- Understanding the Basics
- Step 1: Design Your Audio Player
- Step 2: Create the Audio Player HTML Structure
- Step 3: Enhance with CSS
- Step 4: Add Functionality with JavaScript
- Step 5: Embedding Your Audio Player in WordPress
Audio content can significantly enhance the user experience on your WordPress site, whether it's for showcasing your music, sharing podcasts, or providing auditory feedback. While WordPress supports audio files out of the box with its built-in audio shortcode, creating a custom audio player allows for greater control over the playback experience and the visual presentation of your audio content. This guide will outline how to implement your own audio player in WordPress, from concept to execution.
Understanding the Basics
The foundation of a custom audio player in WordPress is the HTML <audio>
element, which allows for the embedding of sound content in web pages. This element supports various audio formats, including MP3, OGG, and WAV, ensuring wide compatibility across different browsers.
Step 1: Design Your Audio Player
Before diving into the code, consider how you want your audio player to look and function. Key features might include play/pause buttons, volume control, a progress bar, and a download option. Designing your player in a user-friendly manner will enhance the overall experience.
Step 2: Create the Audio Player HTML Structure
Start by crafting the HTML structure for your player. You'll likely want to include the following:
- Container: A div that wraps the entire player.
- Audio Element: The core of the player, which will play the audio file.
- Controls: Custom buttons and sliders for controlling playback, volume, etc.
<div id="custom-audio-player">
<audio id="audio" preload="none">
<source src="path/to/your-audio.mp3" type="audio/mp3">
Your browser does not support the audio tag.
</audio>
<button id="playPause">Play/Pause</button>
<input type="range" id="volumeControl" min="0" max="1" step="0.01">
<progress id="progressBar" value="0" max="100"></progress>
</div>
Step 3: Enhance with CSS
Styling your audio player with CSS is crucial for making it visually appealing and consistent with your site's design. Customize the appearance of the player container, buttons, and progress bar to match your aesthetic.
#custom-audio-player {
/* Player styles */
}
#playPause, #volumeControl {
/* Button and volume slider styles */
}
#progressBar {
/* Progress bar styles */
}
Step 4: Add Functionality with JavaScript
To make your audio player functional, you'll need to write some JavaScript. This script will handle play/pause actions, volume adjustments, and updating the progress bar as the audio plays.
document.addEventListener('DOMContentLoaded', function() {
var audio = document.getElementById('audio');
var playPause = document.getElementById('playPause');
var volumeControl = document.getElementById('volumeControl');
var progressBar = document.getElementById('progressBar');
playPause.addEventListener('click', function() {
if (audio.paused) {
audio.play();
} else {
audio.pause();
}
});
volumeControl.addEventListener('input', function() {
audio.volume = this.value;
});
audio.addEventListener('timeupdate', function() {
var progress = (audio.currentTime / audio.duration) * 100;
progressBar.value = progress;
});
});
Step 5: Embedding Your Audio Player in WordPress
To include your custom audio player in WordPress, you have a few options:
-
Custom Page Template or Theme File: If you're comfortable with PHP, you can directly insert your HTML, CSS, and JavaScript into a custom page template or a specific theme file (
single.php
,page.php
, etc.). -
Gutenberg Block: For a more user-friendly approach, consider creating a custom Gutenberg block that allows you to easily insert your audio player anywhere on your site. This requires knowledge of React and PHP.
-
Shortcode: Another flexible option is to create a WordPress shortcode that outputs your audio player. This allows you to embed the player using the
[yourshortcode]
syntax in posts, pages, and widgets.
function custom_audio_player_shortcode() {
ob_start();
?>
<!-- Your audio player HTML here -->
<?php
return ob_get_clean();
}
add_shortcode('custom_audio_player', 'custom_audio_player_shortcode');
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
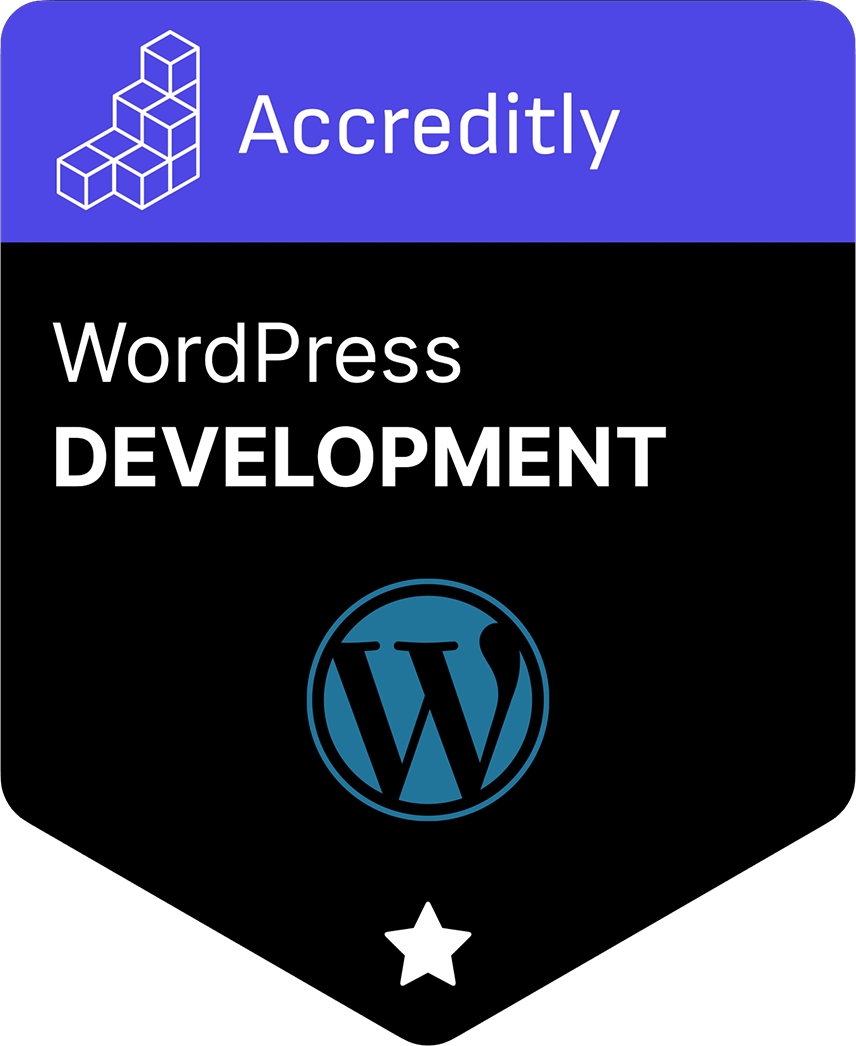