- 1. Classic For Loop
- 2. Array.prototype.indexOf
- 3. Array.prototype.find
- 4. Array.prototype.findIndex
- 5. Array.prototype.includes
- 6. Array.prototype.filter
- 7. Using the some Method
- 8. Using the every Method
- 9. ES6 Destructuring and indexOf
- 10. Using reduce
JavaScript provides a number of methods to search through arrays - those fundamental structures that store lists of values. Whether you're a novice coder or a seasoned developer, understanding the different ways to search an array can greatly enhance your coding toolkit. Let's explore the various paths one can take to find that needle in the JavaScript haystack.
1. Classic For Loop
The time-honored for
loop is the Swiss Army knife for array searching. It's straightforward and offers the most control.
let fruits = ["apple", "banana", "cherry"];
let searchElement = "banana";
let foundIndex = -1;
for (let i = 0; i < fruits.length; i++) {
if (fruits[i] === searchElement) {
foundIndex = i;
break;
}
}
console.log(foundIndex); // Output: 1
2. Array.prototype.indexOf
[]indexOf
](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/indexOf) is a method that returns the first index at which a given element can be found, or -1 if it's not present.
let fruits = ["apple", "banana", "cherry"];
console.log(fruits.indexOf("banana")); // Output: 1
3. Array.prototype.find
find
executes a function for each array element and returns the first element that matches the condition.
let fruits = ["apple", "banana", "cherry"];
let result = fruits.find(fruit => fruit === "banana");
console.log(result); // Output: "banana"
4. Array.prototype.findIndex
Similar to find
, but instead of returning the element, it returns its index.
let fruits = ["apple", "banana", "cherry"];
let foundIndex = fruits.findIndex(fruit => fruit === "banana");
console.log(foundIndex); // Output: 1
5. Array.prototype.includes
This method checks if an array includes a certain value among its entries, returning true or false as appropriate.
let fruits = ["apple", "banana", "cherry"];
console.log(fruits.includes("banana")); // Output: true
6. Array.prototype.filter
filter
creates a new array with all elements that pass the test implemented by the provided function.
let fruits = ["apple", "banana", "cherry", "banana"];
let allBananas = fruits.filter(fruit => fruit === "banana");
console.log(allBananas); // Output: ["banana", "banana"]
some
Method
7. Using the The some
method tests whether at least one element in the array passes the test implemented by the provided function.
let fruits = ["apple", "banana", "cherry"];
let hasBanana = fruits.some(fruit => fruit === "banana");
console.log(hasBanana); // Output: true
every
Method
8. Using the every
is the strict cousin of some
, checking if all elements in the array pass the test.
let fruits = ["banana", "banana", "banana"];
let allBananas = fruits.every(fruit => fruit === "banana");
console.log(allBananas); // Output: true
9. ES6 Destructuring and indexOf
Combine ES6 destructuring with indexOf
to search for an element in a more complex array structure.
let fruits = [{ name: "apple" }, { name: "banana" }, { name: "cherry" }];
let searchElement = { name: "banana" };
let foundIndex = fruits.map(fruit => fruit.name).indexOf(searchElement.name);
console.log(foundIndex); // Output: 1
reduce
10. Using While not traditionally used for searching, reduce
can accumulate elements matching a condition.
let fruits = ["apple", "banana", "cherry"];
let foundBananas = fruits.reduce((acc, fruit) => {
if (fruit === "banana") acc.push(fruit);
return acc;
}, []);
console.log(foundBananas); // Output: ["banana"]
Searching through arrays is a common operation in JavaScript, and as shown, there are many tools at our disposal. Each method offers different benefits, and the best choice depends on the specific requirements of the task at hand. By understanding these varied approaches, JavaScript developers can write more efficient and readable code that perfectly aligns with their array-searching needs.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
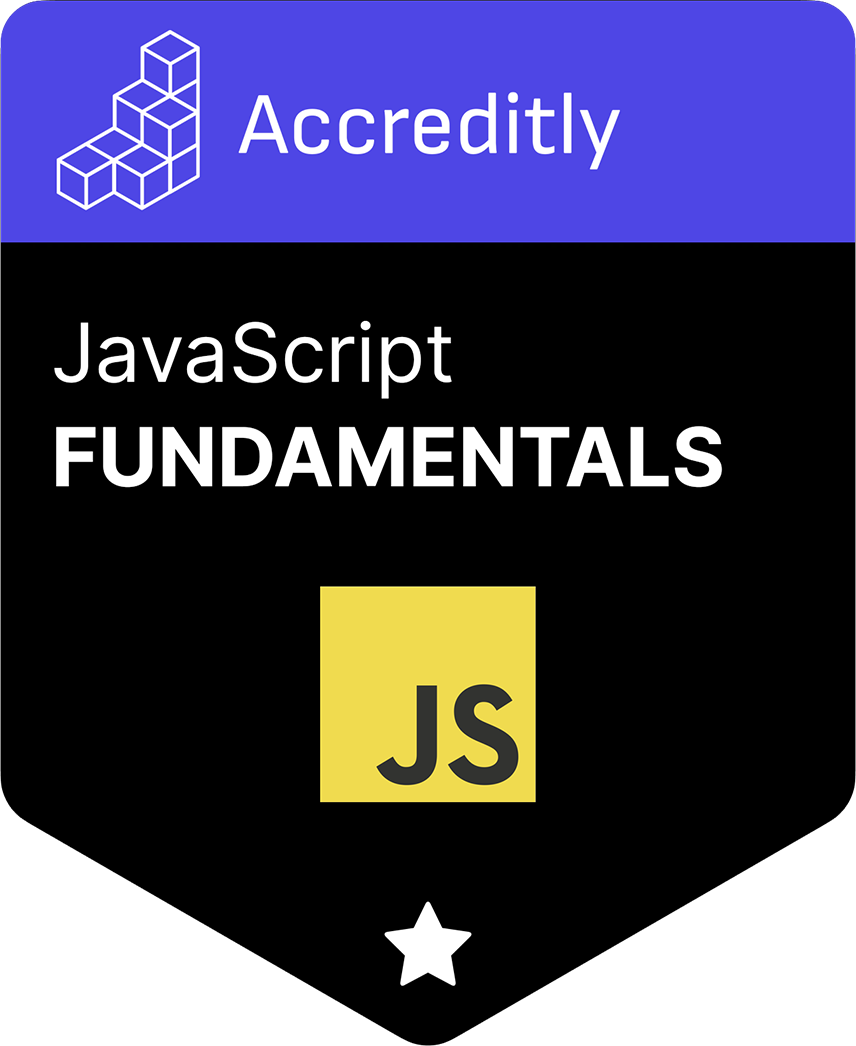
Related articles
Tutorials PHP Database Design Tooling
When and how to squash migrations
Learn about squashing migrations in Laravel, a pivotal technique for optimizing your application's efficiency and maintainability. This guide covers the why behind migration squashing and provides a tutorial on implementing it.