- Pre-facing: 'Vanilla' WordPress
- Prerequisites
- Step 1: Setting Up Your Development Environment
- Step 2: Create Your Block Plugin
- Step 3: Develop Your Block
- Step 4: Styling Your Carousel
- Step 5: Testing and Iteration
The Gutenberg editor, introduced in WordPress 5.0, revolutionized content creation by introducing blocks – a more visual and intuitive way to build and manage content. Among the myriad of available blocks, a carousel is a sought-after feature for displaying images, posts, or custom content in a sliding format. While numerous plugins offer carousel functionality, creating a custom carousel Gutenberg block in vanilla WordPress allows for tailored solutions without bloated extras. This article guides you through the process of building your own carousel block from scratch.
Pre-facing: 'Vanilla' WordPress
When we talk about 'vanilla' WordPress, we're talking about WordPress without any additional plugins installed. It's just plain old WordPress, just as it comes. No plugins, and most-definitely no page builders.
Prerequisites
Before diving into block development, ensure you have a basic understanding of JavaScript, PHP, and WordPress theme structure. Familiarity with React.js is beneficial, as Gutenberg leverages React for block development.
Step 1: Setting Up Your Development Environment
- WordPress Environment: Ensure you have a local or live WordPress environment setup.
- Node.js and NPM: Gutenberg block development requires Node.js and NPM for managing dependencies and running build processes.
Step 2: Create Your Block Plugin
Gutenberg blocks can be added directly to a theme, but encapsulating your block in a plugin is a best practice, ensuring portability and theme independence.
-
Create a Plugin Folder: In your WordPress installation, navigate to
wp-content/plugins
and create a new folder for your block, e.g.,my-carousel-block
. -
Plugin File: Inside this folder, create a PHP file with the same name,
my-carousel-block.php
, and add the plugin header:
<?php
/*
Plugin Name: My Carousel Block
Description: A custom Gutenberg block for creating carousels.
Version: 1.0
Author: Your Name
*/
- Register Block and Scripts: Register your block and enqueue JavaScript and CSS files necessary for the block. Add the following to your plugin file:
function my_carousel_block_register_block() {
wp_register_script(
'my-carousel-block-editor',
plugins_url('build/index.js', __FILE__),
array('wp-blocks', 'wp-element', 'wp-editor'),
filemtime(plugin_dir_path(__FILE__) . 'build/index.js')
);
wp_register_style(
'my-carousel-block-editor',
plugins_url('build/index.css', __FILE__),
array(),
filemtime(plugin_dir_path(__FILE__) . 'build/index.css')
);
register_block_type('my-carousel-block/carousel', array(
'editor_script' => 'my-carousel-block-editor',
'editor_style' => 'my-carousel-block-editor',
));
}
add_action('init', 'my_carousel_block_register_block');
Step 3: Develop Your Block
-
Block Structure: Use JavaScript (React.js) to define your block's structure, attributes, and editing interface. Gutenberg blocks are primarily developed in JavaScript using React.
-
Create
src
andbuild
Folders: Inside your plugin directory, create asrc
folder for your source files and abuild
folder for the compiled output. -
Block Code: Inside the
src
folder, createindex.js
. This file will contain your block's JavaScript code, including editor components and frontend output. Use React components to define the carousel's structure and functionality. -
Compiling Your Block: Use a build tool like Webpack or Parcel to compile your JSX and ES6 code into vanilla JavaScript. The compiled code should be output to the
build
folder. For simplicity, you can also use@wordpress/scripts
package to handle the build process.
npm init
npm install @wordpress/scripts --save-dev
Update your package.json
to include a build script:
"scripts": {
"build": "wp-scripts build"
}
Run npm run build
to compile your code.
Step 4: Styling Your Carousel
Define the carousel's styling in a CSS file (index.css
) in your src
directory. This can include transitions, controls styling, and responsive behavior. Compile this CSS using your chosen build tool, outputting to the build
directory alongside your JavaScript.
Step 5: Testing and Iteration
Activate your plugin from the WordPress dashboard and add the carousel block to a post or page. Test the block in both the editor and the frontend, iterating on its design and functionality based on feedback and requirements.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
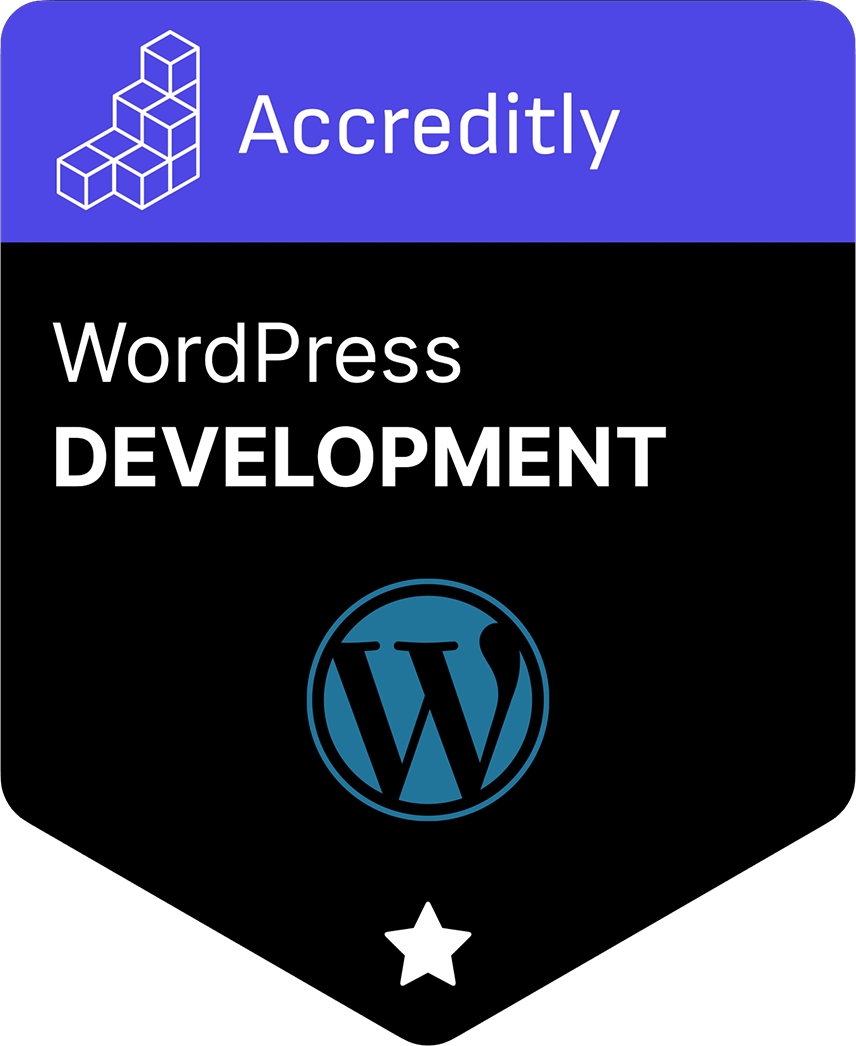