- What is the N+1 Problem?
- A WordPress Example of the N+1 Problem
- Why is the N+1 Problem Bad?
- Identifying the N+1 Problem in WordPress
- Avoiding the N+1 Problem in WordPress
- Advanced Techniques: Optimizing Custom Queries
- Wrapping up
The N+1 problem is a performance issue that can affect any database-driven application, including WordPress. Even though WordPress is a powerful and flexible content management system, the N+1 problem can still arise, especially when dealing with custom queries, themes, or plugins that interact heavily with the database. In this article, we’ll explore what the N+1 problem is in the context of WordPress, how it can impact your website’s performance, and strategies to avoid it.
What is the N+1 Problem?
The N+1 problem occurs when an application makes N additional database queries to retrieve related data for N objects that have already been fetched. This issue is common in situations where data is retrieved lazily—one piece at a time—rather than all at once in a single, optimized query.
In WordPress, this problem can arise in themes, plugins, or custom code that performs database queries in an inefficient manner.
A WordPress Example of the N+1 Problem
To better understand how the N+1 problem can manifest in a WordPress context, let’s consider a scenario where you have a custom post type called events
, and each event has associated attendees
stored as custom post meta.
Imagine you want to display a list of all events along with the names of their attendees. A naive approach in a WordPress theme’s template.php
file might look like this:
$events = get_posts([
'post_type' => 'events',
'numberposts' => -1
]);
foreach ($events as $event) {
$attendees = get_post_meta($event->ID, 'attendees', true);
echo '<h2>' . esc_html($event->post_title) . '</h2>';
foreach ($attendees as $attendee) {
echo '<p>' . esc_html($attendee) . '</p>';
}
}
While this code might seem straightforward, it can lead to the N+1 problem. Here’s why:
- The initial
get_posts()
call fetches all events in a single query. - For each event, the
get_post_meta()
function is called to retrieve its attendees.
If there are 10 events, this code will execute 11 database queries—one to fetch the events and 10 additional queries to fetch the attendees for each event. This results in the N+1 problem, where N is the number of events.
Why is the N+1 Problem Bad?
The N+1 problem can lead to significant performance issues on your WordPress site, particularly as the number of records increases. Here are some reasons why this problem is detrimental:
-
Increased Database Load: Each additional database query adds load to your database server, which can slow down your site, especially if it’s under heavy traffic.
-
Slower Page Load Times: Multiple database queries can slow down page load times, leading to a poor user experience. This is especially critical for WordPress sites where performance is key to user retention and SEO.
-
Scalability Issues: As your site grows and the number of posts or custom data increases, the N+1 problem can become more severe, making your site less scalable and harder to maintain.
Identifying the N+1 Problem in WordPress
To identify the N+1 problem in WordPress, you need to monitor the number of queries being executed during a page load. WordPress provides several tools and plugins to help with this:
1. Query Monitor Plugin
The Query Monitor plugin is a powerful tool for debugging and monitoring WordPress performance. It allows you to see all database queries executed during a page load, making it easier to spot the N+1 problem.
After installing and activating Query Monitor, navigate to any page on your site. In the admin bar, you’ll see a Query Monitor link showing the number of queries executed. Clicking on it will display detailed information about each query, helping you identify if multiple similar queries are being made.
2. Debug Bar Plugin
The Debug Bar plugin is another useful tool that can help you track down the N+1 problem. It provides a debug bar in the WordPress admin area where you can see the number of database queries, memory usage, and other useful metrics.
By using these tools, you can pinpoint where the N+1 problem is occurring in your WordPress code and take steps to optimize it.
Avoiding the N+1 Problem in WordPress
Once you’ve identified the N+1 problem, the next step is to optimize your code to avoid it. Here are some strategies to prevent the N+1 problem in WordPress:
1. Batch Processing and Preloading
Instead of fetching related data for each individual post or object, try to retrieve all the related data in a single query or in batches. In the example above, instead of calling get_post_meta()
for each event, you can preload all the necessary metadata in one query using get_post_meta()
with multiple IDs:
$event_ids = wp_list_pluck($events, 'ID');
$meta_values = get_post_meta($event_ids, 'attendees', true);
foreach ($events as $event) {
$attendees = $meta_values[$event->ID] ?? [];
echo '<h2>' . esc_html($event->post_title) . '</h2>';
foreach ($attendees as $attendee) {
echo '<p>' . esc_html($attendee) . '</p>';
}
}
This reduces the number of queries from N+1 to just two—one for the events and one for the meta values.
meta_query
2. Use WP_Query with When working with custom post types and meta data, you can optimize queries using WP_Query
with a meta_query
. This approach can often eliminate the need for separate queries to retrieve related meta data:
$events = new WP_Query([
'post_type' => 'events',
'meta_query' => [
[
'key' => 'attendees',
'compare' => 'EXISTS'
]
]
]);
while ($events->have_posts()) {
$events->the_post();
$attendees = get_post_meta(get_the_ID(), 'attendees', true);
echo '<h2>' . get_the_title() . '</h2>';
if ($attendees) {
foreach ($attendees as $attendee) {
echo '<p>' . esc_html($attendee) . '</p>';
}
}
}
wp_reset_postdata();
This method allows WordPress to optimize the query internally, reducing the chances of an N+1 problem.
3. Utilize WordPress Transients
If the data doesn’t change frequently, consider using WordPress transients to cache the results of expensive queries. This approach can significantly reduce database load and improve performance:
$events = get_transient('cached_events_with_attendees');
if (false === $events) {
$events = get_posts([
'post_type' => 'events',
'numberposts' => -1
]);
foreach ($events as $event) {
$event->attendees = get_post_meta($event->ID, 'attendees', true);
}
set_transient('cached_events_with_attendees', $events, 12 * HOUR_IN_SECONDS);
}
// Display the cached data
foreach ($events as $event) {
echo '<h2>' . esc_html($event->post_title) . '</h2>';
if (!empty($event->attendees)) {
foreach ($event->attendees as $attendee) {
echo '<p>' . esc_html($attendee) . '</p>';
}
}
}
By caching the events and their attendees, you avoid repeated database queries on subsequent requests, which can help mitigate the N+1 problem.
Advanced Techniques: Optimizing Custom Queries
For more complex scenarios, consider using advanced query optimization techniques, such as custom SQL queries or leveraging WordPress’s built-in functions like update_meta_cache()
or get_post_custom()
. These functions allow you to preload and batch process meta data more effectively.
Wrapping up
The N+1 problem is a common issue that can negatively impact the performance of your WordPress site, but it’s avoidable with careful planning and optimization. By understanding how and why the N+1 problem occurs, and by implementing strategies like batch processing, eager loading, and caching, you can ensure that your WordPress site remains fast, scalable, and capable of handling large amounts of data efficiently.
Whether you’re developing custom themes, plugins, or working with complex datasets, being mindful of the N+1 problem will help you create more efficient WordPress applications that provide a better experience for your users.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
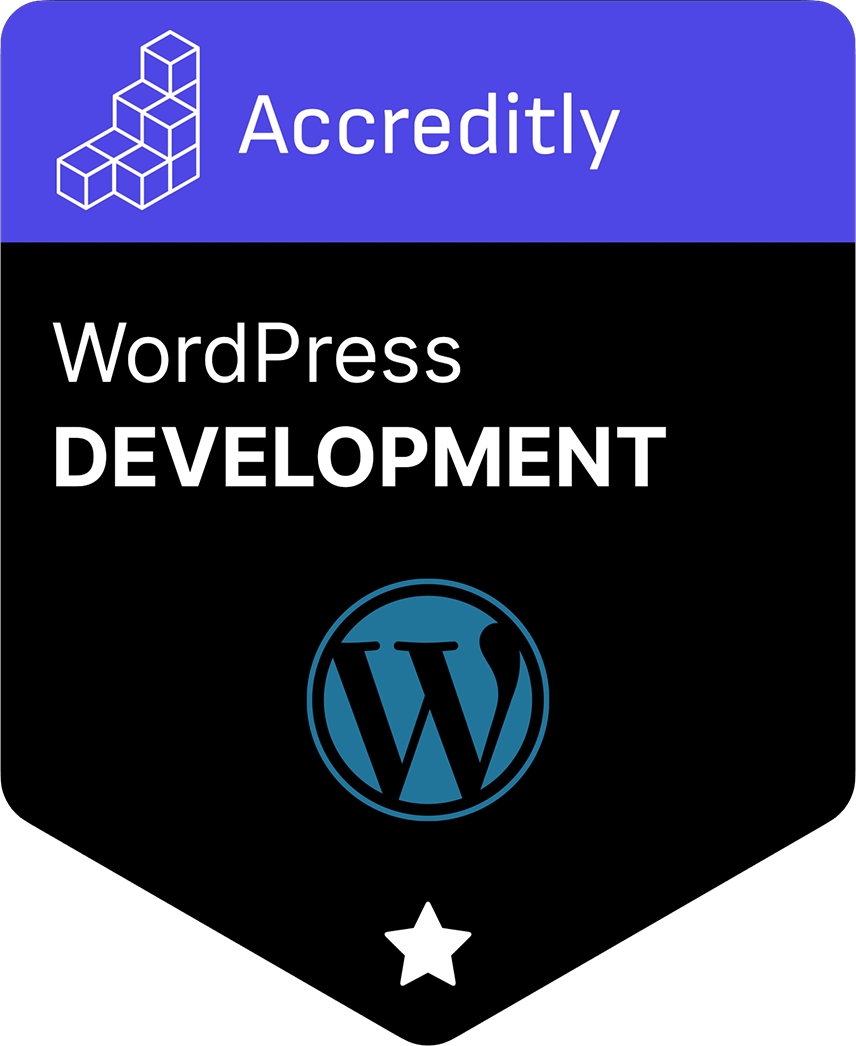
Related articles
Tutorials PHP Database Design Tooling
When and how to squash migrations
Learn about squashing migrations in Laravel, a pivotal technique for optimizing your application's efficiency and maintainability. This guide covers the why behind migration squashing and provides a tutorial on implementing it.