- Understanding the Read and Write Pattern
- Prerequisites
- Step 1: Configure Database Connections
- Step 2: Route Reads and Writes Appropriately
- Step 3: Testing Your Configuration
- Step 4: Handling Replication Lag
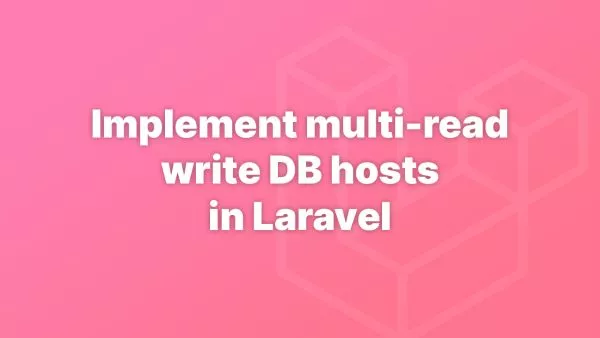
Laravel offers an elegant and straightforward way to interact with databases through Eloquent ORM and the database query builder. For applications experiencing heavy traffic or requiring high availability, implementing a read and write (replication) database pattern can significantly enhance performance. This pattern involves separating database queries into reads (SELECT statements) that go to a read replica, and writes (INSERT, UPDATE, DELETE statements) that go to the primary database. In effect you're able to run multiple database instances for reads, and have another database connection for writes. This tutorial guides you through setting up and utilizing this pattern in Laravel.
Understanding the Read and Write Pattern
Before diving into the implementation, it's essential to understand the concept. A read and write database pattern (or master-slave replication) involves one database server handling all write operations and one or more replicas handling read operations. This separation helps in distributing the load, thereby increasing application performance and availability.
Prerequisites
- Laravel application set up and running.
- Primary database and at least one read replica configured.
- Basic knowledge of Laravel's database and Eloquent ORM system.
Step 1: Configure Database Connections
First, you need to define your database connections in Laravel's config/database.php
configuration file. Here, you'll specify both your primary (write) and replica (read) database connections.
'mysql' => [
'read' => [
'host' => [
'192.168.1.1', // Replica database host
],
],
'write' => [
'host' => [
'196.168.1.2', // Primary database host
],
],
'driver' => 'mysql',
'database' => 'database_name',
'username' => 'database_username',
'password' => 'database_password',
'sticky' => true,
'prefix' => '',
],
In this configuration, read
and write
arrays under the mysql
connection specify the hosts for your read replica and primary database, respectively. The sticky
option, when set to true
, ensures that once a write operation has occurred during the current request lifecycle, further reads for the request are directed to the write connection. This is crucial for data consistency after writes.
Step 2: Route Reads and Writes Appropriately
With Laravel, you don't have to manually specify which connection to use for each query. Laravel intelligently routes database queries to the appropriate connection based on the type of operation:
-
Read Operations: By default, read operations (e.g.,
SELECT
) are routed to theread
connection. -
Write Operations: Write operations (e.g.,
INSERT
,UPDATE
,DELETE
) are automatically routed to thewrite
connection.
However, you can explicitly specify the connection for a particular database operation if needed:
// Using the 'read' connection
$users = DB::connection('mysql::read')->select(...);
// Using the 'write' connection
DB::connection('mysql::write')->update(...);
Step 3: Testing Your Configuration
To ensure your read and write database pattern is correctly implemented, you can perform write operations and verify that subsequent reads reflect the changes, considering any replication lag. Also, monitor the performance to see the distribution of queries between your primary and replica databases.
Step 4: Handling Replication Lag
One challenge with read replicas is the potential for replication lag, where the replica briefly falls behind the primary database. This can cause recently written data to appear missing from read operations. Strategies to mitigate this include:
- Application Logic: Implement logic in your application to detect critical write operations and force reads from the primary database when necessary.
- Database Configuration: Optimize your database configuration for faster replication.
Implementing a read and write database pattern in Laravel can dramatically improve your application's performance and scalability. By efficiently distributing database operations across multiple servers, you ensure that your application can handle increased loads and provide a seamless user experience. Remember, the key to a successful implementation is thorough testing and monitoring to fine-tune your configuration and address any replication lag issues. With Laravel's built-in support for database read and write separation, you're well-equipped to take your application's performance to the next level.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
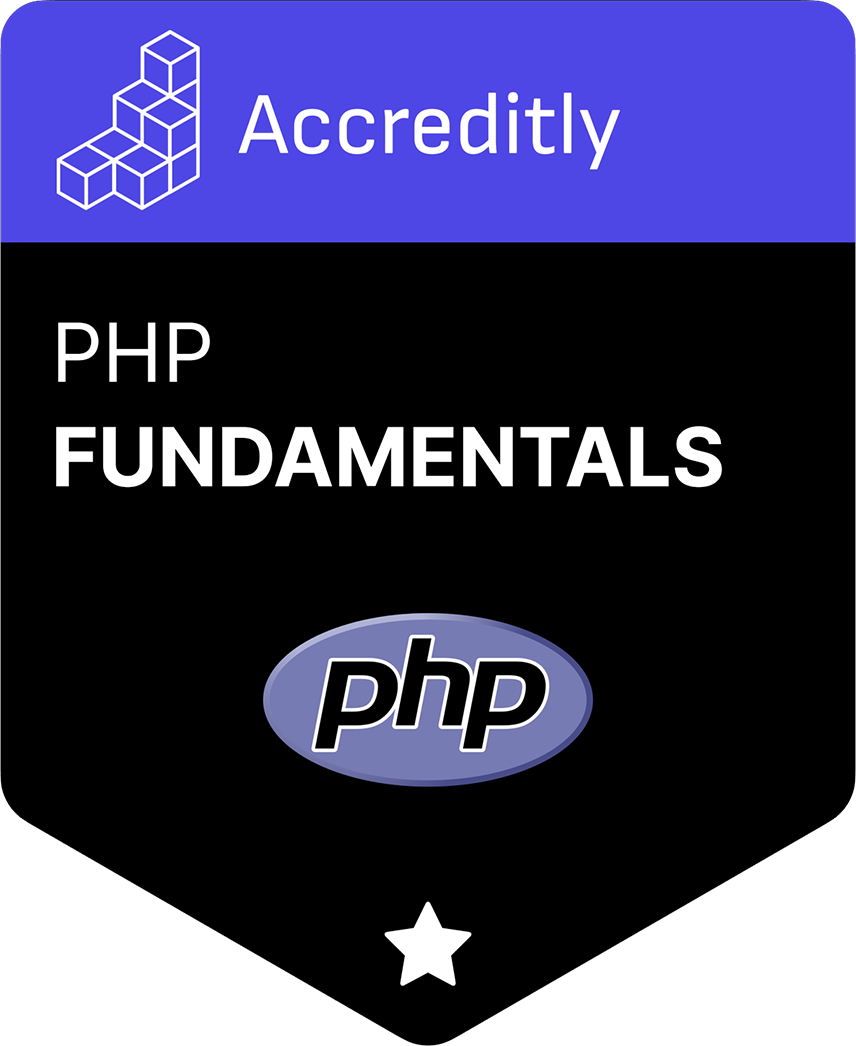