- Introduction to Blade Components
- Creating Blade Components
- Using Blade Components
- Props in Blade Components
- Slots in Blade Components
- Managing Blade Components with Classes
- Nested Blade Components
- Dynamic Components
- Anonymous Components
- Best Practices for Blade Components
Blade is Laravel’s powerful, simple, and flexible templating engine. Blade components take the flexibility of Blade templates to the next level by enabling you to create reusable and modular HTML blocks. This article will provide an in-depth look at Blade components, covering everything from creation to usage, including props, slots, and associated classes.
Introduction to Blade Components
Blade components are reusable pieces of a Blade template that allow you to avoid repetitive code and make your views more manageable. Components can be anything from a simple button to a complex layout with multiple slots and dynamic content.
Blade components bring several advantages:
- Reusability: Components can be reused throughout your application.
- Modularity: Breaking down views into components makes them more manageable.
- Maintainability: Changes in the component reflect wherever it's used, making maintenance easier.
- Separation of Concerns: Components can handle their own logic, making your views cleaner.
Creating Blade Components
Laravel makes it easy to create Blade components using the Artisan command-line tool.
-
Generate a Component: To create a new component, use the
make:component
Artisan command.php artisan make:component Alert
This command creates two files:
- A Blade view file:
resources/views/components/alert.blade.php
- A component class:
app/View/Components/Alert.php
- A Blade view file:
-
Blade View File: The view file contains the HTML structure of your component. Open
resources/views/components/alert.blade.php
and add the following content:<div class="alert alert-{{ $type }}"> {{ $message }} </div>
-
Component Class: The class handles the logic for your component. Open
app/View/Components/Alert.php
and update it as follows:namespace App\View\Components; use Illuminate\View\Component; class Alert extends Component { public $type; public $message; public function __construct($type, $message) { $this->type = $type; $this->message = $message; } public function render() { return view('components.alert'); } }
Using Blade Components
To use a Blade component in your view, use the <x-
syntax followed by the component name. For example, to use the Alert
component, you would write:
<x-alert type="success" message="Operation successful!" />
This will render the alert
component with the specified type
and message
.
Props in Blade Components
Props are the way you pass data to Blade components. They work similarly to HTML attributes. In the Alert
component above, type
and message
are props.
-
Defining Props: Props are defined in the component class constructor.
public $type; public $message; public function __construct($type, $message) { $this->type = $type; $this->message = $message; }
-
Passing Props: When using the component, pass props like HTML attributes.
<x-alert type="danger" message="Something went wrong!" />
-
Default Values for Props: You can set default values for props in the component class.
public function __construct($type = 'info', $message = 'Default message') { $this->type = $type; $this->message = $message; }
-
Accessing Props in Views: Props are accessible within the component's Blade view using standard Blade syntax.
<div class="alert alert-{{ $type }}"> {{ $message }} </div>
Slots in Blade Components
Slots allow you to define placeholder sections in your component that can be filled with content when the component is used.
-
Default Slot: The default slot is the simplest form, representing the content passed between the component tags.
<!-- resources/views/components/alert.blade.php --> <div class="alert alert-{{ $type }}"> {{ $slot }} </div>
-
Using Default Slot: Pass content to the default slot by placing it between the component tags.
<x-alert type="success"> Operation was successful! </x-alert>
-
Named Slots: Named slots allow you to define multiple placeholders within your component.
<!-- resources/views/components/card.blade.php --> <div class="card"> <div class="card-header"> {{ $header }} </div> <div class="card-body"> {{ $slot }} </div> <div class="card-footer"> {{ $footer }} </div> </div>
-
Using Named Slots: Pass content to named slots using the
x-slot
directive.<x-card> <x-slot name="header"> Card Header </x-slot> This is the main content. <x-slot name="footer"> Card Footer </x-slot> </x-card>
Managing Blade Components with Classes
Using classes with Blade components allows you to encapsulate logic and handle more complex scenarios.
-
Component Classes: Define a class for your component using the
make:component
command. The class will handle the logic.php artisan make:component Card
-
Class Structure: The component class typically includes a constructor to handle props and a
render
method to return the view.namespace App\View\Components; use Illuminate\View\Component; class Card extends Component { public $title; public function __construct($title) { $this->title = $title; } public function render() { return view('components.card'); } }
-
Using the Component: Use the component in your views, passing props as needed.
<x-card title="My Card Title"> This is the card content. </x-card>
-
Blade View: Access the prop within the Blade view.
<!-- resources/views/components/card.blade.php --> <div class="card"> <div class="card-header"> {{ $title }} </div> <div class="card-body"> {{ $slot }} </div> </div>
Nested Blade Components
Blade components can be nested to create complex UI structures.
-
Define Parent and Child Components: Create the parent and child components using the
make:component
command.php artisan make:component ParentComponent php artisan make:component ChildComponent
-
Parent Component View: Use the child component within the parent component's view.
<!-- resources/views/components/parent-component.blade.php --> <div class="parent"> <h1>Parent Component</h1> <x-child-component /> </div>
-
Child Component View: Define the structure for the child component.
<!-- resources/views/components/child-component.blade.php --> <div class="child"> <p>This is the child component.</p> </div>
-
Using the Nested Components: Use the parent component in your main view.
<x-parent-component />
Dynamic Components
Dynamic components allow you to render components based on runtime values.
-
Dynamic Component Example: Use the
component
directive to dynamically render a component.@php $componentName = 'alert'; $componentData = ['type' => 'info', 'message' => 'This is an info alert.']; @endphp <x-dynamic-component :component="$componentName" :type="$componentData['type']" :message="$componentData['message']" />
Anonymous Components
Anonymous components are simpler and do not require a class file. They are useful for small, reusable components.
-
Creating Anonymous Components: Create an anonymous component by placing a Blade file in the
resources/views/components
directory.<!-- resources/views/components/alert.blade.php --> <div class="alert alert-{{ $type }}"> {{ $slot }} </div>
-
Using Anonymous Components: Use the component in your views.
<x-alert type="warning"> This is a warning alert. </x-alert>
Best Practices for Blade Components
-
Component Naming: Use descriptive names for your components to make your code more readable.
-
Reusable Components: Create components for UI elements that are reused across your application to maintain consistency.
-
Avoid Logic in Views: Keep your Blade views clean by moving logic to the component class or a dedicated service class.
-
Documentation: Document your components and their props to make them easier to use and maintain.
-
Test Components: Write tests for your components to ensure they render correctly with different prop values and slot content.
Blade components are a powerful feature in Laravel that help you create reusable, maintainable, and modular HTML blocks. They make your views cleaner and your application easier to manage. In this article, we covered everything you need to know about Blade components, including how to create them, use props and slots, manage components with classes, and follow best practices.
By mastering Blade components, you can significantly improve the structure and maintainability of your Laravel applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
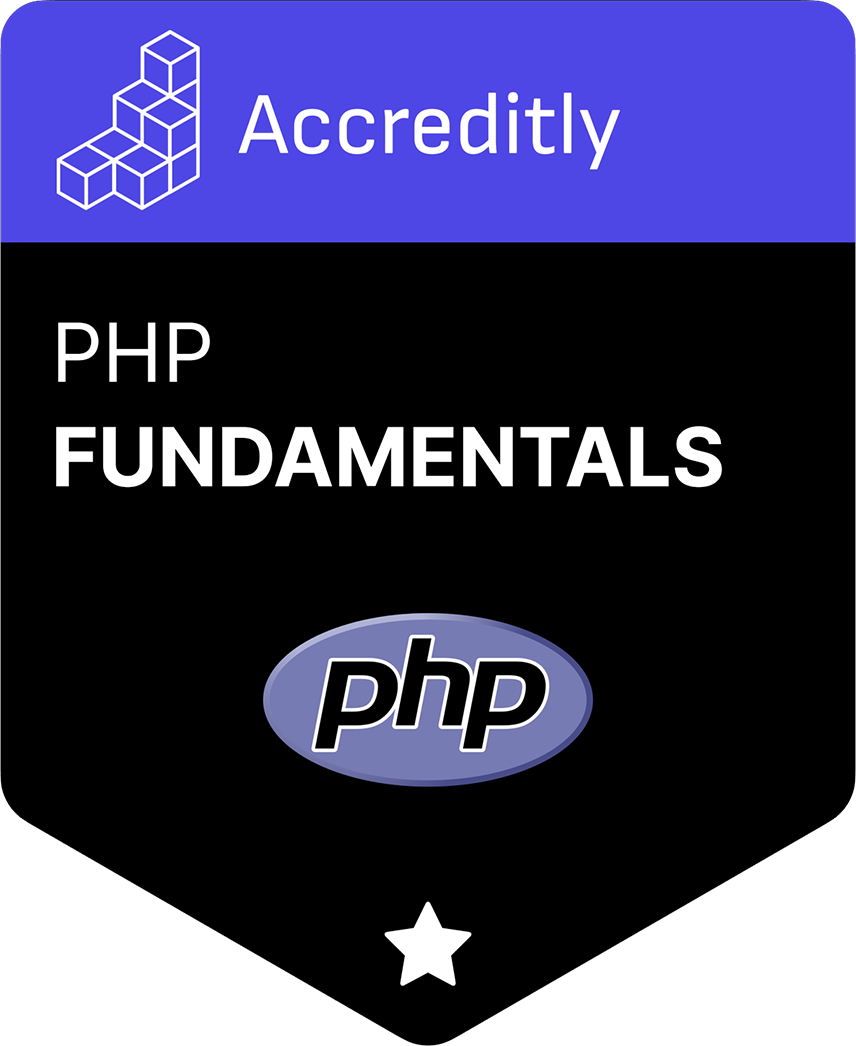