- What is requestAnimationFrame?
- Why Use requestAnimationFrame?
- How Does requestAnimationFrame Work?
- Best Practices
In the realm of web development, creating smooth animations and achieving optimal rendering performance are critical for enhancing user experience. Traditionally, animations were managed using JavaScript setInterval
or setTimeout
functions, which, while functional, often led to less-than-ideal performance. The introduction of requestAnimationFrame
(rAF) marked a significant advancement, providing developers with a more efficient mechanism for creating animations. This article explores requestAnimationFrame
, outlining its advantages, how it works, and best practices for its use.
requestAnimationFrame
?
What is requestAnimationFrame
is a JavaScript API that allows developers to perform animations in a way that's optimized for browser performance. It works by telling the browser you wish to perform an animation and requesting the browser to call a specified function to update the animation before the next repaint. The method takes a callback as an argument, which is executed before the browser's next repaint. Unlike setInterval
or setTimeout
, which execute the callback at a fixed time interval, requestAnimationFrame
adjusts to the browser's refresh rate, leading to smoother animations and less CPU load.
requestAnimationFrame
?
Why Use Performance Optimization: rAF is synced with the browser's refresh rate, typically 60 frames per second (fps), though this can vary with high refresh rate displays. This synchronization ensures animations run as smoothly as possible without causing layout thrashing or unnecessary reflows.
Resource Friendly: The browser can optimize concurrent animations together into a single reflow and repaint cycle, leading to higher performance and lower resource consumption.
Visibility Awareness: rAF callbacks are paused in hidden or non-active tabs, reducing CPU, GPU, and battery usage, a significant advantage for mobile devices and laptops.
requestAnimationFrame
Work?
How Does To utilize requestAnimationFrame
in your web application, you essentially loop through the animation frames by recursively calling rAF within its callback function. Here's a simple example:
let lastFrameTime = 0;
function animate(time) {
if (lastFrameTime === 0) {
lastFrameTime = time;
}
const timePassed = time - lastFrameTime;
// Perform animation logic here
console.log(`Time passed since last frame: ${timePassed}ms`);
lastFrameTime = time;
requestAnimationFrame(animate);
}
requestAnimationFrame(animate);
In this example, animate
is the callback function passed to requestAnimationFrame
. It calculates the time passed since the last frame and logs it, providing a foundation upon which animation logic can be built.
Best Practices
1. Throttling for Performance: In scenarios where your animation doesn't need to run at 60fps (e.g., slower moving animations), it's a good practice to throttle your requestAnimationFrame
calls to reduce the workload on the browser.
2. Fallback for Older Browsers: Although rAF is widely supported across modern browsers, always include a fallback mechanism for older browsers. You can use a polyfill or revert to setTimeout
with a fixed delay.
window.requestAnimationFrame = window.requestAnimationFrame || function(callback) {
return setTimeout(callback, 1000 / 60);
};
3. Stopping the Animation: Since requestAnimationFrame
calls itself recursively, stopping the animation when it's no longer needed is crucial. You can achieve this by using cancelAnimationFrame
, passing it the identifier returned by requestAnimationFrame
.
let animationFrameId;
function stopAnimation() {
cancelAnimationFrame(animationFrameId);
}
function animate() {
// Animation logic
animationFrameId = requestAnimationFrame(animate);
}
requestAnimationFrame(animate);
requestAnimationFrame
offers a sophisticated approach to creating animations in web development, ensuring they are as smooth and efficient as possible. By syncing with the browser's refresh rate and minimizing unnecessary processing, rAF enhances the visual performance of web applications, contributing to a superior user experience. As you delve into creating animations, integrating requestAnimationFrame
into your toolkit will undoubtedly elevate the quality and performance of your animated features.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
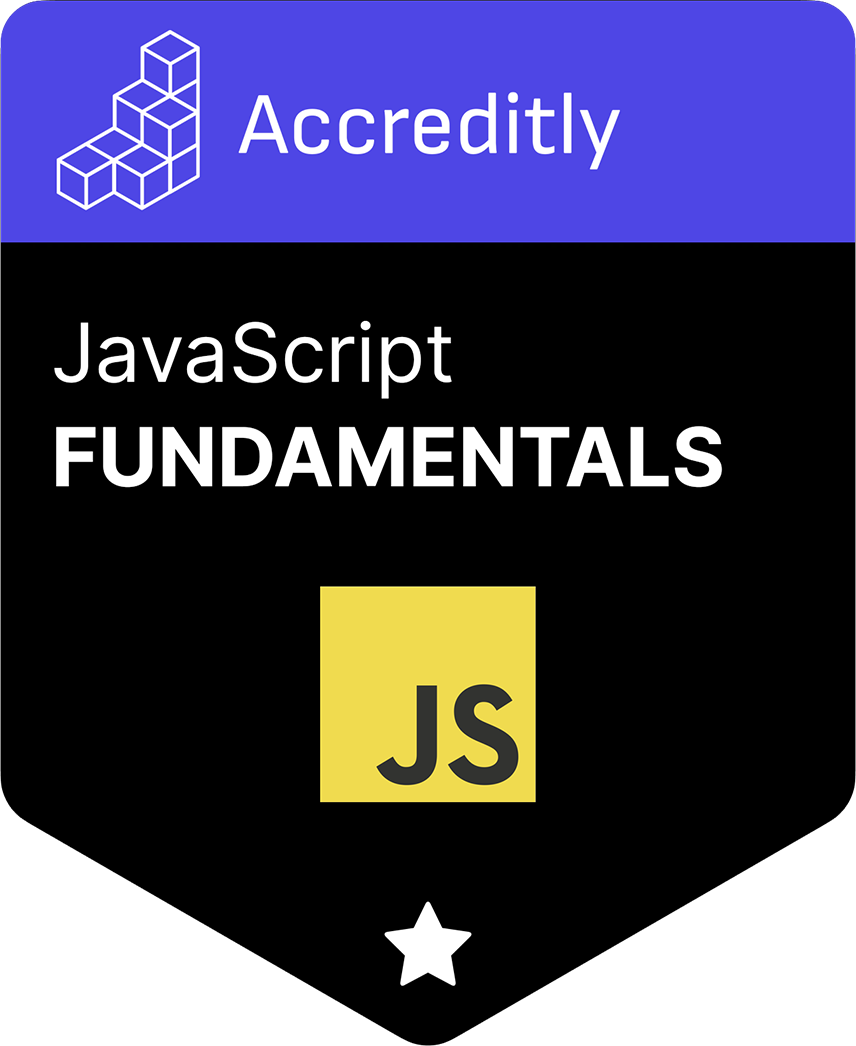