; // Initially, myVar is a number
console.log(typeof myVar); // "number"
myVar = "Hello World"; // Now, myVar is a string
console.log(typeof myVar); // "string"
myVar = true; // Now, myVar is a boolean
console.log(typeof myVar); // "boolean"
Example 2: Implicit Type Conversion
When operating on values of different types, JavaScript often converts types implicitly.
let result = "3" + 5; // "3" is a string, 5 is a number
console.log(result); // Outputs "35" - number 5 is converted to a string
Understanding Type Coercion
Type coercion is a fundamental concept in loosely typed languages where the interpreter automatically converts types at runtime.
Numeric String and Number Operations
let sum = "100" - 50; // "100" is a string, 50 is a number
console.log(sum); // Outputs 50 - "100" is coerced to a number
Boolean Operations and Coercion
let flag = "true";
if (flag) {
console.log("Flag is true"); // Outputs "Flag is true" - non-empty string is truthy
}
Common Pitfalls and Solutions
Pitfall: Loose Equality vs. Strict Equality
Using ==
(loose equality) can lead to unexpected results due to type coercion.
Example:
console.log(0 == "0"); // true - number is converted to string
console.log(1 == "1"); // true
console.log(0 == ""); // true - empty string is converted to 0
console.log(0 == false); // true - false is converted to 0
Solution: Use Strict Equality
console.log(0 === "0"); // false - strict equality checks value and type
console.log(1 === "1"); // false
Pitfall: Implicit Global Variable Declaration
In non-strict mode, assigning a value to an undeclared variable creates it as a global variable.
Example:
function setNumber() {
num = 42; // num is not declared
}
setNumber();
console.log(num); // 42 - num is now a global variable
Solution: Declare Variables Properly
function setNumber() {
let num = 42; // Proper declaration
}
setNumber();
console.log(typeof num); // undefined
Loose Typing in Arrays
Arrays in JavaScript can hold elements of different types due to loose typing.
Example:
let myArray = [1, "hello", true, { name: "John" }];
console.log(myArray);
Handling Loose Typing in Functions
Functions in JavaScript can accept arguments of any type and return any type.
Example:
function mixTypes(a, b) {
return a + b;
}
console.log(mixTypes(10, "20")); // "1020"
console.log(mixTypes("Hello", { name: "World" })); // "Hello[object Object]"
Loose Typing and Object Properties
Objects can have properties of any type, and their types can change.
Example:
let myObject = {
prop1: 10,
prop2: "Hello"
};
myObject.prop1 = "Changed to a string";
console.log(myObject);
Best Practices and Guidelines
- Use Strict Mode: It helps in catching accidental global variable declarations and other mistakes.
- Avoid Implicit Type Coercion: Be aware of how JavaScript coerces types and avoid scenarios where this could lead to bugs.
- Use Tools Like Linters: Tools like ESLint can help catch potential type-related errors.
- Test Thoroughly: Especially in cases where functions or operations involve multiple data types.
Summing up
Understanding loose typing in JavaScript is crucial for writing effective and reliable code. While it provides flexibility, being mindful of its intricacies helps prevent common pitfalls associated with dynamic typing. As you grow as a JavaScript developer, learning to navigate and utilize loose typing will enhance your coding skill set and prepare you for complex coding scenarios.
Loose typing in JavaScript is like playing with a shape-shifting puzzle. It offers flexibility and adaptability but requires keen awareness and understanding to fit the pieces together correctly. By understand loose typing thoroughly, you equip yourself with the knowledge to build more dynamic, robust, and versatile apps.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
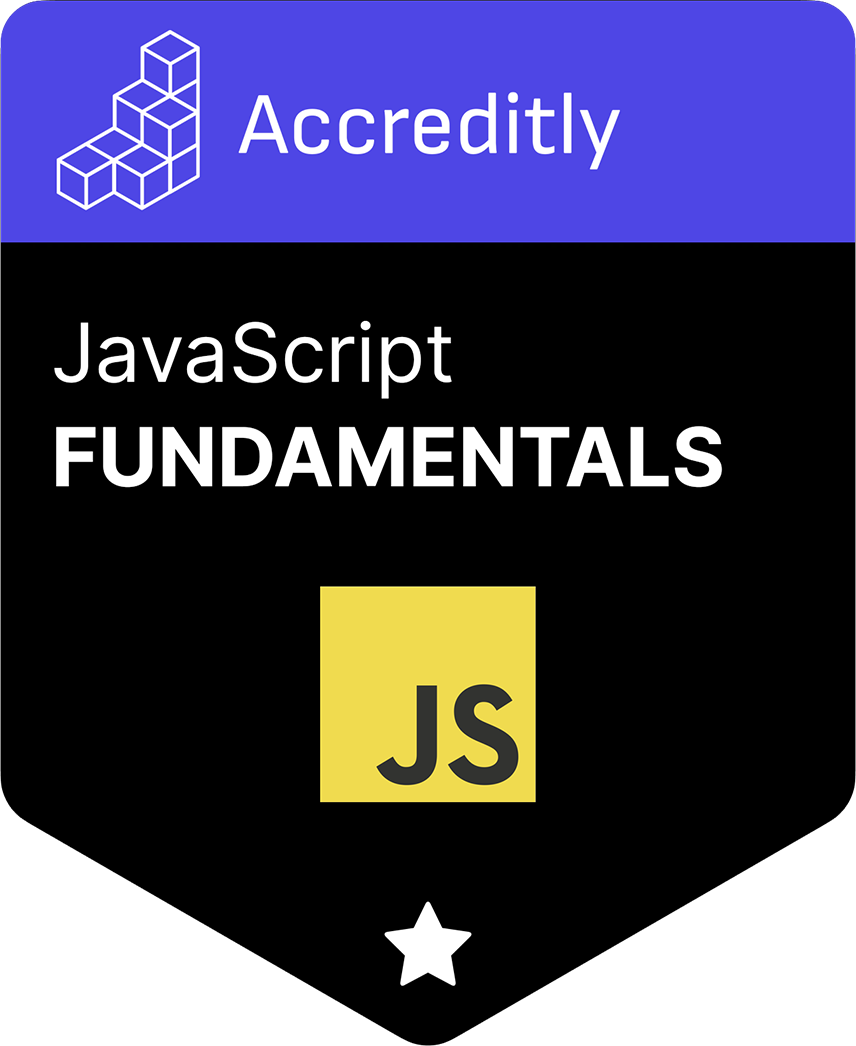