- Understanding Chunked File Uploads
- Prerequisites
- Step 1: Setting Up the Client-Side Code
- Step 2: Receiving Chunks on the Server
- Step 3: Merging File Chunks
- Step 4: Security and Validation
- Conclusion
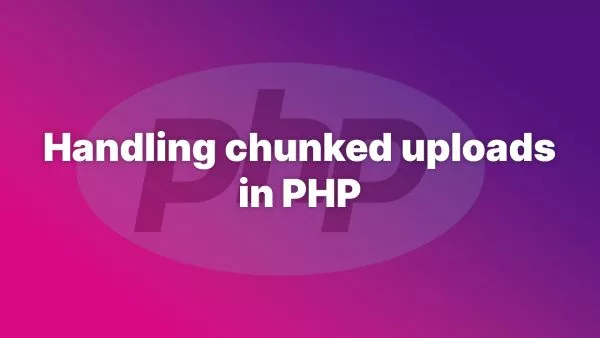
Handling large file uploads is a common challenge in web development, often leading to timeouts or failed uploads due to server limitations or unstable network conditions. Chunked file uploads provide a robust solution by breaking down a large file into smaller, manageable pieces (chunks) and uploading them sequentially. This method not only improves the reliability of file uploads but also allows for pause, resume, and retry functionalities. This article guides you through implementing chunked file uploads on the server-side using PHP.
Understanding Chunked File Uploads
Chunked file uploads work by dividing a file into multiple chunks on the client-side, then sequentially uploading those chunks to the server. Once all chunks have been uploaded, they are reassembled into the original file. This process requires coordinated effort between the client-side code (JavaScript) and the server-side logic (PHP).
Prerequisites
- Basic knowledge of PHP and JavaScript
- Access to a server with PHP installed
- Familiarity with client-side file upload mechanisms
Step 1: Setting Up the Client-Side Code
Before handling the uploads on the server, you need to prepare the client-side code to split files into chunks and send them. While this article focuses on the PHP side, understanding the client-side logic is crucial. You might use JavaScript's Blob.slice()
method to divide the file and FormData
to send chunks via AJAX.
We have a comprehensive tutorial on chunking files for chunked uploads.
Step 2: Receiving Chunks on the Server
Each chunk sent by the client needs to be received and temporarily stored by the server until all parts of the file are uploaded. Here's a simple PHP script to handle an incoming chunk:
<?php
$targetDir = "uploads/chunks"; // Directory to store file chunks
$targetFile = $targetDir . '/' . basename($_FILES["file"]["name"]);
// Make sure the directory exists
if (!file_exists($targetDir)) {
mkdir($targetDir, 0777, true);
}
// Move the uploaded chunk to the target directory
if (move_uploaded_file($_FILES["file"]["tmp_name"], $targetFile)) {
echo "The file " . htmlspecialchars(basename($_FILES["file"]["name"])) . " has been uploaded.";
} else {
echo "Sorry, there was an error uploading your file.";
}
This script assumes each chunk comes in as a separate request. It moves the received chunk to a specified directory. You should enhance this basic example with mechanisms to identify different chunks of the same file, typically handled via additional POST data (e.g., file identifier, chunk index).
Step 3: Merging File Chunks
After all chunks are uploaded, the next step is to merge them back into the original file. This can be triggered by a separate request indicating the completion of the upload process or automatically detected by checking if all expected chunks have been received.
Here's how you can merge chunks in PHP:
<?php
$targetDir = "uploads/chunks";
$finalFile = "uploads/final/" . $_POST['fileName']; // Final file path and name
// Open the final file handle
$fp = fopen($finalFile, 'wb');
// Assuming chunks are named sequentially (file.part1, file.part2, ...)
for ($i=0; $i < $_POST['totalChunks']; $i++) {
$chunkFile = $targetDir . '/' . $_POST['fileName'] . ".part" . $i;
// Read and write the current chunk
$chunk = file_get_contents($chunkFile);
fwrite($fp, $chunk);
// Optionally, delete chunk file after appending
unlink($chunkFile);
}
// Close the final file handle
fclose($fp);
echo "Upload and merge complete.";
This script collects all chunks, ordered sequentially, and writes their contents into the final file. Adjust the naming conventions as needed based on your client-side implementation.
Step 4: Security and Validation
Handling file uploads requires careful consideration of security and data validation:
- Validate file types both on the client and server sides to prevent malicious uploads.
- Implement error handling for interrupted uploads, including cleanup of partial uploads.
- Secure the temporary storage directory, either through
.htaccess
restrictions or by placing it outside the web root.
Conclusion
Implementing chunked file uploads in PHP enhances your application's ability to handle large files, improving usability and reliability. This guide outlines the server-side logic necessary to receive, store, and reassemble file chunks. Remember, the key to a successful chunked upload implementation lies in the seamless integration between client-side mechanisms and server-side processing, coupled with robust security and error handling practices. With these foundations, your application can efficiently manage large file uploads, providing a smooth experience
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
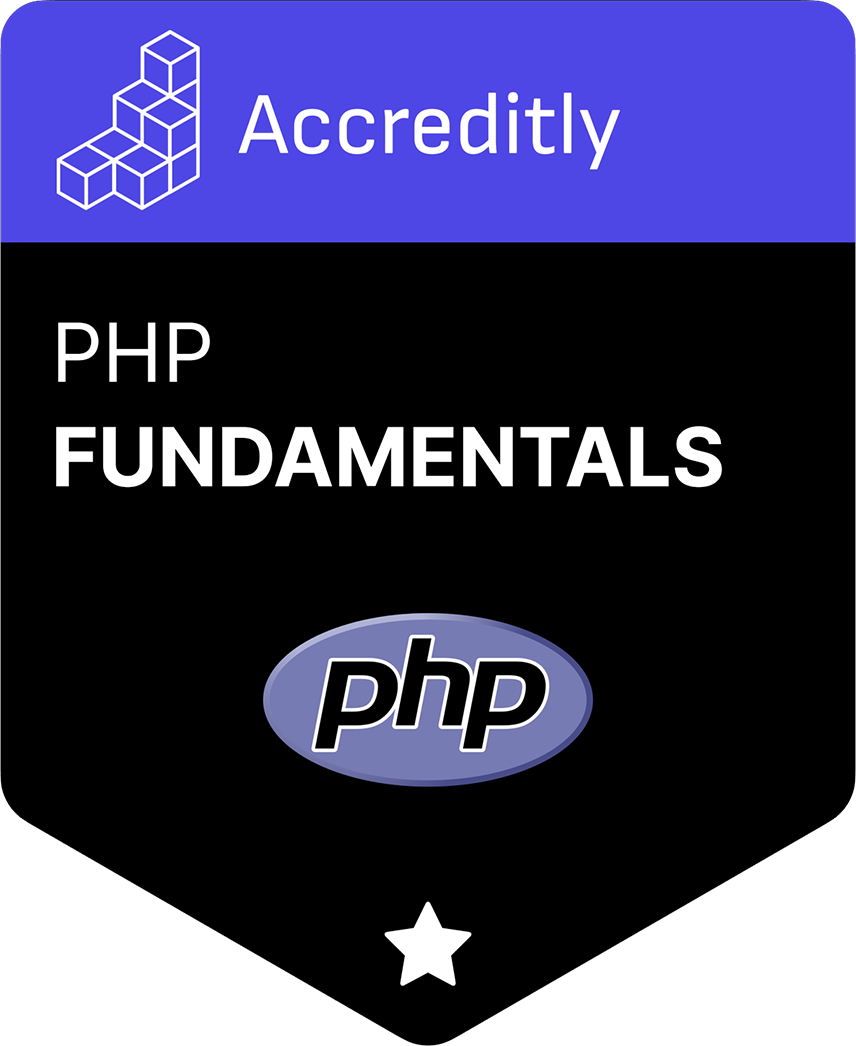
Related articles
Tutorials WordPress PHP Tooling
Optimizing WordPress YouTube Embeds with Lite YouTube Embed
Learn how to enhance your WordPress site's performance by replacing the native YouTube embed with Lite YouTube Embed. This guide takes you through the steps to override the default oEmbed behaviour for YouTube videos.