- Browser Developer Tools
- Console.log and Console Functions
- Breakpoints
- Debugging Statements
- Watch Expressions
- Call Stack and Scope
- Debugging with External Tools
- Summary
Debugging is an essential skill for any developer, and JavaScript is no exception. As your applications grow in complexity, it's crucial to have a solid understanding of the tools and techniques available for debugging JavaScript code. In this article, we'll explore various tools and techniques for debugging JavaScript applications, including browser developer tools, breakpoints, and debugging utilities.
Browser Developer Tools
Modern web browsers come with a powerful set of built-in developer tools that can help you debug JavaScript code. Most developer tools include a console, debugger, and other useful utilities for inspecting and modifying your application at runtime.
Example:
-
Google Chrome: Press
F12
orCtrl + Shift + I
(Windows/Linux) orCmd + Opt + I
(Mac) to open the Chrome Developer Tools. -
Mozilla Firefox: Press
F12
orCtrl + Shift + I
(Windows/Linux) orCmd + Opt + I
(Mac) to open the Firefox Developer Tools. -
Safari: Press
Cmd + Opt + I
to open the Web Inspector in Safari. Note that you'll need to enable the Develop menu in Preferences > Advanced > Show Develop menu in menu bar.
From here you'll have access to a plethora of tools, from profilers, to the console, and tons more. What is available differs depending on the browser, with Chrome and Firefox's developer tools being particularly useful. We'll look into some of the available tools in this article.
Console.log and Console Functions
The console.log()
function is a simple but powerful tool for logging messages and values to the browser console. You can use it to print the values of variables, log messages, and display the results of expressions.
In addition to console.log()
, there are other useful console functions, such as console.error()
, console.warn()
, and console.info()
, which can help you categorize your console messages.
We have an article available on dev.to that goes into much more detail on the console
methods available.
Example:
const x = 10;
const y = 20;
console.log('x:', x); // Output: x: 10
console.error('An error occurred'); // Output: An error occurred
console.warn('This is a warning'); // Output: This is a warning
console.info('This is an info message'); // Output: This is an info message
Breakpoints
Breakpoints allow you to pause the execution of your JavaScript code at specific points, enabling you to inspect the values of variables, modify the application state, and step through your code. You can set breakpoints using browser developer tools.
Example:
In the Chrome Developer Tools or Firefox Developer Tools:
- Open the Sources (Chrome) or Debugger (Firefox) panel.
- Navigate to the JavaScript file where you want to set a breakpoint.
- Click on the line number where you want to pause execution.
Debugging Statements
The debugger;
statement is a programmatic way to set a breakpoint in your JavaScript code. When the browser encounters this statement and developer tools are open, it will pause the execution of your code at that point, just like a breakpoint.
Checkout MDN for docs on debugger;
Example:
function sum(a, b) {
debugger; // Execution will pause here
return a + b;
}
console.log(sum(1, 2)); // Output: 3
Watch Expressions
Watch expressions are a feature in browser developer tools that allow you to monitor the values of variables or expressions as your code executes. They can be useful for tracking the state of your application and understanding how variables change over time.
Example:
In the Chrome Developer Tools or Firefox Developer Tools:
- Open the Sources (Chrome) or Debugger (Firefox) panel.
- Click on the "Watch" tab (Chrome) or "Watch Expressions" section (Firefox).
- Click "Add Expression" (Chrome) or the "+" button (Firefox) and enter a variable name or expression you want to watch.
Call Stack and Scope
When debugging JavaScript code, it's important to understand the call stack and the scope of your variables. The call stack shows the order of function calls that led to the current point in your code, while the scope displays the values of variables in different contexts.
Example:
In the Chrome Developer Tools or Firefox Developer Tools:
- Pause the execution of your code using a breakpoint or the
debugger;
statement. - Open the "Call Stack" (Chrome) or "Call Stack & Scopes" (Firefox) panel to view the current call stack and the scope of your variables.
Debugging with External Tools
There are also external tools and libraries available that can help you debug JavaScript code. These tools often provide additional functionality beyond what's included in browser developer tools, such as advanced logging, error handling, and performance analysis.
Examples:
- Visual Studio Code: This popular code editor includes a built-in JavaScript debugger that allows you to set breakpoints, step through your code, and inspect variables directly from the editor.
- Sentry: Sentry is an error tracking service that can help you monitor and fix crashes in real-time. It provides detailed error reports, including stack traces and user information, making it easier to reproduce and fix issues.
Summary
Debugging JavaScript applications can be a challenging task, but with the right tools and techniques, you can quickly identify and fix issues in your code. By mastering browser developer tools, breakpoints, watch expressions, and external debugging utilities, you'll be well-equipped to tackle even the most complex JavaScript applications. Remember to take advantage of these powerful debugging tools to streamline your development process and create more reliable, efficient, and maintainable code.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
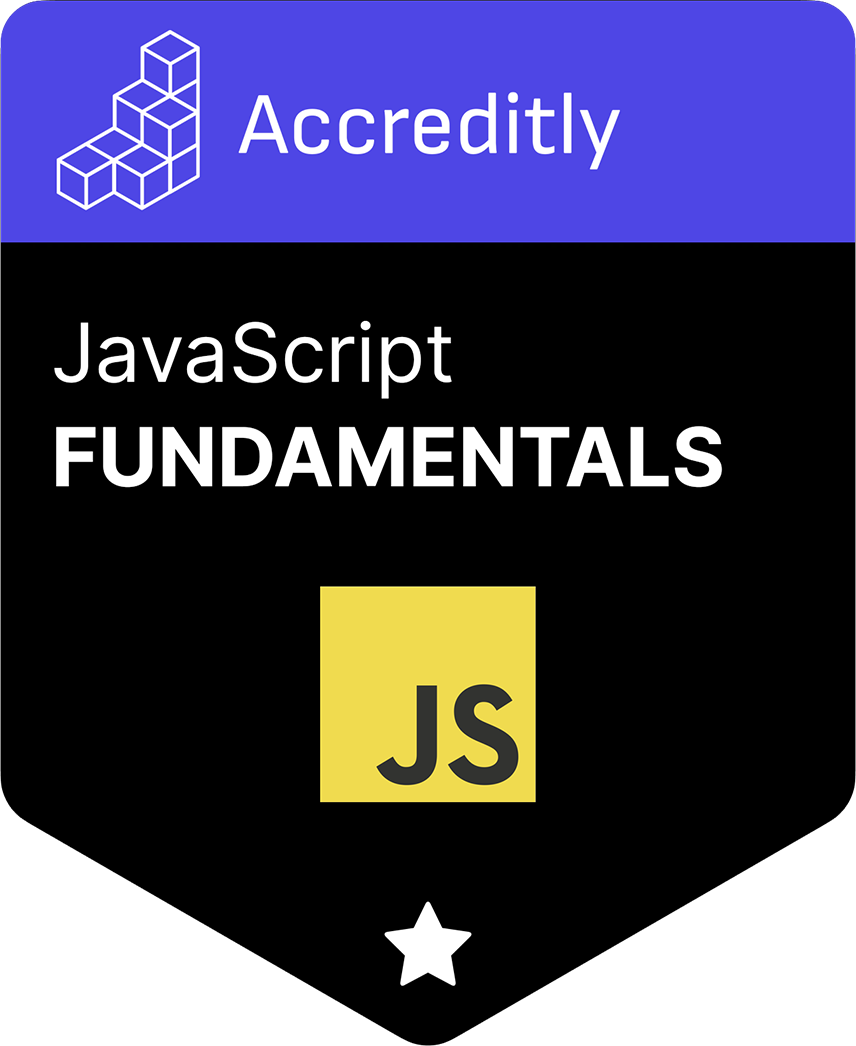