- Basics of Exception Handling in PHP
- The finally Block
- Creating Custom Exceptions
- Best Practices for Exception Handling in PHP
Exception handling is an essential aspect of programming, as it helps developers manage errors and control the flow of a program when unexpected issues arise. PHP, like many other programming languages, provides robust exception handling capabilities to help you build more reliable and maintainable applications.
The PHP docs do cover exception handling, but it isn't a tutorial. This article aims to take you through exception handling in more detail, with some examples to make things as clear as possible.
In this detailed guide, we'll explore exception handling in PHP, covering topics like using try-catch blocks, creating custom exceptions, and best practices for managing errors in your applications. By the end of this article, you'll have a solid understanding of how to handle exceptions effectively in PHP.
Basics of Exception Handling in PHP
Exceptions are events that occur when an error is encountered during the execution of a program. In PHP, exceptions are represented by the Exception
class, which contains information about the error, such as the error message, the file where the error occurred, and the line number.
To handle exceptions in PHP, you'll use try-catch blocks. The try
block contains the code that might throw an exception, while the catch
block is responsible for handling the exception if it occurs. Here's a basic example:
try {
// Code that might throw an exception
$result = 10 / 0;
} catch (Exception $e) {
// Handle the exception
echo "Caught exception: " . $e->getMessage();
}
In the example above, dividing by zero causes an exception to be thrown. The catch block catches the exception and displays a message to the user.
The finally Block
In addition to the try-catch block, PHP also supports a finally
block, which is executed regardless of whether an exception is thrown or not. This can be useful for cleaning up resources or performing other tasks that need to be done regardless of the outcome. Here's an example:
try {
// Code that might throw an exception
$result = 10 / 0;
} catch (Exception $e) {
// Handle the exception
echo "Caught exception: " . $e->getMessage();
} finally {
// Code in the finally block will always be executed
echo "The finally block has been executed.";
}
In this example, the message "The finally block has been executed." will be displayed even if an exception occurs.
Creating Custom Exceptions
PHP allows you to create custom exception classes by extending the base Exception
class. This can be useful for creating more specific exception types that provide additional information or functionality. Here's an example of a custom exception class:
class MyCustomException extends Exception {
public function customFunction() {
echo "This is a custom function for this exception.";
}
}
try {
// Code that might throw an exception
throw new MyCustomException("A custom exception has occurred.");
} catch (MyCustomException $e) {
// Handle the custom exception
echo "Caught MyCustomException: " . $e->getMessage();
$e->customFunction();
}
In this example, we create a custom exception class called MyCustomException
that extends the Exception
class and adds a customFunction method. When the custom exception is thrown and caught, the customFunction
method is called.
Best Practices for Exception Handling in PHP
Here are some best practices to keep in mind when handling exceptions in PHP:
- Be specific with your exceptions.
Instead of catching general Exception objects, catch specific exception types that you expect to be thrown. This helps you handle each exception in a more targeted and efficient way.
try {
// Code that might throw an exception
$result = 10 / 0;
} catch (DivisionByZeroError $e) {
// Handle the specific exception
echo "Caught DivisionByZeroError: " . $e->getMessage();
} catch (Exception $e) {
// Handle other general exceptions
echo "Caught exception: " . $e->getMessage();
}
- Create custom exceptions for better error handling.
Creating custom exceptions for specific error cases allows you to handle them more effectively and provides better context for debugging. Make use of custom exceptions when appropriate.
- Don't suppress exceptions.
Suppressing exceptions (using the @
operator) can make it difficult to identify and debug issues in your code. Instead, handle exceptions properly using try-catch blocks and provide meaningful error messages to the user.
- Log exceptions.
While displaying error messages to users can be helpful, it's also essential to log exceptions for debugging purposes. Use a logging library or PHP's built-in error logging functionality to record exceptions and other errors.
- Use the finally block for cleanup.
If you have code that needs to be executed regardless of whether an exception is thrown, use the finally
block to ensure it runs even in case of an error.
- Handle exceptions at the right level.
Catch and handle exceptions at the appropriate level of your application. In some cases, it might be better to let an exception bubble up to a higher level where it can be handled more effectively.
Use meaningful exception messages: When creating custom exceptions or throwing built-in exceptions, provide a meaningful error message that helps identify the cause of the problem. This will make it easier to debug and understand the issue.
Exception handling is a crucial aspect of PHP programming that helps you build more reliable and maintainable applications. By understanding how to use try-catch blocks, creating custom exceptions, and following best practices for managing errors, you can improve your PHP code and create more robust applications.
In this detailed guide, we've explored exception handling in PHP, covering topics like using try-catch blocks, creating custom exceptions, and best practices for managing errors in your applications. With this knowledge, you can effectively handle exceptions and build more reliable PHP applications.
If you understand exceptions in PHP then our PHP fundamentals certification will be great for you, as exception and error handling is a core part of the exam.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
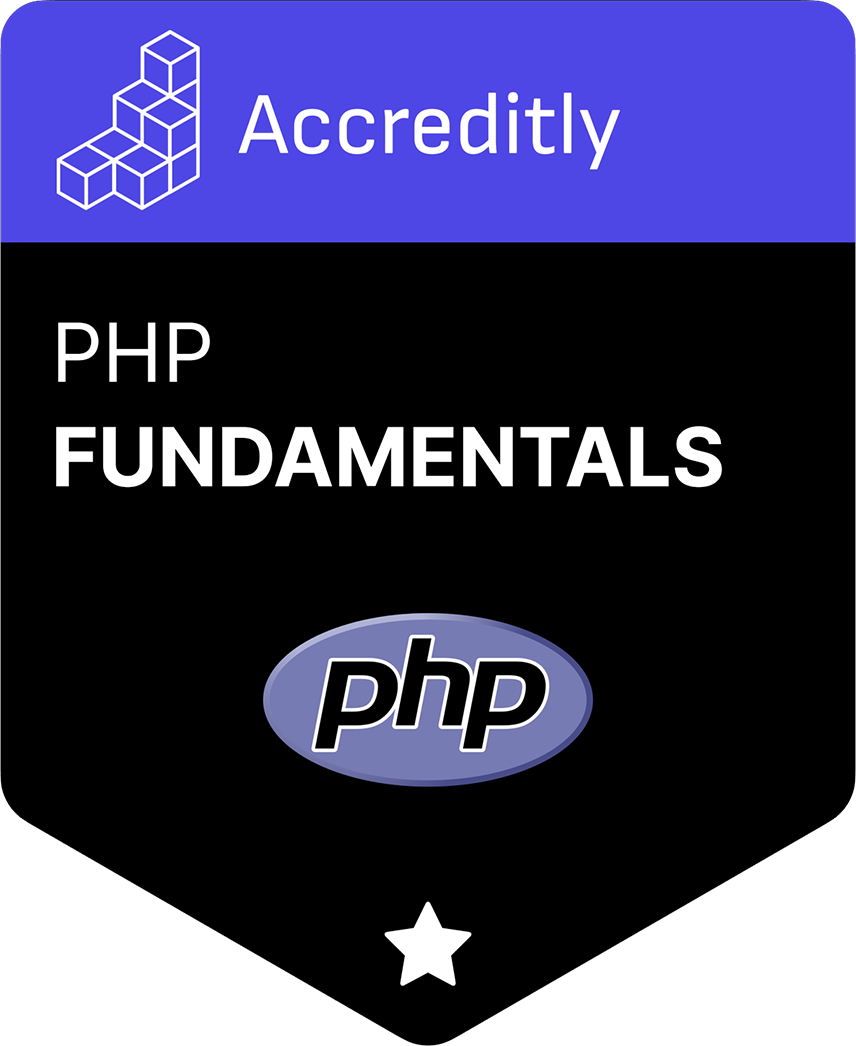