- Prerequisites
- Step 1: Set Up Your Mux Account and API Keys
- Step 2: Install the Mux PHP SDK
- Step 3: Initialize the Mux PHP SDK
- Step 4: Create a Live Stream
- Step 5: Embed the Video Player
- Step 6: Start Streaming with Your Streaming Software
- Summary and troubleshooting
- Possible use cases
Mux is a powerful video platform that offers developers easy-to-use APIs for managing and streaming live video content. Content can be normal video files where Mux handles transcoding and delivery, but can also be live streaming.
In this tutorial, we'll guide you through the process of using Mux APIs and SDK with PHP to live stream video. We'll cover setting up your Mux account, installing the PHP SDK, creating a live stream, and embedding the video player on your website.
Prerequisites
Before we begin, make sure you have the following:
- A Mux account: Sign up for a Mux account at https://mux.com.
- PHP installed on your machine: PHP 8.1 or higher is recommended, but PHP 7.2 will work (although is at end of life at time of writing).
- Composer: Install Composer, a dependency manager for PHP, from https://getcomposer.org.
Step 1: Set Up Your Mux Account and API Keys
First, sign in to your Mux account and navigate to the API Access section (Settings > Access Tokens). Generate a new API token by clicking on "Generate new token". Make sure to grant both "Mux Video" and "Mux Data" permissions, and copy the "Access Token ID" and "Secret Key" for later use.
Step 2: Install the Mux PHP SDK
To install the Mux PHP SDK, use Composer. Open a terminal and run the following command:
composer require muxinc/mux-php
This command installs the Mux PHP SDK and its dependencies.
Step 3: Initialize the Mux PHP SDK
Create a new PHP file, mux.php
, and include the following code to initialize the Mux PHP SDK:
<?php
require_once 'vendor/autoload.php';
use MuxPhp\Configuration;
use MuxPhp\Api\AssetsApi;
use MuxPhp\Api\LiveStreamsApi;
use MuxPhp\Models\CreateAssetRequest;
use MuxPhp\Models\InputSettings;
use MuxPhp\Models\CreateLiveStreamRequest;
use MuxPhp\Models\PlaybackPolicy;
// Set up the Mux Configuration with your API keys
$config = Configuration::getDefaultConfiguration()
->setUsername('<YOUR_ACCESS_TOKEN_ID>')
->setPassword('<YOUR_SECRET_KEY>');
// Create instances of the Mux APIs
$assetsApi = new AssetsApi(
new GuzzleHttp\Client(),
$config
);
$liveStreamsApi = new LiveStreamsApi(
new GuzzleHttp\Client(),
$config
);
Replace <YOUR_ACCESS_TOKEN_ID>
and <YOUR_SECRET_KEY>
with the API keys from your Mux account in step 1.
Step 4: Create a Live Stream
To create a live stream, add the following code to your mux.php
file:
// Set up the live stream settings
$liveStreamRequest = new CreateLiveStreamRequest();
$liveStreamRequest->setPlaybackPolicy([PlaybackPolicy::PUBLIC_PLAYBACK_POLICY]);
// Create the live stream
$liveStream = $liveStreamsApi->createLiveStream($liveStreamRequest);
// Save the playback ID and stream key
$playbackId = $liveStream->getData()->getPlaybackIds()[0]->getId();
$streamKey = $liveStream->getData()->getStreamKey();
echo "Live Stream created:\n";
echo "Playback ID: {$playbackId}\n";
echo "Stream Key: {$streamKey}\n";
This code creates a public live stream and prints the playback ID and stream key. The stream key is required to configure your streaming software (e.g., OBS, Streamlabs), while the playback ID is used to generate the playback URL for your video player.
Step 5: Embed the Video Player
To embed the video player on your website, create an HTML file, index.html, and include the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Live Stream</title>
<style>
#video-player {
width: 100%;
height: 100%;
max-width: 640px;
max-height: 360px;
}
</style>
</head>
<body>
<video id="video-player" controls>
<source src="https://stream.mux.com/<PLAYBACK_ID>.m3u8" type="application/x-mpegURL">
Your browser does not support the video tag.
</video>
</body>
</html>
Replace <PLAYBACK_ID>
with the playback ID from the previous step. Save the file and open it in your browser to see the video player. The player will show the live stream when you start streaming using your streaming software. You may need to refresh this page after you complete the next step.
Step 6: Start Streaming with Your Streaming Software
Configure your streaming software with the following settings:
- Stream Type: RTMP
- Server URL:
rtmp://global-live.mux.com:5222/app/
- Stream Key: The stream key obtained in Step 4.
Start streaming from your software, and the live stream should appear in the video player on your website. You may need to refresh the page to see it (index.html
).
Summary and troubleshooting
If you encounter any problems check out the Mux dashboard and look at the API activity log, it often shows where things might be going wrong. Streaming video can be intensive on both your computer and your internet connection, so diagnosing problems can often be more challenging than integrating normal APIs.
Problems with the API itself can be diagnosed via Mux's dashboard, which keeps a log of all activity. Everything else will require knowledge of your network and infrastructure. Your streaming software will also give you some pointers should something go wrong.
Possible use cases
There are many applications for this tech. Previously this would have required a lot of infrastructure, both on-premise and in the cloud, whereas with tools such as Mux and advances in streaming software, it's possible to build a live streaming platform from within your own application.
The use cases are vast, but could include anything from building your own streaming service like Twitch or YouTube Live, to branding up and owning the full experience of an in-house webinar.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
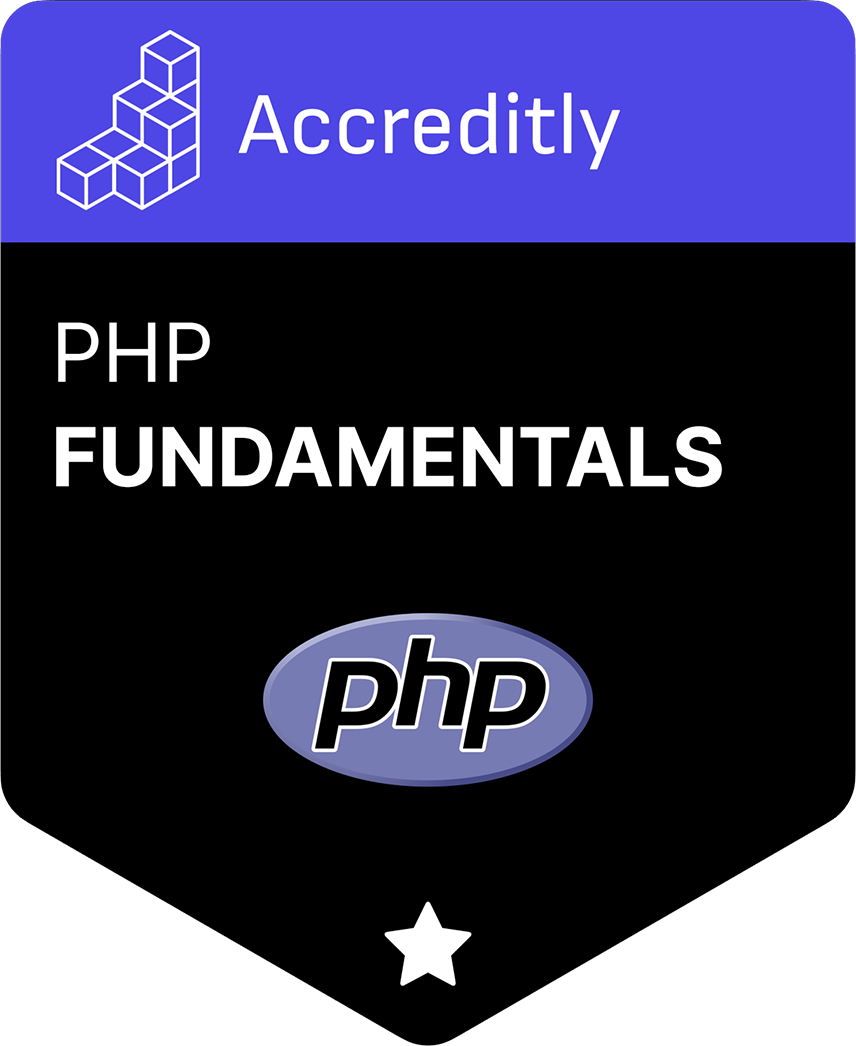
Related articles
Tutorials WordPress Database Design Tooling
How to store and output currency values in WordPress
Effectively manage currency values in WordPress, from storage best practices to displaying currency amounts on your website. This guide covers everything to ensure accurate and user-friendly currency handling in WordPress.