- Understanding HTTP Status Codes
- Method 1: Using Fetch API
- Method 2: Using XMLHttpRequest
- Method 3: Using the performance API
- Method 4: Server-Side Logging
- Conclusion
When developing web applications, it's often useful to know the HTTP status code of the current page. HTTP status codes are vital for understanding the result of an HTTP request, such as whether a page has loaded successfully, encountered an error, or requires authentication. In JavaScript, while there's no direct way to retrieve the HTTP status code of the current page directly from the browser, several workarounds can achieve this. This article will explore various methods to obtain the HTTP status code using JavaScript.
Understanding HTTP Status Codes
Before diving into the methods, let's briefly review HTTP status codes:
- 1xx Informational: Request received, continuing process.
- 2xx Success: The action was successfully received, understood, and accepted (e.g., 200 OK).
- 3xx Redirection: Further action must be taken to complete the request (e.g., 301 Moved Permanently, 302 Found).
- 4xx Client Error: The request contains bad syntax or cannot be fulfilled (e.g., 404 Not Found).
- 5xx Server Error: The server failed to fulfill an apparently valid request (e.g., 500 Internal Server Error).
Method 1: Using Fetch API
The Fetch API is a modern way to make network requests and handle responses, including retrieving status codes. However, it's important to note that this method works for making new requests rather than obtaining the status code of the current page directly. Here's how you can use the Fetch API to get the HTTP status code of a request:
fetch(window.location.href)
.then(response => {
console.log(`HTTP Status Code: ${response.status}`);
})
.catch(error => {
console.error('Error fetching the page:', error);
});
This code fetches the current page URL and logs the HTTP status code.
Method 2: Using XMLHttpRequest
XMLHttpRequest is an older way to make network requests in JavaScript. Similar to the Fetch API, it doesn't provide a direct way to get the status code of the current page but can be used to make a new request and obtain the status code:
var xhr = new XMLHttpRequest();
xhr.open('GET', window.location.href, true);
xhr.onload = function() {
console.log(`HTTP Status Code: ${xhr.status}`);
};
xhr.onerror = function() {
console.error('Error fetching the page');
};
xhr.send();
This script creates an XMLHttpRequest
to fetch the current page and logs the HTTP status code.
performance
API
Method 3: Using the The performance
API provides various metrics about the loading of the current page, including resource timing. By accessing the performance entries, you can find detailed information about the network request, including the HTTP status code. However, this method might not be reliable across all browsers and scenarios:
function getStatusCode() {
const entries = performance.getEntriesByType('navigation');
if (entries.length > 0) {
const entry = entries[0];
console.log(`HTTP Status Code: ${entry.responseStatus}`);
} else {
console.log('No navigation entry found.');
}
}
window.onload = getStatusCode;
This function retrieves the navigation performance entries and logs the HTTP status code of the current page. Note that responseStatus
might not be available in all browsers.
Method 4: Server-Side Logging
If client-side methods are insufficient, a server-side approach can be more robust. By logging the HTTP status code on the server and sending it to the client via an endpoint or embedded script, you can reliably obtain the status code. Here's an example using Node.js and Express:
// Server-side (Node.js with Express)
const express = require('express');
const app = express();
app.get('/', (req, res) => {
// Simulate different status codes for demonstration
const statusCode = 200; // You can change this to any valid status code
res.status(statusCode).send(`Status Code: ${statusCode}`);
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
<!-- Client-side (HTML with embedded script) -->
<!DOCTYPE html>
<html>
<head>
<title>Get HTTP Status Code</title>
</head>
<body>
<script>
fetch('/status')
.then(response => response.text())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error fetching status:', error);
});
</script>
</body>
</html>
In this setup, the server sends the status code as part of the response, which the client can then fetch and log.
Conclusion
While JavaScript doesn't provide a built-in way to directly access the HTTP status code of the current page, several workarounds can achieve this goal. Whether using the Fetch API, XMLHttpRequest, performance API, or server-side logging, you can obtain and utilize HTTP status codes to enhance your web application's functionality and user experience.
Understanding and handling HTTP status codes is crucial for robust web development, ensuring that your application can gracefully handle different scenarios and provide meaningful feedback to users.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
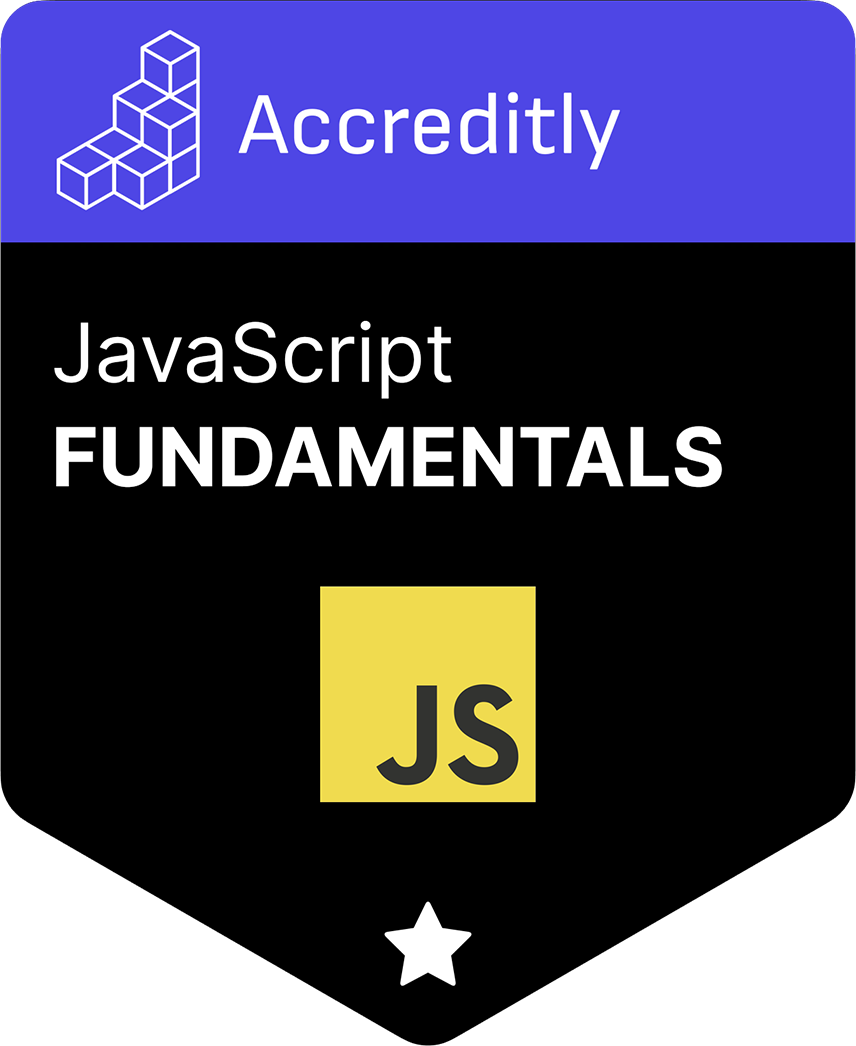