- 1. Creating a Breadcrumb Function
- 2. Handling the Home Link
- 3. Handling Built-in Post Types
- 4. Handling Custom Post Types
- 5. Handling Taxonomies
- 6. Displaying the Breadcrumbs
- 7. Styling the Breadcrumbs
Breadcrumbs are an essential part of any website's user experience. They provide a clear and efficient way for users to navigate your site and understand their current location within the site's hierarchy. Breadcrumbs are also an essential part of SEO, as they provide internal links to relevant parts of your website, and prevent orphaned pages.
In WordPress, you can dynamically generate and display breadcrumbs for built-in post types, custom post types, and taxonomies with just a few lines of code, without the need of a plugin that will undoubtedly add bloat. In this tutorial, we will guide you through the process of implementing breadcrumbs in your WordPress theme, including handling built-in post types, custom post types, and taxonomies with practical code examples.
Although building out breadcrumbs in WordPress is fairly straightforward there is no 'catch-all' method to catch everything. Instead, we must handle all of the possible links that could be part of a breadcrumb ourselves. While that might be more work, it does allow us an extra layer of customisation, should we need it.
Remember, if this all seems easy to you, then you're probably going to be interested in our WordPress certification. If none of this post seems difficult to implement then the certification is a good fit for you.
1. Creating a Breadcrumb Function
The first step in implementing breadcrumbs in WordPress is to create a function that will generate the breadcrumb markup. This function will be responsible for determining the current page's hierarchy and outputting the appropriate breadcrumb links. Let's start by creating a simple function called accreditly_breadcrumbs()
in your theme's functions.php
file. Remember to prefix your functions with your theme or plugin name to prevent namespacing issues. In our examples we use accreditly_
. Unsure why? Check out our post on why you should prefix functions in WordPress.
function accreditly_breadcrumbs() {
// Breadcrumb generation code will go here
}
2. Handling the Home Link
The first breadcrumb in any breadcrumb trail is typically the home link. We'll add this to our accreditly_breadcrumbs()
function:
function accreditly_breadcrumbs() {
$breadcrumb = '<nav class="breadcrumb">';
$breadcrumb .= '<a href="' . home_url() . '">Home</a>';
// Additional breadcrumb generation code will go here
$breadcrumb .= '</nav>';
echo $breadcrumb;
}
The home link is optional, and it depends on what your website does as to whether you want to include it or not. You may also want to add some logic to only display the home link if you're not on the home page, to prevent a link to 'Home' being placed on the screen when you're already there.
3. Handling Built-in Post Types
Now that we have the basic structure for our breadcrumb function, we can start adding support for different types of content. First, let's handle built-in post types like pages and blog posts:
function accreditly_breadcrumbs() {
// ... (Home link code)
if (is_single()) {
$categories = get_the_category();
$breadcrumb .= ' » <a href="' . get_category_link($categories[0]->term_id) . '">' . $categories[0]->name . '</a>';
$breadcrumb .= ' » ' . get_the_title();
} elseif (is_page()) {
$breadcrumb .= ' » ' . get_the_title();
}
// ... (Closing breadcrumb markup)
}
Here, we're using the is_single()
and is_page()
conditional tags to check if we're on a single blog post or a page, respectively. For single posts, we also display the post's category.
4. Handling Custom Post Types
To add support for custom post types, we'll need to modify our function to handle the is_singular()
conditional tag. This tag checks if we're on a single post, page, or any custom post type:
function accreditly_breadcrumbs() {
// ... (Previous code)
if (is_singular()) {
// Get the post type object
$post_type = get_post_type_object(get_post_type());
// Check if it's a custom post type
if ($post_type->name !== 'post' && $post_type->name !== 'page') {
$breadcrumb .= ' » <a href="' . get_post_type_archive_link($post_type->name) . '">' . $post_type->label . '</a>';
}
$breadcrumb .= ' » ' . get_the_title();
}
// ... (Closing breadcrumb markup)
}
Now, our breadcrumb function will display a link to the custom post type's archive page, followed by the current post's title.
5. Handling Taxonomies
To handle taxonomies, such as categories and tags, as well as custom taxonomies, we'll need to update our function to account for the is_tax()
, is_category()
, and is_tag()
conditional tags:
function accreditly_breadcrumbs() {
// ... (Previous code)
if (is_tax() || is_category() || is_tag()) {
$term = get_queried_object();
$breadcrumb .= ' » ' . $term->name;
}
// ... (Closing breadcrumb markup)
}
Here, we use get_queried_object()
to retrieve the current term being displayed and add it to the breadcrumb trail.
6. Displaying the Breadcrumbs
To display the breadcrumbs on your site, simply call the accreditly_breadcrumbs()
function within your theme's template files where you want the breadcrumbs to appear. For example, you might add the following code to your header.php
or single.php
file:
<?php accreditly_breadcrumbs(); ?>
7. Styling the Breadcrumbs
Finally, you'll likely want to apply some CSS styles to your breadcrumb navigation to match your site's design. Here's a simple example of how you might style your breadcrumbs:
.breadcrumb {
font-size: 14px;
color: #666;
}
.breadcrumb a {
color: #666;
text-decoration: none;
}
.breadcrumb a:hover {
text-decoration: underline;
}
And that's it! You've successfully implemented dynamic breadcrumbs in your WordPress theme, handling built-in post types, custom post types, and taxonomies. With this feature in place, your users will have an easier time navigating your site, and search engines will have a better understanding of your site's structure.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
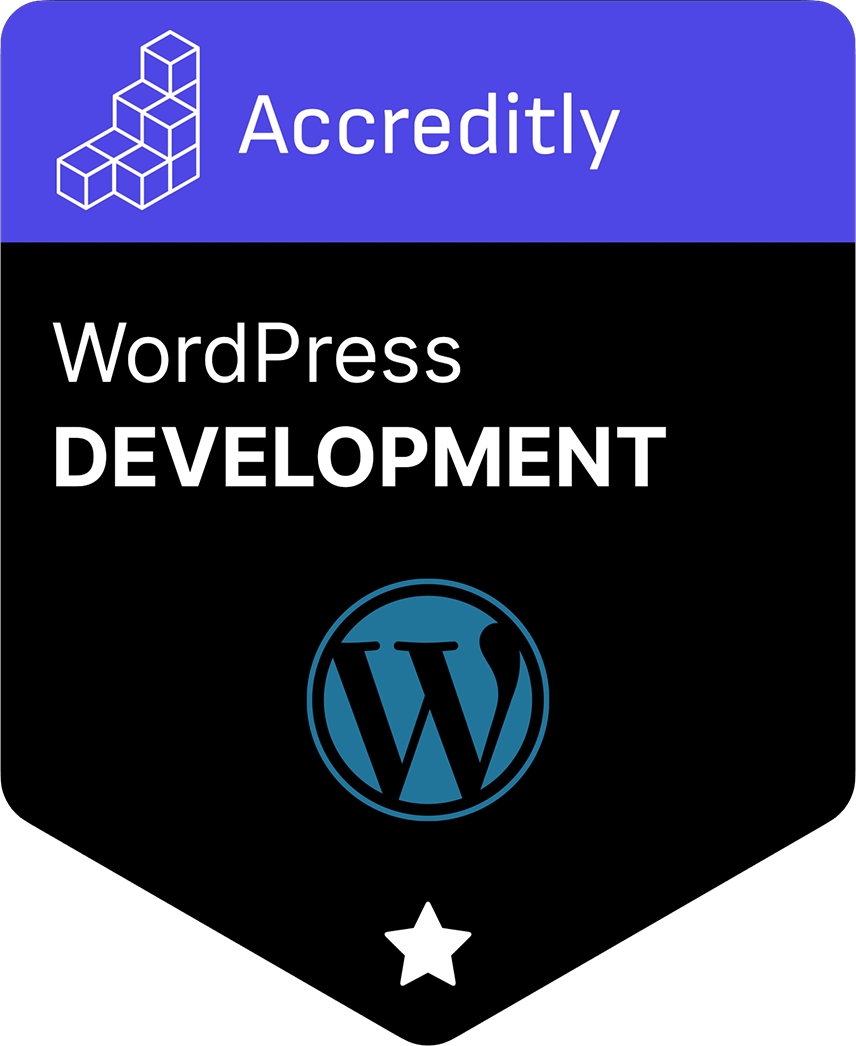