- What is the N+1 Problem?
- Why is the N+1 Problem Bad?
- Identifying the N+1 Problem
- How to Avoid the N+1 Problem in PHP
- When to Use Eager Loading
- Advanced Techniques: Batch Loading and Query Optimization
When working with database-driven applications using frameworks like Laravel or Symfony, the N+1 problem is a common yet often overlooked issue that can severely impact the performance of your application. This article will delve into what the N+1 problem is, why it occurs, how it can affect your PHP applications, and the best practices to avoid it.
What is the N+1 Problem?
The N+1 problem is a performance issue that occurs when an application makes N additional database queries to retrieve related data for N objects that have already been fetched. This problem typically arises when an Object-Relational Mapper (ORM) like Laravel's Eloquent or Doctrine in Symfony is used to manage database interactions, and it often happens due to lazy loading of related data.
A Simple Example in PHP
To understand the N+1 problem, let's consider a common scenario in a PHP application where you have two related database tables: authors
and books
. Each author can have multiple books, and you want to display a list of all authors along with the titles of the books they’ve written.
Here’s how the code might look using Laravel’s Eloquent ORM:
$authors = Author::all();
foreach ($authors as $author) {
$books = $author->books;
foreach ($books as $book) {
echo $book->title;
}
}
At first glance, this code seems perfectly fine. However, if there are 10 authors in the database, this code will result in 11 database queries:
- The first query retrieves all authors:
SELECT * FROM authors;
- Then, for each of the 10 authors, a separate query is made to fetch the books associated with that author:
SELECT * FROM books WHERE author_id = ?;
In this example, 1 query is used to fetch the authors, and N additional queries (where N is the number of authors) are used to fetch the books for each author, hence the term N+1 problem.
Why is the N+1 Problem Bad?
The N+1 problem can significantly degrade the performance of your application. Each additional database query adds overhead, especially if the database is on a separate server or if the data set is large. As the number of records (N) increases, the performance hit becomes more pronounced. For instance, if there are 1000 authors, your application will execute 1001 queries instead of just two or three, leading to unnecessary load on the database and slower response times.
Identifying the N+1 Problem
Identifying the N+1 problem in your PHP application usually involves monitoring the number of queries your application executes during a request. Many PHP frameworks provide tools for this purpose.
In Laravel, for instance, you can use the DB::listen
method to log all SQL queries executed during a request:
DB::listen(function ($query) {
var_dump($query->sql);
});
This allows you to see if your application is making more queries than necessary and can help pinpoint where the N+1 problem occurs.
How to Avoid the N+1 Problem in PHP
Fortunately, avoiding the N+1 problem is straightforward once you know what to look for. The key is to use eager loading instead of lazy loading to fetch related data. Eager loading retrieves all necessary data with as few queries as possible, usually by using JOINs or separate preloaded queries.
Eager Loading with Laravel’s Eloquent
Using the previous example, you can eliminate the N+1 problem in Laravel by modifying the query to use eager loading:
$authors = Author::with('books')->get();
foreach ($authors as $author) {
foreach ($author->books as $book) {
echo $book->title;
}
}
This code generates only two queries:
- One to fetch all authors:
SELECT * FROM authors;
- One to fetch all books related to these authors:
SELECT * FROM books WHERE author_id IN (?, ?, ...);
By using with('books')
, Laravel automatically handles the relationships, loading all related books in a single query and significantly reducing the number of queries executed.
Eager Loading with Doctrine in Symfony
Similarly, in Symfony using Doctrine, you can avoid the N+1 problem by using the fetch
or join
options to eagerly load related entities. Here’s how it might look:
$authors = $entityManager->createQuery('
SELECT a, b
FROM App\Entity\Author a
JOIN a.books b
')->getResult();
This query fetches all authors and their associated books in one go, ensuring that only one query is executed, no matter how many authors or books are in the database.
When to Use Eager Loading
While eager loading is a great way to avoid the N+1 problem, it’s important to use it judiciously. Eager loading all related data all the time can lead to over-fetching, where your application loads more data than it needs, which can also impact performance. The key is to balance between eager loading and lazy loading based on the specific needs of your queries.
For instance, if you’re certain that you’ll need related data for every object in the result set, eager loading is the way to go. However, if related data is only occasionally required, lazy loading might be more appropriate, but you should be mindful of the potential N+1 problem.
Advanced Techniques: Batch Loading and Query Optimization
In addition to eager loading, there are other techniques you can use to optimize your queries and avoid the N+1 problem:
-
Batch Loading: Batch loading involves grouping related queries into fewer, larger batches instead of executing one query per object. This can be useful when dealing with complex data structures where multiple levels of relationships exist.
-
Query Optimization: Optimizing your queries by selecting only the necessary columns or filtering data at the database level can also help reduce the number of queries and the amount of data transferred between your application and the database.
The N+1 problem is a common performance issue that can sneak into your PHP applications if you’re not careful. Understanding how and why it occurs is the first step in avoiding it. By using techniques like eager loading, monitoring your queries, and optimizing your database interactions, you can ensure that your PHP applications remain fast and efficient, even as they scale.
Whether you’re using Laravel, Symfony, or any other PHP framework, being mindful of the N+1 problem will help you write better, more performant code that can handle large datasets and complex relationships without unnecessary database overhead.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
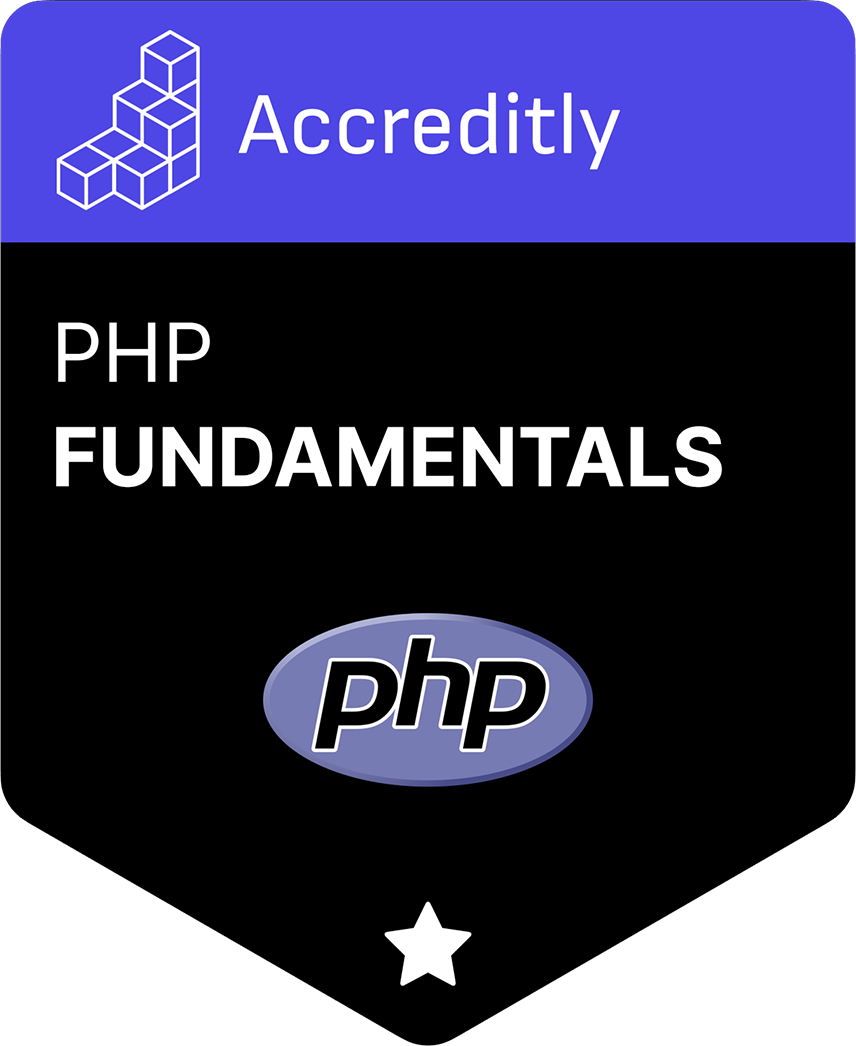