- Understanding the Geolocation API
- Prerequisites
- Step 1: Check for Geolocation Support
- Step 2: Obtain the Current Location
- Step 3: Implement Real-Time Location Tracking
- Step 4: Handling Permissions and Errors
- Best Practices
- Summary
Accessing real-time location data has become a cornerstone for personalized user experiences. Whether it's for location-based services, tracking, or simply enhancing user interaction, geolocation can significantly uplift your web project's value. JavaScript, with its Geolocation API, provides a powerful yet straightforward way to harness this capability. Here’s how to get started with real-time location tracking in your web applications.
Understanding the Geolocation API
The Geolocation API is a part of the Navigator object, available in the global window scope of the browser. It allows access to geographical position data, offering several methods to retrieve the device's current location. Notably, getCurrentPosition()
and watchPosition()
are the primary functions used to obtain location data, with the latter providing real-time tracking capabilities.
Prerequisites
- Ensure your website is served over HTTPS, as modern browsers require a secure context for Geolocation API access.
- Familiarize yourself with handling asynchronous operations in JavaScript, as retrieving location data involves Promise-based API calls.
Step 1: Check for Geolocation Support
Before attempting to access location data, check if the user's browser supports the Geolocation API:
if ('geolocation' in navigator) {
// Geolocation API is supported
} else {
console.log('Geolocation API not supported by this browser.');
}
Step 2: Obtain the Current Location
The getCurrentPosition()
method fetches the device's current position. It takes three parameters: a success callback, an optional error callback, and optional position options.
navigator.geolocation.getCurrentPosition(position => {
console.log('Latitude:', position.coords.latitude);
console.log('Longitude:', position.coords.longitude);
}, error => {
console.error('Error obtaining location:', error);
});
Step 3: Implement Real-Time Location Tracking
For applications requiring continuous location updates, watchPosition()
becomes invaluable. This method works similarly to getCurrentPosition()
but provides ongoing updates to the user's position as they move.
const watchId = navigator.geolocation.watchPosition(position => {
console.log('Updated Latitude:', position.coords.latitude);
console.log('Updated Longitude:', position.coords.longitude);
}, error => {
console.error('Error obtaining location:', error);
}, {
enableHighAccuracy: true,
timeout: 5000,
maximumAge: 0
});
// To stop watching the position
// navigator.geolocation.clearWatch(watchId);
Step 4: Handling Permissions and Errors
Effective error handling and permissions management are crucial for a seamless user experience. The Geolocation API provides error codes to help identify issues like permission denials or timeouts.
function errorHandler(error) {
switch(error.code) {
case error.PERMISSION_DENIED:
console.error("User denied the request for Geolocation.");
break;
case error.POSITION_UNAVAILABLE:
console.error("Location information is unavailable.");
break;
case error.TIMEOUT:
console.error("The request to get user location timed out.");
break;
default:
console.error("An unknown error occurred.");
break;
}
}
Best Practices
- Always ensure the user is aware of and consents to location tracking. The Geolocation API inherently prompts the user for permission, but it’s good practice to precede this with an explanation of why and how the location data will be used.
- Handle location data with care. Be transparent with your users about data collection practices and adhere to privacy laws and regulations.
- Use the
options
parameter to control the frequency and accuracy of location updates according to your application's needs. Adjusting these can help conserve battery life on mobile devices.
Summary
Real-time location tracking in JavaScript opens up a plethora of possibilities for web developers to create engaging, personalized user experiences. By leveraging the Geolocation API, you can introduce a variety of location-based features into your web applications, from user tracking to localized content. Remember, the key to successful implementation lies in respecting user privacy, ensuring accuracy, and handling permissions gracefully. With these considerations in mind, you're well on your way to integrating powerful real-time geolocation functionality into your projects.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
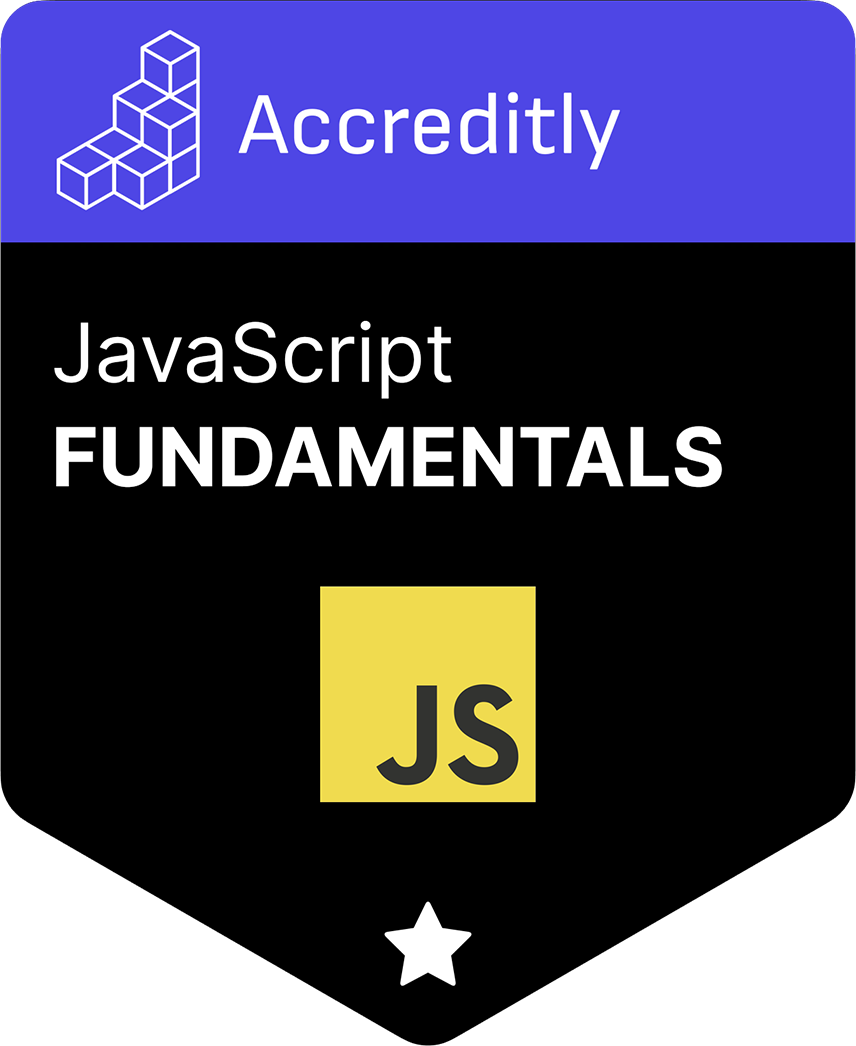