- Step 1: Prepare Your Images
- Step 2: Create a Text File with Image Paths
- Step 3: Use FFmpeg to Create the Video
This tutorial will guide you through the process of creating a video from a series of images using PHP and FFmpeg. FFmpeg is a powerful tool that allows programmers to convert, stream digital audio, and video in numerous formats. PHP will be used for managing the images and invoking the FFmpeg commands.
Note: To follow along, make sure you have FFmpeg installed on your system and accessible from your PHP environment.
Step 1: Prepare Your Images
The first step is to prepare the images you want to transform into a video. Let's assume you have a series of images named image1.jpg
, image2.jpg
, image3.jpg
, and so on, stored in a directory named images
.
$dir = '/path/to/your/images/directory/';
Step 2: Create a Text File with Image Paths
FFmpeg can read from a text file to get the list of images to use for the video. This text file will contain the file path for each image.
$fileContent = '';
foreach (glob($dir . '*.jpg') as $filename) {
$fileContent .= 'file \'' . $filename . "'\n";
}
file_put_contents($dir . 'files.txt', $fileContent);
Step 3: Use FFmpeg to Create the Video
Now that you have a text file listing all the images, you can use FFmpeg to create the video. The shell_exec()
function in PHP can be used to execute the FFmpeg command.
$output = $dir . 'output.mp4';
$frameRate = 1; // Change this to adjust the frame rate
$cmd = "ffmpeg -f concat -r $frameRate -i {$dir}files.txt -c:v libx264 $output";
shell_exec($cmd);
In the FFmpeg command:
-
-f concat
tells FFmpeg to concatenate the input files. -
-r $frameRate
sets the frame rate of the video (frames per second). -
-i {$dir}files.txt
specifies the input file that contains the list of images. -
-c:v libx264
specifies the codec to be used for the video.
As you can see from the code we're actually just calling FFmpeg from the command line via PHP, rather than actually using PHP to generate the video file. The downside to this is that feedback from the command will need to be parsed to understand the outcome of the encoding.
You should also be aware of the security risks of using shell_exec()
, and that many hosting providers block the use of the function all together.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
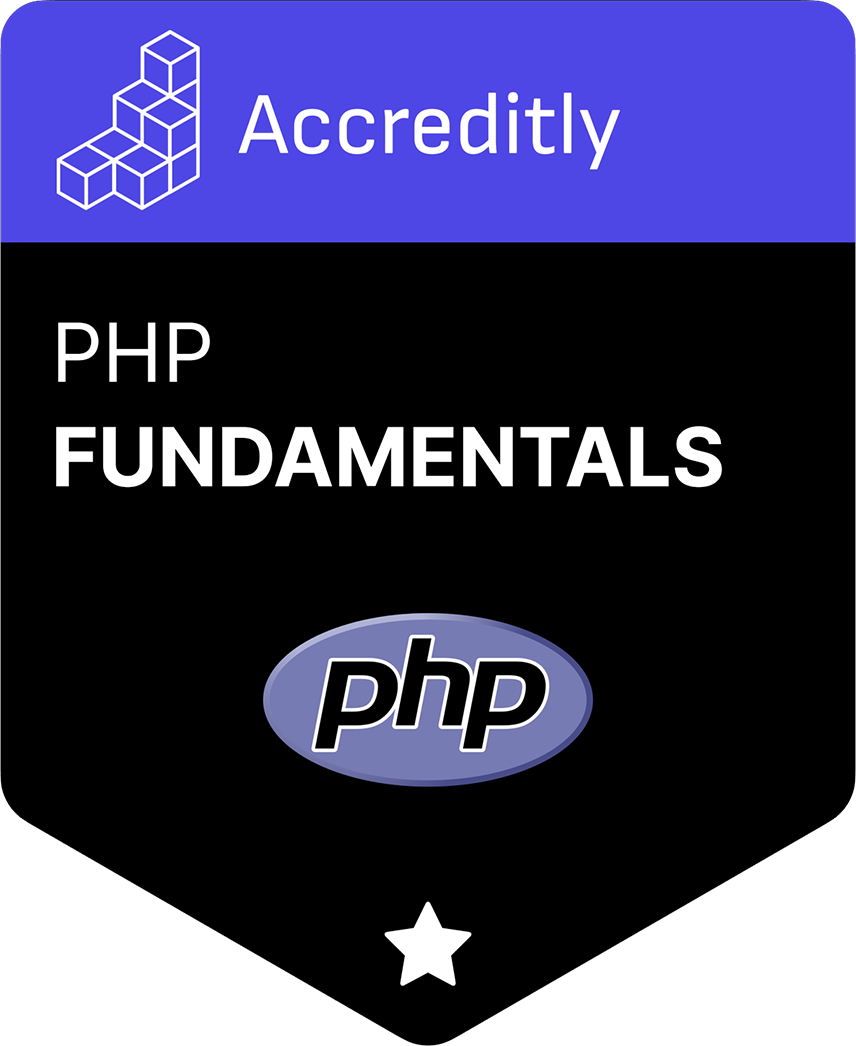