- Understanding srand
- Using srand in PHP
- Seeding Random Numbers with a Blog Post's ID
- More on srand and random_int
- Potential Pitfalls of srand
Random numbers are used in a multitude of programming scenarios - from simulating probability in games to selecting random items from a list. But did you know that you can seed random numbers to produce a consistent pattern of random numbers? In PHP, the srand
function allows you to seed the random number generator, providing the benefit of consistent randomness in your code.
srand
Understanding The srand
function stands for "Seed Random." It is used to seed the random number generator, which can be useful when you need the same sequence of random numbers to be generated consistently across multiple runs of the script. The syntax is as follows:
srand($seed);
Where $seed
is an optional parameter. If not provided, the current timestamp is used as the seed value.
srand
in PHP
Using To use srand
, you simply call it before generating a random number. Although some might think this is obvious, it's also a common pitfall. To be clear:
srand
does not return a random number, instead it seeds the random number generator for future random generations. srand
used alone is useless, you must use a function like rand
or random_int
to actually generate a random number.
srand(10);
echo rand(); // Will always output the same number
Every time you run this script, you will get the same number, as the seed for the random number generator remains the same. If you change the seed, the output will change as well.
srand(20);
echo rand(); // Will output a different number than the previous example
Seeding Random Numbers with a Blog Post's ID
Let's take a look at a real-world scenario: suppose you have a blog and you want to display related posts from the same category on each blog post page. However, instead of showing the same related posts every time, you want to show a random selection. But there's a twist - you want the selection to be consistent for each individual blog post. In other words, every time a user visits a blog post, they see the same set of related posts, but this set is different for each blog post.
Here's how you can achieve this using srand
and random_int
. Some functions used here will need to be created for this to actually work:
// Let's assume $post_id holds the ID of the current blog post and
// $related_posts is an array holding the IDs of all related posts in the same category
$post_id = get_post()['id'];
$related_posts = get_related_posts($post_id); // This is your function to get related posts
// Seed the random number generator with the post ID
srand($post_id);
// Shuffle the related posts array
for ($i = count($related_posts) - 1; $i > 0; $i--) {
$j = random_int(0, $i);
// Swap the elements at indices $i and $j
$temp = $related_posts[$i];
$related_posts[$i] = $related_posts[$j];
$related_posts[$j] = $temp;
}
// Now you can display the related posts, they will be in a random order
// that is consistent for each individual blog post
foreach ($related_posts as $related_post) {
display_post($related_post); // This is your function to display a post
}
In this example, the blog post's ID is used as the seed for the random number generator. This means that the sequence of random numbers generated by random_int
will be consistent for each individual blog post, leading to a consistent shuffle of the related posts array. As a result, each blog post page will display a different, but consistent set of related posts.
In WordPress you can do something like the following:
// Supposing you are in a single post context and want to get related posts
global $post;
// We'll use the post ID as the seed for srand
srand($post->ID);
// Suppose we have a function get_related_posts that fetches related posts based on category
$related_posts = get_related_posts($post->ID);
// We want to select 3 random related posts, but always the same for this post
$random_related_posts_keys = array_rand($related_posts, 3);
// Reset the random seed to avoid affecting other random functions
srand();
// Prepare our array of selected related posts
$random_related_posts = array();
foreach ($random_related_posts_keys as $key) {
$random_related_posts[] = $related_posts[$key];
}
Unfortunately WordPress doesn't have a native way of relating common posts together. You have a number of different options available here, such as:
- Just selecting posts from the same category
- Using some kind of taxonomy to group them, even if this is not shown on the frontend
- Using a post selection via some means, such as ACF Post Object field
- Using a dedicated plugin (although we always recommend not using plugins wherever possible)
In the above example get_related_posts()
handles this aspect, so you'll need to replace that for something more fully-featured that's right for your website.
If you're a WordPress developer then we strongly recommend looking at our WordPress certification.
srand
and random_int
More on While srand
seeds the random number generator and rand generates a random number, it's worth noting that PHP offers another function for generating random integers: random_int
.
The random_int
function is cryptographically secure, which means it's suitable for generating random numbers where security matters, such as for password generation or cryptography. While we don't need this level of security for our blog post example, it's still a good habit to use random_int over rand for a couple of reasons:
- It's not significantly slower or more resource-intensive than
rand
. - Using
random_int
consistently helps to avoid accidentally using rand where a cryptographically secure random number is actually needed.
Here's how you can use random_int
:
echo random_int(0, 10); // Outputs a cryptographically secure random integer between 0 and 10
Remember that random_int
throws an Exception
if it was not able to generate a secure random integer. So, it's a good practice to use it inside a try-catch block:
try {
echo random_int(0, 10);
} catch (Exception $e) {
echo "Could not generate a random integer.";
}
A Random\RandomException
can also be thrown.
srand
Potential Pitfalls of It's important to note that srand
seeds the random number generator globally in your PHP script. This means that if you call srand
somewhere in your script, it will affect the sequence of random numbers generated by rand
or random_int
in all other parts of your script.
Therefore, if you want different parts of your script to generate different sequences of random numbers, you will need to call srand
with a different seed each time.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
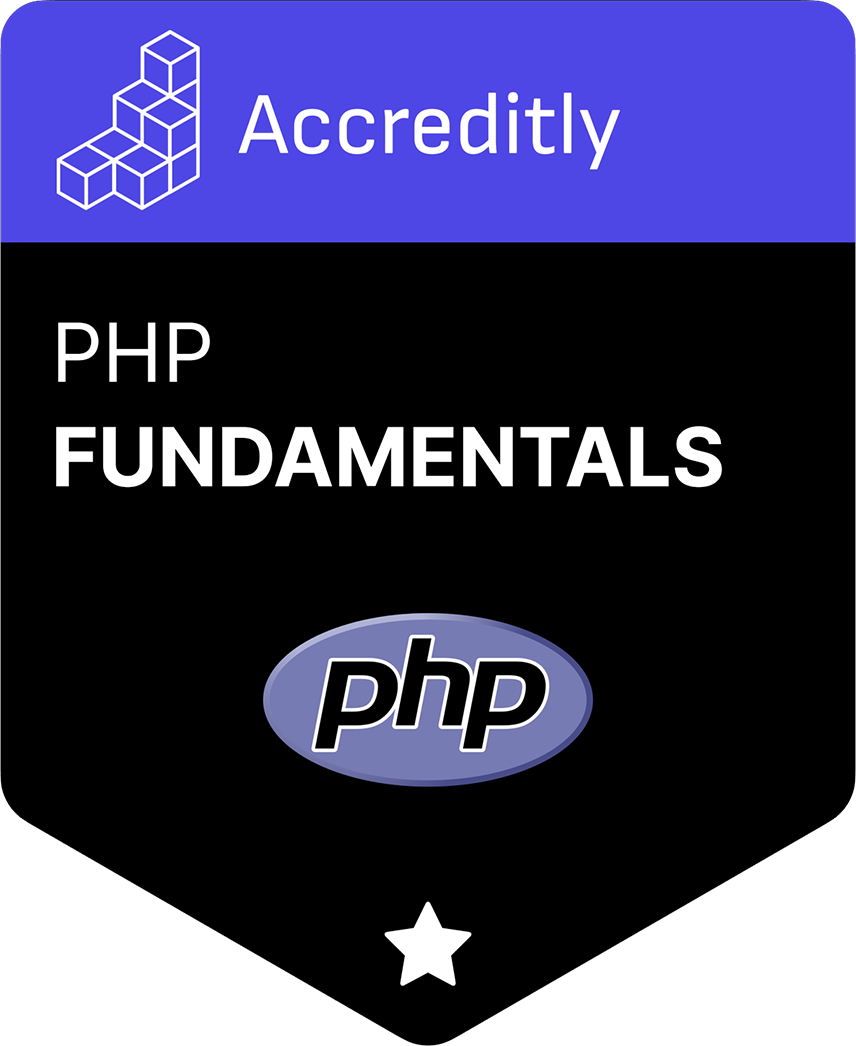