Cloning objects can be useful for a few reasons:
-
You want to make changes to an object but not affect the original
-
You need multiple instances of a complex object with the same basic state
-
You want to duplicate something that took time and resources to initialize
Instead of creating a new object from scratch and manually setting all its properties, cloning saves time and effort.
PHP Clone Syntax
PHP has a built-in way to clone objects - the clone
keyword. Here's the syntax:
$copy = clone $original;
This makes $copy
a full duplicate of $original
. Any changes made to $copy
will not affect $original
.
You can also call the __clone()
magic method manually:
$copy = $original->__clone();
This does the same thing as using the clone
keyword.
Cloning Example
Here's a full object cloning example:
// Original object
class Book {
public $title;
public $numPages;
public function __construct($title, $pages) {
$this->title = $title;
$this->numPages = $pages;
}
}
// Create an instance
$book1 = new Book("A Tale of Two Cities", 384);
// Clone it
$book2 = clone $book1;
// Change the clone's title
$book2->title = "Oliver Twist";
// Original book is unchanged
echo $book1->title; // A Tale of Two Cities
echo $book2->title; // Oliver Twist
As you can see, cloning allows you to duplicate an object and modify it without impacting the original instance.
When Not to Clone
Cloning objects does have some downsides to consider:
- Performance overhead - Cloning takes time and memory
- Complex object graphs - Child objects are shallow copied by default
Just keep these limitations in mind when deciding whether cloning is appropriate.
So in summary, the clone keyword in PHP provides an easy way to duplicate objects. Understanding object cloning helps unlock the full power of object-oriented code reuse.
There are some additional examples and helpful resources on PHP's object cloning page.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
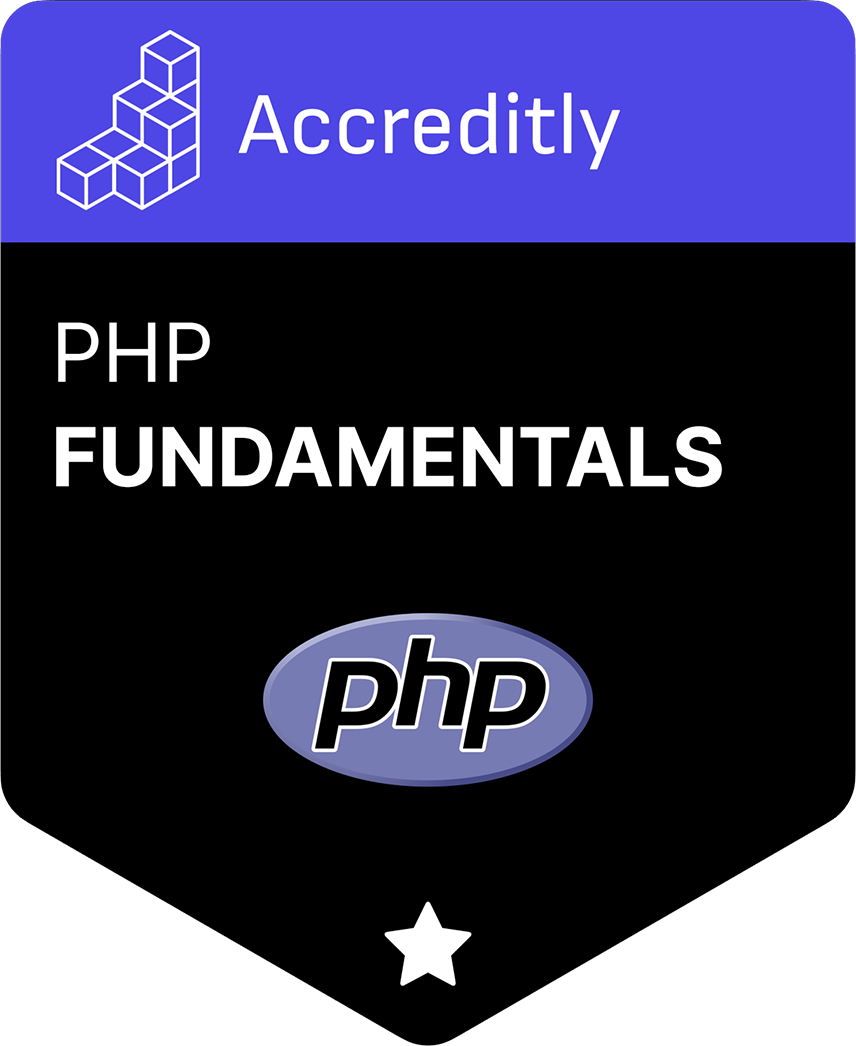