- Overview
- Syntax
- Basic Example
- Advanced Use Cases
- Handling Async Operations:
- Things to Remember:
- Conclusion:
JavaScript is renowned for its robust array manipulation capabilities, and among its many useful methods, .map()
stands out for its power and flexibility. This guide will delve deep into understanding the .map()
method, showcasing its syntax, use cases, and providing practical examples to illuminate its functionality.
Overview
The .map()
method creates a new array populated with the results of calling a provided function on every element in the calling array. It doesn't change the original array but returns a new array that represents the results.
Syntax
Here's the basic syntax for the .map()
method in JavaScript:
let newArray = arr.map(callback(currentValue[, index[, array]]) {
// return element for newArray
}, thisArg);
Where:
-
arr
is the array to be mapped over. -
callback
is the function to execute on each element. -
currentValue
is the current element being processed. -
index
(optional) is the index of thecurrentValue
in the array. -
array
(optional) is the arraymap
was called upon. -
thisArg
(optional) is the value to use asthis
when executingcallback
.
Basic Example
Let's start with a basic example:
const numbers = [1, 2, 3, 4];
const squares = numbers.map(number => number ** 2);
console.log(squares); // Output: [1, 4, 9, 16]
In this example, the .map()
method iterates over each element in the numbers
array and squares each number. The result is a new array, squares
.
Advanced Use Cases
Mapping Objects:
You can use the .map()
method to iterate through an array of objects and modify the objects:
const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 22 },
{ name: 'Jim', age: 32 }
];
const userNames = users.map(user => user.name);
console.log(userNames); // Output: ['John', 'Jane', 'Jim']
Chainability:
.map()
can be chained with other array methods for more complex operations:
const numbers = [1, 2, 3, 4];
const doubledEvenNumbers = numbers
.filter(number => number % 2 === 0)
.map(number => number * 2);
console.log(doubledEvenNumbers); // Output: [4, 8]
In this example, .filter()
is used to select only even numbers, and .map()
then doubles them.
Handling Async Operations:
The .map()
method can also work with async/await patterns:
const urls = ['url1', 'url2', 'url3'];
const fetchData = async () => {
const responses = await Promise.all(urls.map(async url => {
const response = await fetch(url);
return response.json();
}));
console.log(responses);
}
fetchData();
Things to Remember:
- Does Not Mutate Original Array:
.map()
does not change the original array, it returns a new array. - Same Length as Original: The returned array will always be the same length as the original array.
- Use When a New Array is Needed: Use
.map()
when you want to transform elements of an array and create a new array.
Conclusion:
The .map()
method in JavaScript is a powerful tool for processing arrays and should be a part of every developer's toolkit. By understanding its syntax, behavior, and advanced use cases, developers can write cleaner, more readable code, and efficiently manipulate array data in JavaScript.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
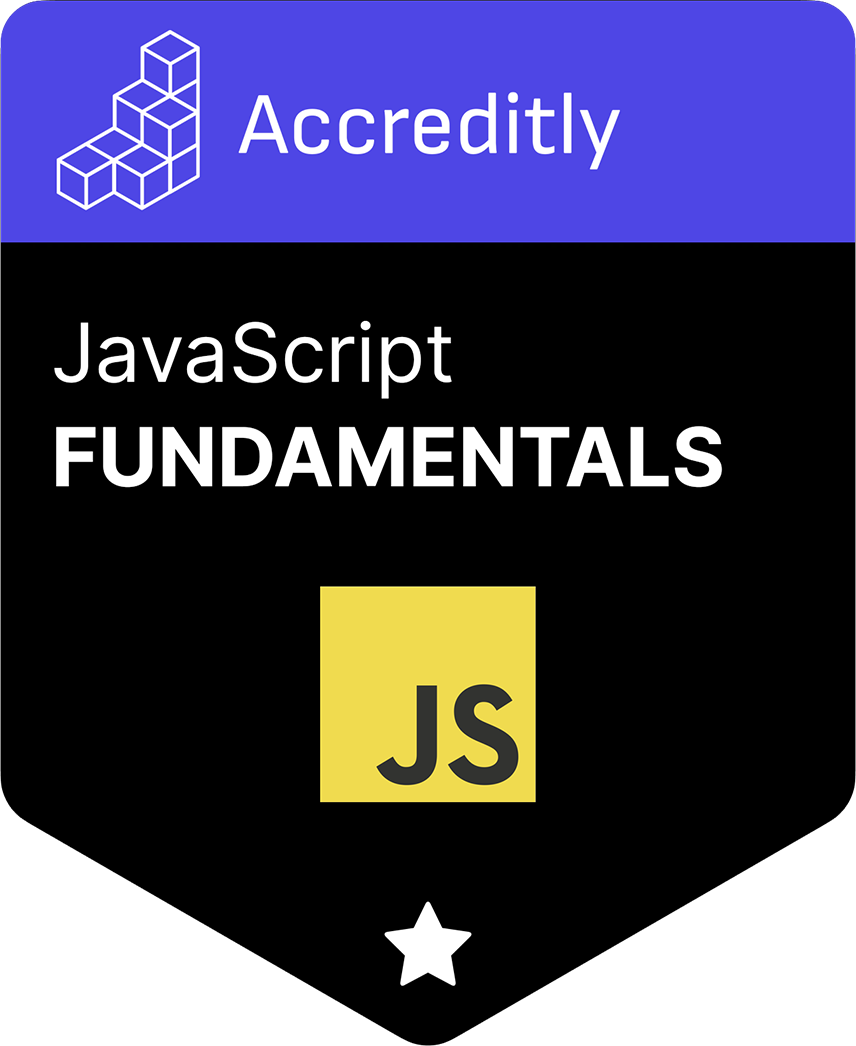