There are a number of ways of getting the current WordPress version that you are running on your website.
Quick methods, not in code
The quick methods which are available to you not in code are pretty obvious.
The first one is by logging into the admin panel and looking in the bottom right of the WordPress admin panel, which will state the version (eg: Version 6.1.1).
The second option is on the "At a Glance" box that appears on the dashboard when logging in.
The third one is in the Updates screen, by clicking Dashboard > Updates. This screen will state the current version.
Looking within code
If you have the codebase open in your code editor and want to find the current version then this is pretty straightforward to do.
There are a number of reasons why you might want to do this. An example is if you've been sent a WordPress website to migrate or deploy, but need to check the WordPress version first (perhaps to configure hosting appropriately). Using this method you'll be able to check the version before going to the effort of deploying.
Using your favourite IDE or code editor, open the project and navigate to the following file:
wp-includes/version.php
This file will list a number of available versions, including:
-
$wp_version
- this is the WordPress version. -
$wp_db_version
- the WordPress revision, which increments when a change to the WP database happens. This often prompts the database update screen when logging in to the admin. -
$tinymce_version
- The version of TinyMCE being run. -
$required_php_version
- Very useful for hosting requirements, this is the minimum required PHP version. Note that some versions of WordPress will produce warnings on very high or bleeding edge PHP versions. -
$required_mysql_version
- Also useful for hosting requirements. This hasn't changed from5.0
for a long time.
An example full file is available below:
<?php
/**
* WordPress Version
*
* Contains version information for the current WordPress release.
*
* @package WordPress
* @since 1.2.0
*/
/**
* The WordPress version string.
*
* Holds the current version number for WordPress core. Used to bust caches
* and to enable development mode for scripts when running from the /src directory.
*
* @global string $wp_version
*/
$wp_version = '6.1.1';
/**
* Holds the WordPress DB revision, increments when changes are made to the WordPress DB schema.
*
* @global int $wp_db_version
*/
$wp_db_version = 53496;
/**
* Holds the TinyMCE version.
*
* @global string $tinymce_version
*/
$tinymce_version = '49110-20201110';
/**
* Holds the required PHP version.
*
* @global string $required_php_version
*/
$required_php_version = '5.6.20';
/**
* Holds the required MySQL version.
*
* @global string $required_mysql_version
*/
$required_mysql_version = '5.0';
Getting the PHP version inside code/PHP
If you need to access the current WordPress version inside PHP for any reason, perhaps to check for support for certain features then you're able to access it using any of the variables available in the version.php
file available above.
For example, to simply output the version in a file in your theme, simply do the following:
<?php
echo $wp_version;
?>
This will output something like: 6.1.1
.
Comparing version strings
Simply having a version string such as 6.1.1
isn't very useful if you need to do a comparison. You can't simply convert it to an integer, or remove the periods (.
) to easily compare.
However, PHP has a built-in function available for comparing versions just like this.
Take the following code, which checks if the current version is higher than the desired version. In this example we check if the current version of WordPress is the same or greater than version 5.0, which is when Gutenberg was introduced.
if( version_compare($wp_version, '5.0', '>=') ) {
// At least version 5.0 of WordPress, so we can assume Gutenberg is around
}
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
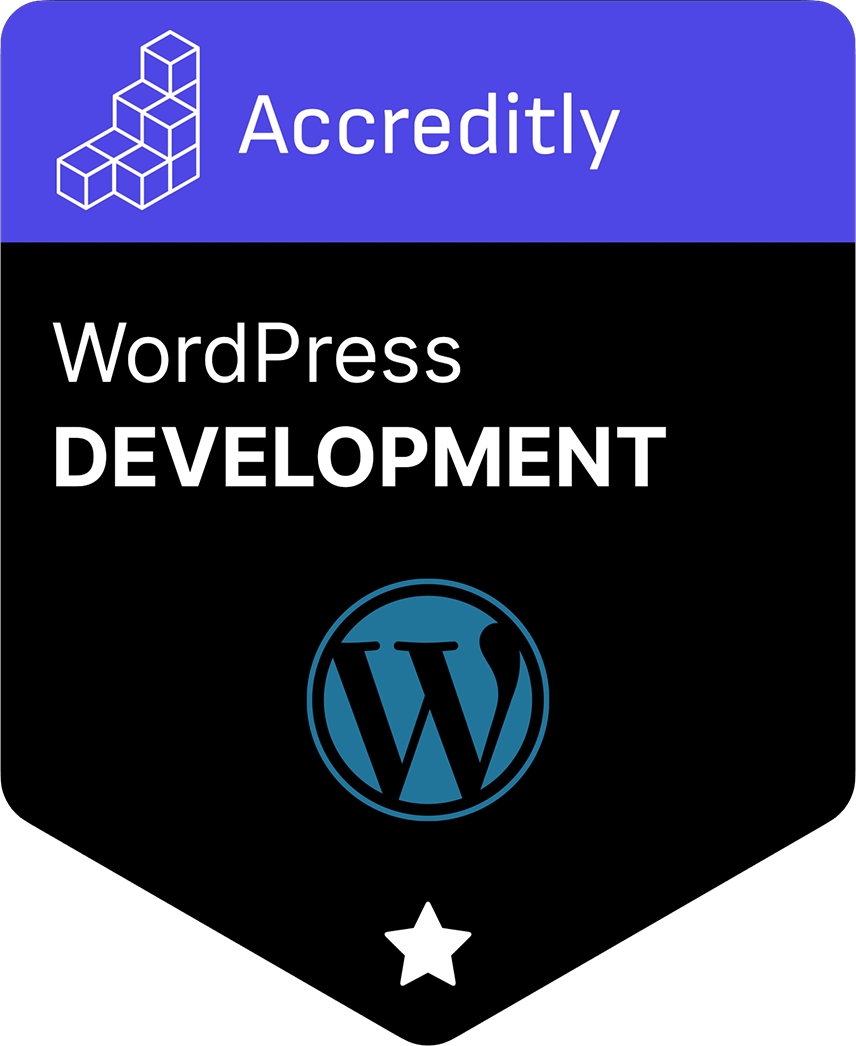