- 1. What is a Form Request?
- 2. Generating a Form Request
- 3. Specifying Validation Rules
- 4. Handling Authorization
- 5. Customizing Error Messages
- 6. Using the Form Request in Controllers
- 7. Benefits of Using Form Requests
Handling form data can often be messy. Ensuring data validation and possibly authorization can litter your controller with logic that doesn't necessarily belong there. Laravel addresses this problem with a feature known as Form Requests. This powerful tool allows developers to separate the concerns of form validation and data authorization from the main application logic.
1. What is a Form Request?
A Form Request is a custom request class that contains validation and authorization logic. It is especially useful when you have a form, or any type of user input, that needs validation before proceeding.
2. Generating a Form Request
Using Laravel's Artisan command-line tool, you can generate a Form Request:
php artisan make:request StoreBlogPost
This will create a new request file in app/Http/Requests/StoreBlogPost.php
.
3. Specifying Validation Rules
Inside the generated request class, you will find a method named rules
. This is where you define your validation rules:
public function rules() {
return [
'title' => 'required|max:255',
'body' => 'required',
];
}
4. Handling Authorization
For scenarios where you also need to authorize the action (e.g., updating a blog post), you can use the authorize
method:
public function authorize() {
$post = $this->route('post');
return $post && $this->user()->can('update', $post);
}
In this example, before the post is updated, the application checks if the authenticated user has the permission to update the post.
5. Customizing Error Messages
To provide custom error messages for the defined validation rules, override the messages
method:
public function messages() {
return [
'title.required' => 'A title is mandatory',
'body.required' => 'Don't forget the post body!',
];
}
6. Using the Form Request in Controllers
Once you have defined a Form Request, you can type-hint it in your controller methods:
public function store(StoreBlogPost $request) {
// The incoming request is valid...
// You can access validated data with $request->validated()
}
If the validation rules or the authorize method fail, Laravel will automatically redirect the user back to their previous location, and all the validation errors will be available in the session.
7. Benefits of Using Form Requests
- Cleaner Controllers: By extracting validation and authorization logic, controllers become more focused on their primary responsibility.
- Reusability: Form Requests can be reused across multiple controllers or methods, promoting the DRY (Don't Repeat Yourself) principle.
- Centralized Logic: Having a dedicated place for validation and authorization logic can make the application more maintainable.
Laravel's Form Requests provide an elegant solution to handle form validation and data authorization. By leveraging this feature, developers can write more maintainable, cleaner, and more efficient code. Whether you're working on a small project or a large-scale application, incorporating Form Requests can significantly improve the structure and clarity of your Laravel application.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
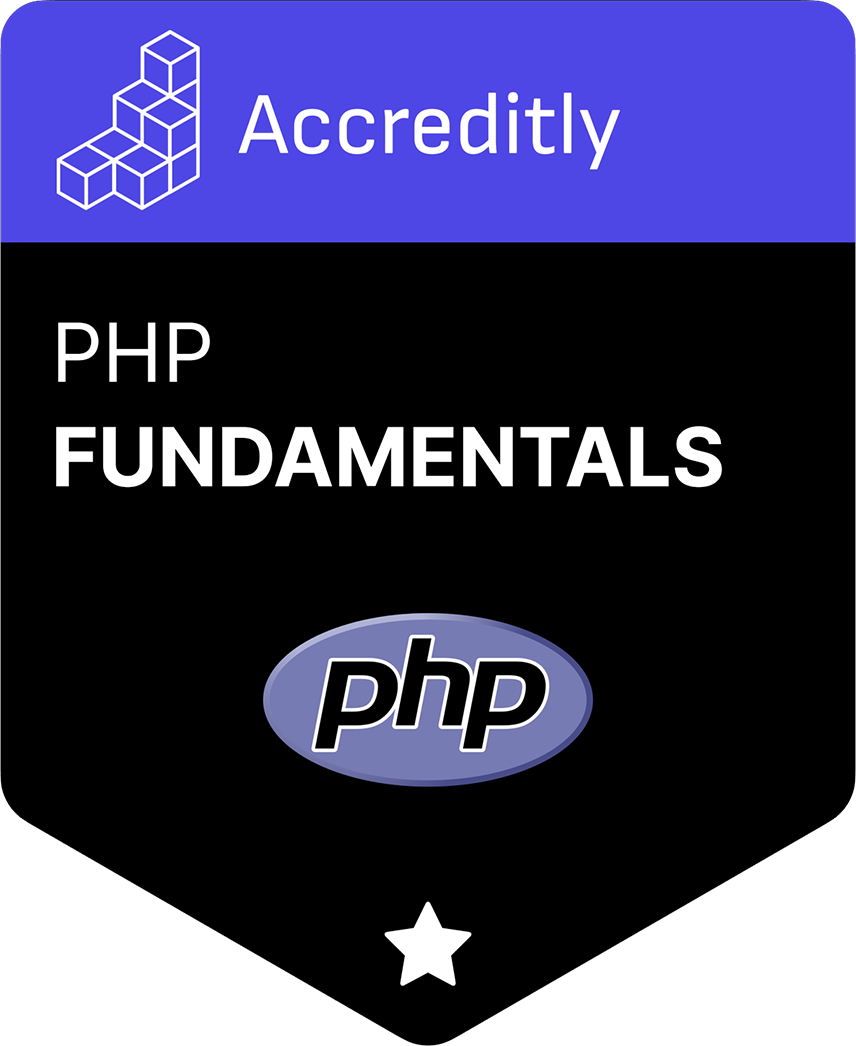