- Understanding the MediaDevices API
- Prerequisites
- Step 1: Check for API Availability
- Step 2: Request Camera Access
- Step 3: Display the Camera Stream
- Step 4: Capture a Still Image from the Stream
- Step 5: Handle Permissions and User Denial
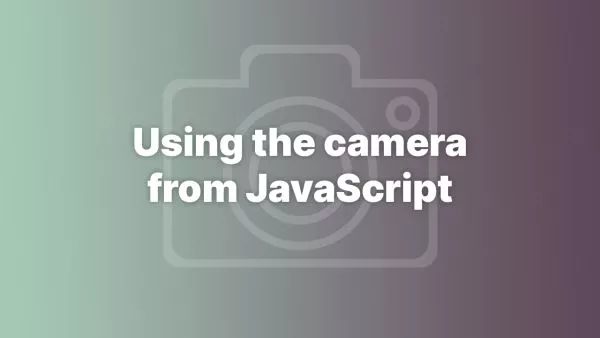
Accessing a device's camera through the browser has become a fundamental requirement. From social media platforms to QR code scanners, the ability to control the camera directly from a web page enhances functionality and user engagement. JavaScript, through the MediaDevices API, offers a streamlined approach to incorporating camera access into your web applications. This article explores the steps necessary to capture images or video from a user's camera using JavaScript.
Understanding the MediaDevices API
The MediaDevices
API is part of the WebRTC (Web Real-Time Communications) specification that provides access to connected media input devices like cameras and microphones. The getUserMedia()
method is particularly useful for requesting access to a user's camera, allowing for both still photography and video streaming within web applications.
Prerequisites
Before you begin, ensure your website is served over HTTPS. Modern browsers require a secure context for accessing media devices to protect user privacy.
Step 1: Check for API Availability
First, it's important to verify that the user's browser supports the MediaDevices
API. This can be done with a simple check:
if (navigator.mediaDevices && navigator.mediaDevices.getUserMedia) {
// MediaDevices API is available
} else {
alert("Camera API not supported by this browser.");
}
Step 2: Request Camera Access
You can request access to the camera using the getUserMedia()
method, which returns a Promise. This method takes a constraints object that specifies the type of media to request. For accessing the camera, we're interested in video:
const constraints = {
video: true
};
navigator.mediaDevices.getUserMedia(constraints)
.then(function(stream) {
// You have access to the stream
})
.catch(function(error) {
console.error("Error accessing the camera", error);
});
Step 3: Display the Camera Stream
Once access is granted, the next step is to display the camera stream. This is typically done by setting the srcObject
of a video element to the stream obtained from getUserMedia()
:
<video id="camera-stream" autoplay></video>
navigator.mediaDevices.getUserMedia({ video: true })
.then(function(stream) {
const videoElement = document.getElementById('camera-stream');
videoElement.srcObject = stream;
})
.catch(function(error) {
console.error("Error accessing the camera", error);
});
The autoplay
attribute on the video element ensures the video starts playing as soon as the stream is available.
Step 4: Capture a Still Image from the Stream
Capturing a still image from the camera stream involves drawing the current video frame to a canvas element, from which you can then export an image:
<canvas id="image-capture" style="display:none;"></canvas>
function captureImage() {
const canvas = document.getElementById('image-capture');
const context = canvas.getContext('2d');
const video = document.getElementById('camera-stream');
canvas.width = video.videoWidth;
canvas.height = video.videoHeight;
context.drawImage(video, 0, 0, canvas.width, canvas.height);
// Export the canvas image as data URL
const imageDataUrl = canvas.toDataURL('image/png');
// You can now use `imageDataUrl` for displaying or uploading purposes
}
Step 5: Handle Permissions and User Denial
It's crucial to handle scenarios where a user denies camera access or when permissions have not been granted for the device. The promise rejection from getUserMedia()
should be used to inform the user or adjust the UI accordingly:
navigator.mediaDevices.getUserMedia({ video: true })
.then(function(stream) {
/* Stream available */
})
.catch(function(error) {
if (error.name === "NotAllowedError") {
alert("Camera access was denied.");
}
});
Accessing the camera with JavaScript opens up a number of possibilities for enhancing web applications with real-time media capabilities. By following the steps outlined in this guide, developers can seamlessly integrate camera functionality, creating more interactive and engaging user experiences. Always remember to respect user privacy and permissions when accessing camera devices, ensuring a secure and trustworthy environment for your users.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
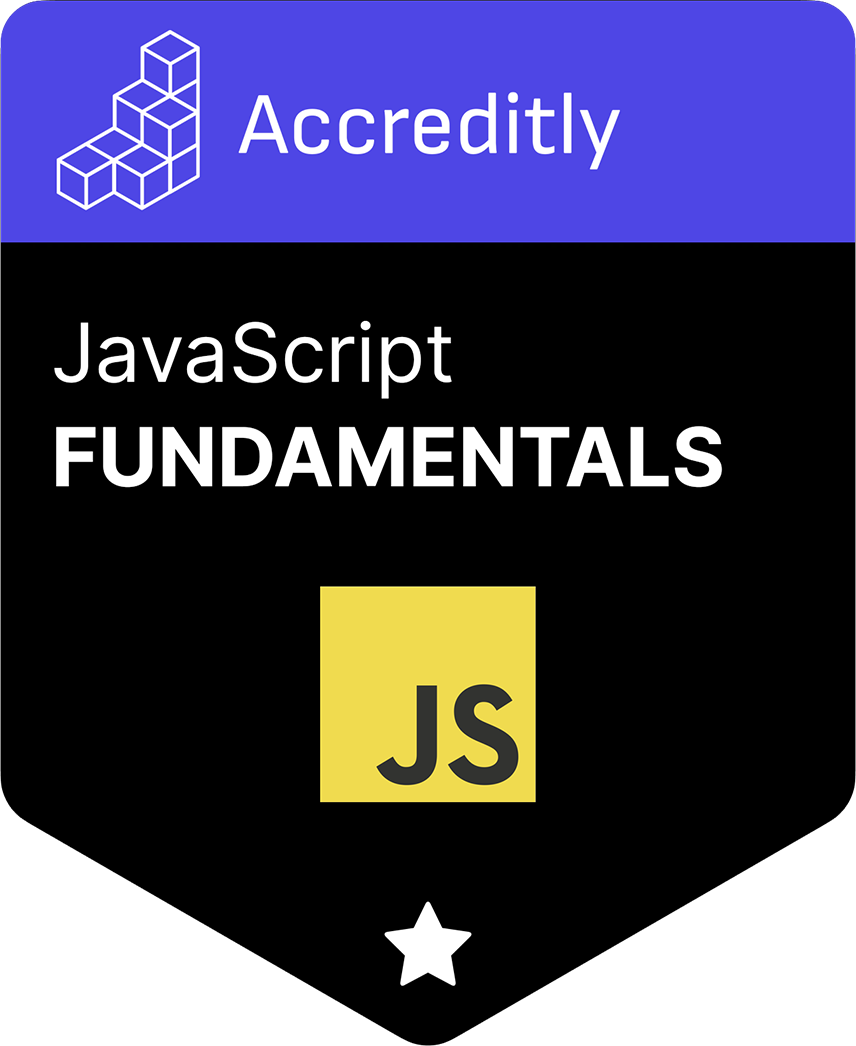