- Understanding Laravel Packages
- Why Avoid Forking?
- Methods to Extend a Laravel Package
- Practical Example: Extending a Real Package
- Takeaways
Extending a Laravel package without forking it is a common requirement for developers who want to add or modify functionality without maintaining a separate codebase. Laravel's flexibility and rich ecosystem make it easy to customize packages. This article explores various methods to extend a package in Laravel without forking, ensuring your modifications remain manageable and maintainable.
Understanding Laravel Packages
Laravel packages are essentially pieces of reusable code that can be included in Laravel applications to provide specific functionality. They range from simple helper libraries to complex frameworks. Some popular packages include Laravel Debugbar for debugging and Spatie's Laravel Permissions for handling roles and permissions.
Why Avoid Forking?
Forking a package means creating your own copy of the package repository, which you then modify. While this gives you complete control, it also means you must manually merge updates from the original package. This can be tedious and error-prone. Instead, Laravel offers several ways to extend packages without forking, allowing you to keep your customizations separate from the original package code.
Methods to Extend a Laravel Package
- Service Providers
- Publishing Configuration Files
- Overriding Views
- Using Package Events
- Creating Wrapper Classes
1. Service Providers
Service providers are the central place to configure and extend a Laravel package. They are responsible for binding classes into the service container and registering event listeners, routes, and more.
Example: Extending a Package with a Custom Service Provider
Suppose you are using a package that provides a service class you want to extend. First, create your custom service provider:
php artisan make:provider CustomServiceProvider
In your CustomServiceProvider
, override the service binding:
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use PackageNamespace\OriginalService;
class CustomServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->singleton(OriginalService::class, function ($app) {
return new CustomService();
});
}
}
Then, register your custom service provider in config/app.php
:
'providers' => [
// Other Service Providers
App\Providers\CustomServiceProvider::class,
],
2. Publishing Configuration Files
Many packages come with configuration files that you can publish and modify to suit your needs. This method is straightforward and does not require modifying the package code directly.
Example: Publishing Configuration Files
To publish a package's configuration file, run:
php artisan vendor:publish --provider="PackageNamespace\PackageServiceProvider" --tag="config"
This command will copy the package’s configuration file to the config
directory. You can then modify this file as needed.
3. Overriding Views
If a package includes views that you need to customize, you can override them without modifying the package directly.
Example: Overriding Package Views
First, publish the package’s views:
php artisan vendor:publish --provider="PackageNamespace\PackageServiceProvider" --tag="views"
This will copy the package’s views to the resources/views/vendor/package
directory. You can then edit these views as required.
4. Using Package Events
Packages often dispatch events that you can listen to and respond to in your application. This is a powerful way to extend functionality without altering the package code.
Example: Listening to Package Events
First, define an event listener in your EventServiceProvider
:
protected $listen = [
'PackageNamespace\Events\SomeEvent' => [
'App\Listeners\HandleSomeEvent',
],
];
Then, create the event listener:
namespace App\Listeners;
use PackageNamespace\Events\SomeEvent;
class HandleSomeEvent
{
public function handle(SomeEvent $event)
{
// Custom logic here
}
}
5. Creating Wrapper Classes
Sometimes, you may need to extend or modify the behavior of a class provided by a package. You can achieve this by creating a wrapper class that extends the package’s class.
Example: Creating a Wrapper Class
Suppose you want to add a method to a package’s service class:
namespace App\Services;
use PackageNamespace\Services\OriginalService;
class CustomService extends OriginalService
{
public function newMethod()
{
// Custom functionality here
}
}
Then, bind your custom class in a service provider as shown in the service provider example above.
Practical Example: Extending a Real Package
Let's extend the functionality of the Spatie Laravel Permission package by adding a custom method to the Role
model.
Step 1: Create a Custom Model
namespace App\Models;
use Spatie\Permission\Models\Role as SpatieRole;
class Role extends SpatieRole
{
public function hasUser($userId)
{
return $this->users()->where('id', $userId)->exists();
}
}
Step 2: Bind the Custom Model in a Service Provider
Create a custom service provider if you haven't already:
php artisan make:provider CustomServiceProvider
In your CustomServiceProvider
, override the model binding:
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use Spatie\Permission\Models\Role as SpatieRole;
use App\Models\Role;
class CustomServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->bind(SpatieRole::class, Role::class);
}
}
Register your service provider in config/app.php
:
'providers' => [
// Other Service Providers
App\Providers\CustomServiceProvider::class,
],
Now, when you use the Role
model provided by Spatie’s package, your custom model will be used instead.
Takeaways
Extending a Laravel package without forking it allows you to keep your codebase maintainable and up-to-date with the latest package versions. By using service providers, publishing configuration files, overriding views, listening to events, and creating wrapper classes, you can tailor packages to your needs while avoiding the pitfalls of maintaining a fork.
For more detailed information, refer to the Laravel Documentation on Service Providers and the Composer Documentation. By mastering these techniques, you can efficiently extend Laravel packages and maintain a clean, manageable codebase.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
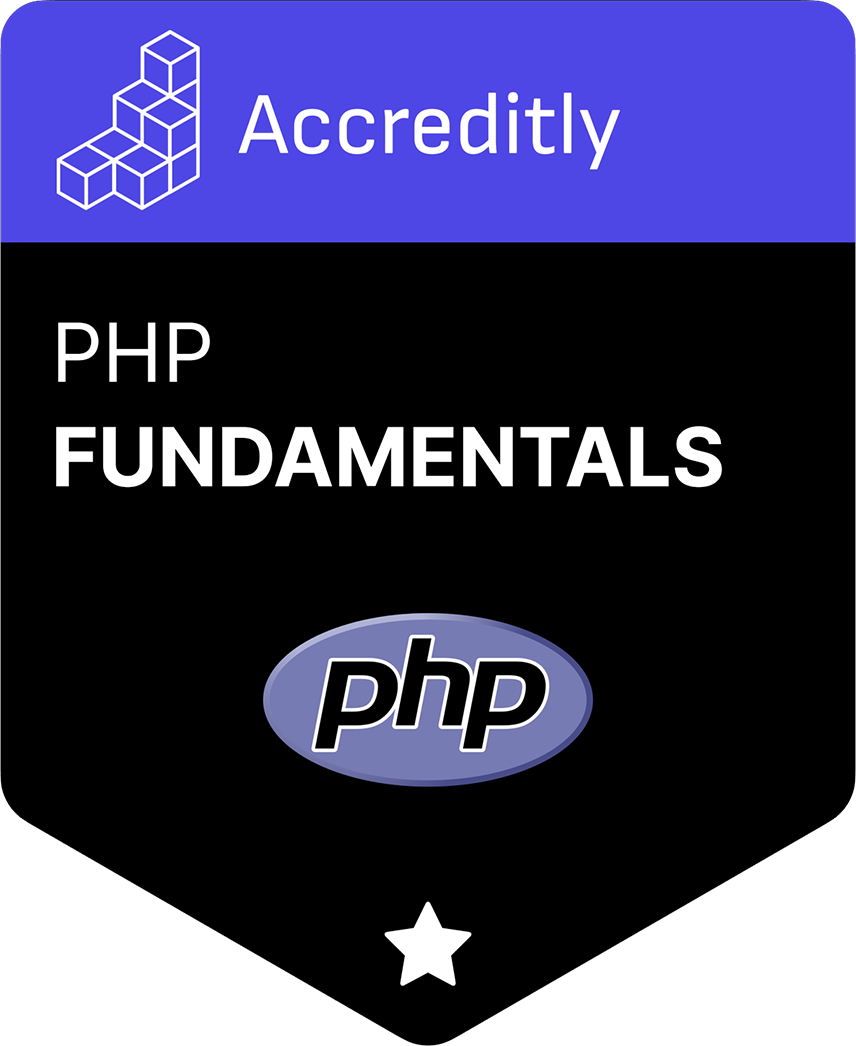