- Understanding Dashboard Widgets
- Setting Up Your Plugin
- Writing the Widget Code
- Adding Styles and Scripts
- Activating Your Plugin
- Best Practices
- Expanding Your Widget
WordPress, with its extensible nature, allows for adding custom widgets to the admin dashboard, providing quick access to important information or functionalities. Creating a dashboard widget plugin is an excellent way to introduce custom features or data to your WordPress backend. This article will guide you through the process of creating a basic dashboard widget plugin for WordPress.
Understanding Dashboard Widgets
Dashboard widgets in WordPress appear on the admin dashboard and can range from simple shortcuts and feeds to complex data representations. By creating a custom widget, you can tailor the admin experience to better suit your or your client's needs.
Setting Up Your Plugin
1. Create a Plugin Folder: In your WordPress installation, navigate to wp-content/plugins
and create a new folder for your plugin, like my-dashboard-widget
.
2. Create the Main Plugin File: Inside this folder, create a PHP file with the same name, my-dashboard-widget.php
.
3. Add the Plugin Header: Open the file and add the following plugin header:
```php
<?php
/*
Plugin Name: My Dashboard Widget
Description: Adds a custom widget to the WordPress admin dashboard.
Version: 1.0
Author: Your Name
*/
```
Writing the Widget Code
1. Hook into the Dashboard Setup: Use the wp_dashboard_setup
action hook to add your custom widget.
```php
add_action('wp_dashboard_setup', 'my_custom_dashboard_widget');
```
2. Define the Widget Function: Create the function that defines your widget. This function will register the widget with WordPress.
```php
function my_custom_dashboard_widget() {
wp_add_dashboard_widget(
'my_dashboard_widget', // Widget slug.
'My Custom Dashboard Widget', // Title.
'my_dashboard_widget_display' // Display callback.
);
}
```
3. Create the Display Callback Function: This function will output the content of your widget.
```php
function my_dashboard_widget_display() {
echo "
Welcome to My Custom Dashboard Widget!
";// Add more custom widget content here
}
```
Adding Styles and Scripts
If your widget requires specific styles or scripts, enqueue them using the admin_enqueue_scripts
action hook.
function my_dashboard_widget_scripts() {
// Enqueue styles and scripts here
}
add_action('admin_enqueue_scripts', 'my_dashboard_widget_scripts');
Activating Your Plugin
1. Navigate to the WordPress admin area.
2. Go to "Plugins".
3. Find "My Dashboard Widget" and click "Activate".
Best Practices
-
Keep it Lightweight: Dashboard widgets should be lightweight and load quickly.
-
User Experience: Consider the user experience -- make sure your widget adds value and isn't intrusive.
-
Security: Sanitize and validate any data input or output to ensure security, especially if your widget interacts with the database.
-
Testing: Test your widget across different WordPress setups and versions for compatibility.
Expanding Your Widget
As you become more comfortable with widget development, consider adding more features:
-
Ajax Calls: Implement Ajax to update widget content dynamically.
-
User Preferences: Allow users to customize the widget according to their preferences.
-
Integrate with APIs: Fetch data from external APIs to display in your widget.
Creating a custom dashboard widget plugin in WordPress is a fantastic way to enhance the functionality of your website's admin area. Whether it's for displaying custom analytics, shortcuts to frequently used functions, or integrating API data, a well-designed dashboard widget can significantly improve the efficiency and effectiveness of website management.
Also remember that these widgets will be one of the most access parts of the admin area, so if you are accessing APIs or external data that could be slow then ensure you're caching data appropriately to not slow the admin area loading down for all users.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
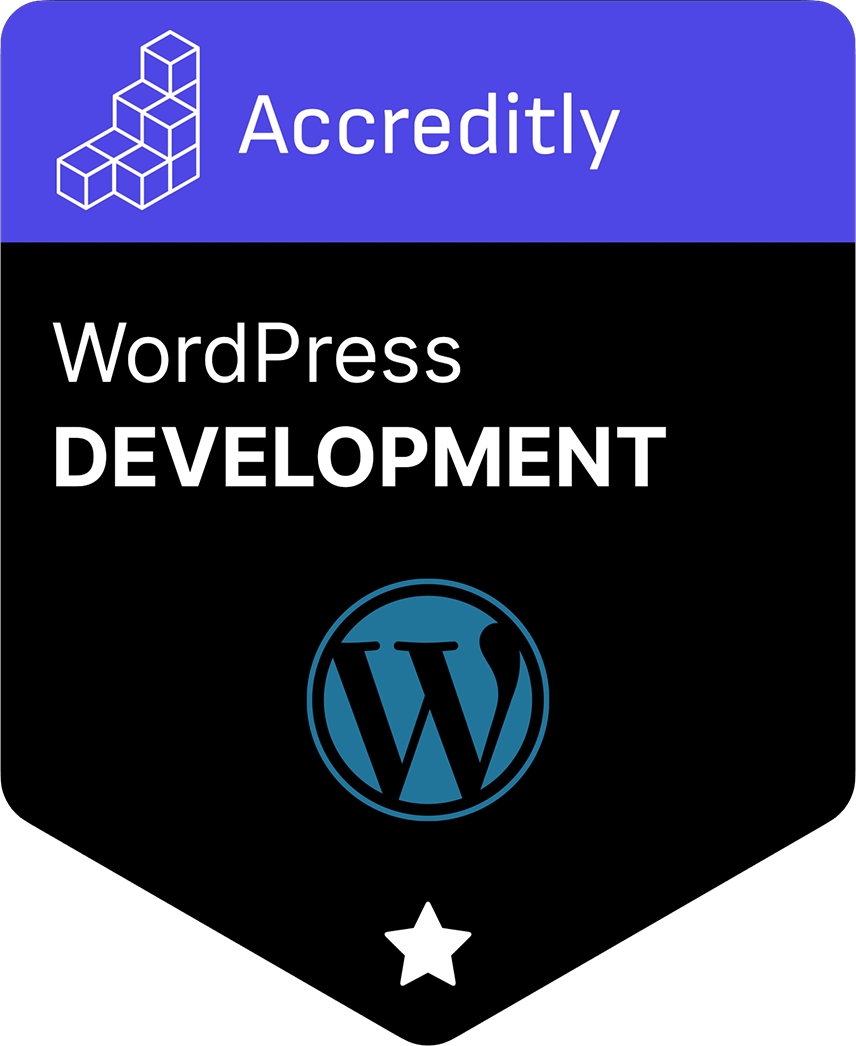