- Understanding the Risks
- The Encryption Strategy
- Step 1: Generating Encryption Keys
- Step 2: Encrypting .env Values
- Step 3: Decrypting .env Values in Your Application
- Step 4: Securing the Encryption Key
- Considerations and Best Practices
- Laravel
- Conclusion
The .env
file plays a pivotal role in configuring your PHP application's environment-specific variables. It's a central hub for sensitive information, such as database credentials, API keys, and other secrets critical to your application's functionality and security. However, storing sensitive information in plain text, even within an .env
file, poses significant security risks. Encryption is a vital step in mitigating these risks, ensuring that even if unauthorized access occurs, your sensitive data remains protected. This article explores practical methods to encrypt .env
values in a PHP environment.
Understanding the Risks
Before delving into the encryption process, it's crucial to understand the risks associated with storing sensitive information in plain text. Exposure of sensitive data can lead to security breaches, data theft, and potentially catastrophic consequences for your application and its users.
The Encryption Strategy
Encrypting your .env
values involves two main steps: encrypting the sensitive values before they enter the .env
file and decrypting them within your application when needed. PHP, with its robust set of cryptographic functions, facilitates this process through libraries like OpenSSL and Sodium.
Step 1: Generating Encryption Keys
First, you need a strong encryption key. You can generate a secure key using PHP's openssl_random_pseudo_bytes
function or similar methods provided by PHP encryption libraries.
Example:
$key = bin2hex(openssl_random_pseudo_bytes(32));
echo "Encryption key: " . $key;
Store this key securely; it is crucial for both encrypting and decrypting your data. Do not store it in your .env
file or version control.
.env
Values
Step 2: Encrypting With your encryption key in hand, you can now encrypt the sensitive values before adding them to your .env
file. PHP's OpenSSL extension provides functions to encrypt and decrypt data securely.
Example Encryption Script:
// Your encryption key
$key = 'your-32-char-encryption-key-here';
// Sensitive data to encrypt
$dataToEncrypt = "your-sensitive-data";
// Encrypt the data
$encrypted = openssl_encrypt($dataToEncrypt, 'aes-256-cbc', $key, 0, $iv);
echo "Encrypted value: " . $encrypted;
After encrypting your sensitive data, place the encrypted values in your .env
file, not the plain text.
.env
Values in Your Application
Step 3: Decrypting To use the encrypted values within your PHP application, decrypt them at runtime using the same key.
Example Decryption Script:
// Fetch your encrypted .env value
$encryptedEnvValue = getenv('ENCRYPTED_ENV_VARIABLE');
// Your encryption key (same as used for encrypting)
$key = 'your-32-char-encryption-key-here';
// Decrypt the data
$decrypted = openssl_decrypt($encryptedEnvValue, 'aes-256-cbc', $key, 0, $iv);
echo "Decrypted value: " . $decrypted;
Step 4: Securing the Encryption Key
Securing your encryption key is as crucial as encrypting the data itself. Ideally, the key should be stored outside of your application's codebase and .env
file—consider using a secure secrets management service or at least a separate, restricted configuration file on your server, not accessible from version control or by unauthorized users.
Considerations and Best Practices
- Never commit your
.env
file or encryption keys to version control. Use.env.example
to share the structure without revealing sensitive data. - Ensure strict access controls for any system or service storing your encryption keys.
- Regularly rotate your encryption keys to mitigate long-term exposure risks. Remember, you'll need to re-encrypt your
.env
values with the new key. - Implement a secure backup and recovery plan for your encryption keys. Losing the key could result in irreversible data loss.
Laravel
Laravel, from version 9.32 onwards, has an encryption method for .env
files built in. In Laravel it's as simple as:
php artisan env:encrypt
This will encrypt the .env
as .env.encrypted
, and provide a key. You can then decrypt it with:
php artisan env:decrypt --key=your-key-here
Conclusion
Encrypting your .env
file values in PHP adds a critical layer of security to your application, protecting sensitive data from unauthorized access. While this process involves several steps—from generating an encryption key to encrypting and decrypting values—it significantly enhances the overall security posture of your application. Remember, security is an ongoing process, requiring vigilance, regular updates, and adherence to best practices to safeguard your application and user data effectively.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
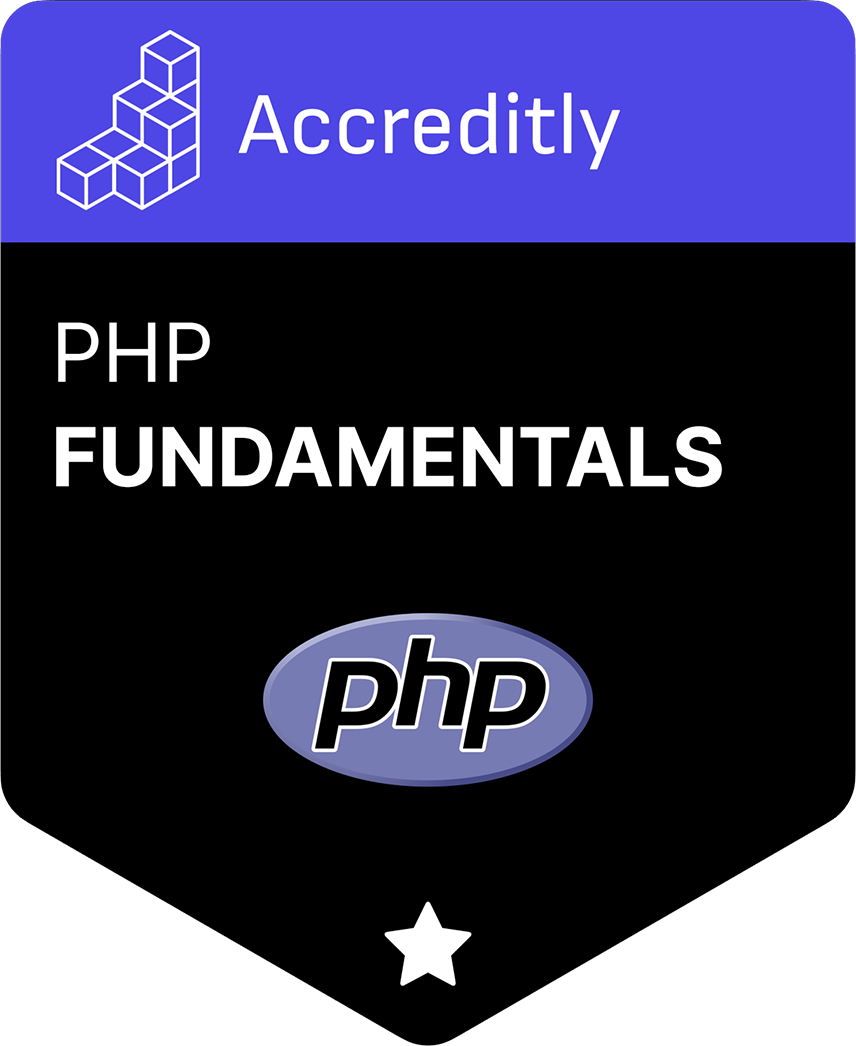