- 1. Creating a Custom Post Type
- 2. Defining the URL structure
- 3. Creating a Listing Page
- 4. Creating Detail Pages
WordPress, as a versatile CMS, provides developers with tools to create custom URLs and pages, facilitating advanced data manipulation. This article will guide you on how to create a database of entities, route custom URLs, and create listing and detail pages for each item in the database using an ID in the URL.
1. Creating a Custom Post Type
First, you need to register a custom post type. WordPress provides the register_post_type()
function for this purpose. The following code snippet should be added in your theme’s functions.php
file (always remember to properly organise your theme code):
function create_custom_post_type() {
$args = array(
'public' => true,
'label' => 'Entities'
);
register_post_type('entity', $args);
}
add_action('init', 'create_custom_post_type');
This creates a new post type 'entity' which will be public and labelled as 'Entities'.
2. Defining the URL structure
You can define the URL structure for your entities using WordPress's rewrite API.
function create_custom_post_type() {
$args = array(
'public' => true,
'label' => 'Entities',
'rewrite' => array('slug' => 'entity'),
);
register_post_type('entity', $args);
}
add_action('init', 'create_custom_post_type');
With the rewrite parameter, the URL for each entity will now look like yourwebsite.com/entity/post_slug
.
3. Creating a Listing Page
A listing page, which is a page that lists all of your entities, can be created by making a custom template file in your theme folder. Let's call it archive-entity.php
.
Here is a basic example of what your archive-entity.php
might look like:
<?php
get_header();
$args = array(
'post_type' => 'entity',
'posts_per_page' => -1
);
$query = new WP_Query($args);
if($query->have_posts()) {
while($query->have_posts()) {
$query->the_post();
?>
<h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<?php
}
}
get_footer();
This code will display all entities with a link to their individual detail page.
4. Creating Detail Pages
For the detail pages, WordPress will automatically generate these based on your custom post type. It will use the single.php file in your theme to display the entity's post.
However, if you want a custom layout for your entity posts, you can create a single-entity.php
file in your theme folder. WordPress will automatically use this template to display the entity posts.
Here's an example of a single-entity.php
file:
<?php
get_header();
while(have_posts()) {
the_post();
?>
<h1><?php the_title(); ?></h1>
<p><?php the_content(); ?></p>
<?php
}
get_footer();
This code will display the title and content of the entity.
Remember, after making changes to the URL structure, always visit the Permalinks page in your WordPress admin to flush the rewrite rules and make your new URL structure work correctly.
In this article, we've learned how to create custom routes for our entities, and created listing and detail pages for each item in our database using WordPress. This allows for highly customizable and dynamic content, depending on the needs of your project.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
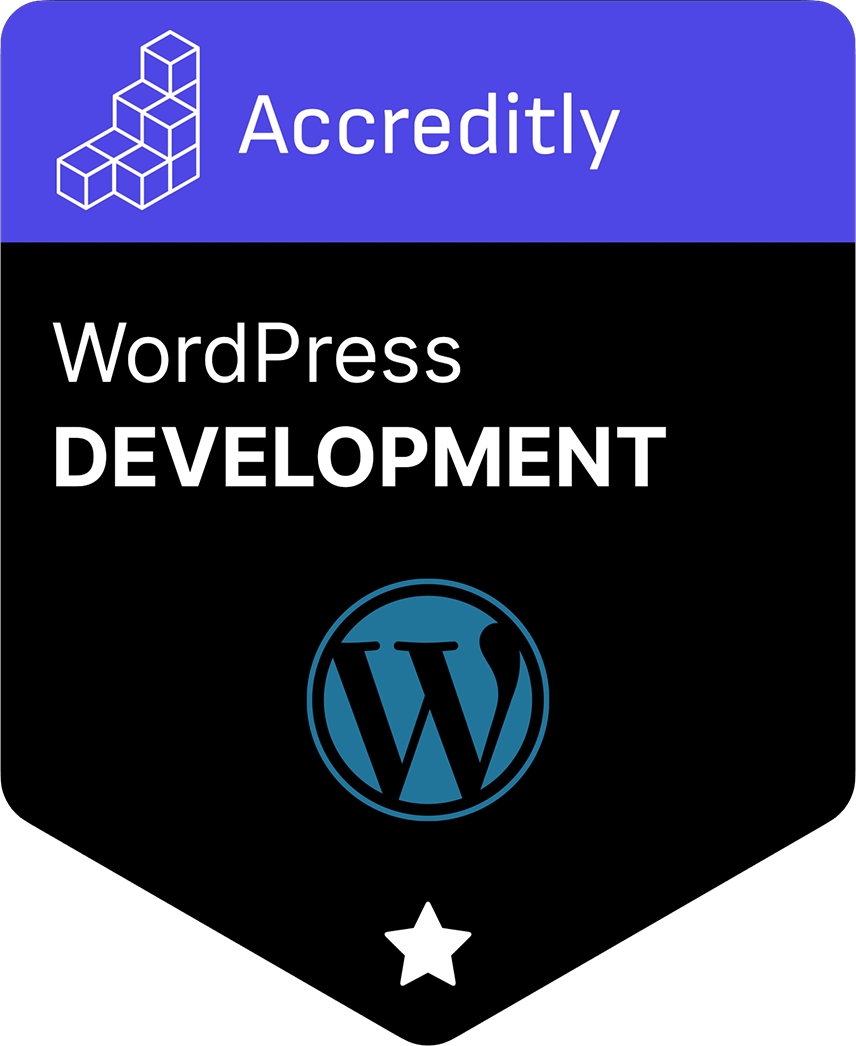