- Understanding HTML Emails with Plaintext Fallback
- Prerequisites
- Step 1: Structure Your Email Content
- Step 2: Create the Email Headers
- Step 3: Combine the Versions
- Step 4: Send the Email
- Best Practices
Sending emails through PHP is a common functionality in many web applications. However, crafting emails that look good and work consistently across different email clients can be challenging. One effective approach is to send HTML emails with a plaintext fallback. This ensures that if the recipient's email client doesn't support or is set not to display HTML content, the email is still readable in plain text.
Understanding HTML Emails with Plaintext Fallback
An email with HTML and plaintext parts contains two versions of the same message. The email client then displays the version best suited to its capabilities or user settings. This approach also improves accessibility and is considered a best practice in email design.
Prerequisites
1. PHP Environment: Ensure you have PHP installed and configured.
2. Mail Sending Function: PHP's mail()
function or a library like PHPMailer to send emails.
Step 1: Structure Your Email Content
Create two versions of your email: one in HTML and one in plaintext.
- HTML Version: Design this like a regular HTML page, but remember that email HTML is different from web HTML. Keep your styling inline and use tables for layout.
```html
Hello, John Doe!
Welcome to our service.
```
- Plaintext Version: Strip all HTML tags and styling to leave only the plain text.
```plaintext
Hello, John Doe!
Welcome to our service.
```
Step 2: Create the Email Headers
The email headers will indicate that the email contains both HTML and plaintext versions.
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-Type: multipart/alternative; boundary=\"boundary_string\"" . "\r\n";
- Replace
"boundary_string"
with a unique string that will not appear anywhere else in your email content.
Step 3: Combine the Versions
Combine the HTML and plaintext versions into a single body with the boundary string. The --boundary_string
separates the different parts of the email.
$message = "--boundary_string\r\n";
$message .= "Content-Type: text/plain; charset=UTF-8\r\n";
$message .= "Content-Transfer-Encoding: 7bit\r\n\r\n";
$message .= $plain_text_version . "\r\n\r\n";
$message .= "--boundary_string\r\n";
$message .= "Content-Type: text/html; charset=UTF-8\r\n";
$message .= "Content-Transfer-Encoding: 7bit\r\n\r\n";
$message .= $html_version . "\r\n\r\n";
$message .= "--boundary_string--";
Step 4: Send the Email
Use PHP's mail()
function to send the email. You can also use a library like PHPMailer for more advanced features.
mail($to, $subject, $message, $headers);
Best Practices
1. Test on Multiple Email Clients: Email clients vary in how they interpret HTML and CSS. Test your emails across different clients to ensure compatibility.
2. Keep HTML Simple: Avoid complex layouts and JavaScript. Stick to simple, inline CSS.
3. Comply with Email Standards: Ensure your emails follow standards to avoid being marked as spam.
4. Accessibility: Consider accessibility best practices, like using alt text for images.
Sending HTML emails with a plaintext fallback in PHP ensures broader compatibility and accessibility, making your emails more professional and user-friendly. Whether you're sending transactional emails, newsletters, or alerts, this method will help you reach all your users effectively.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
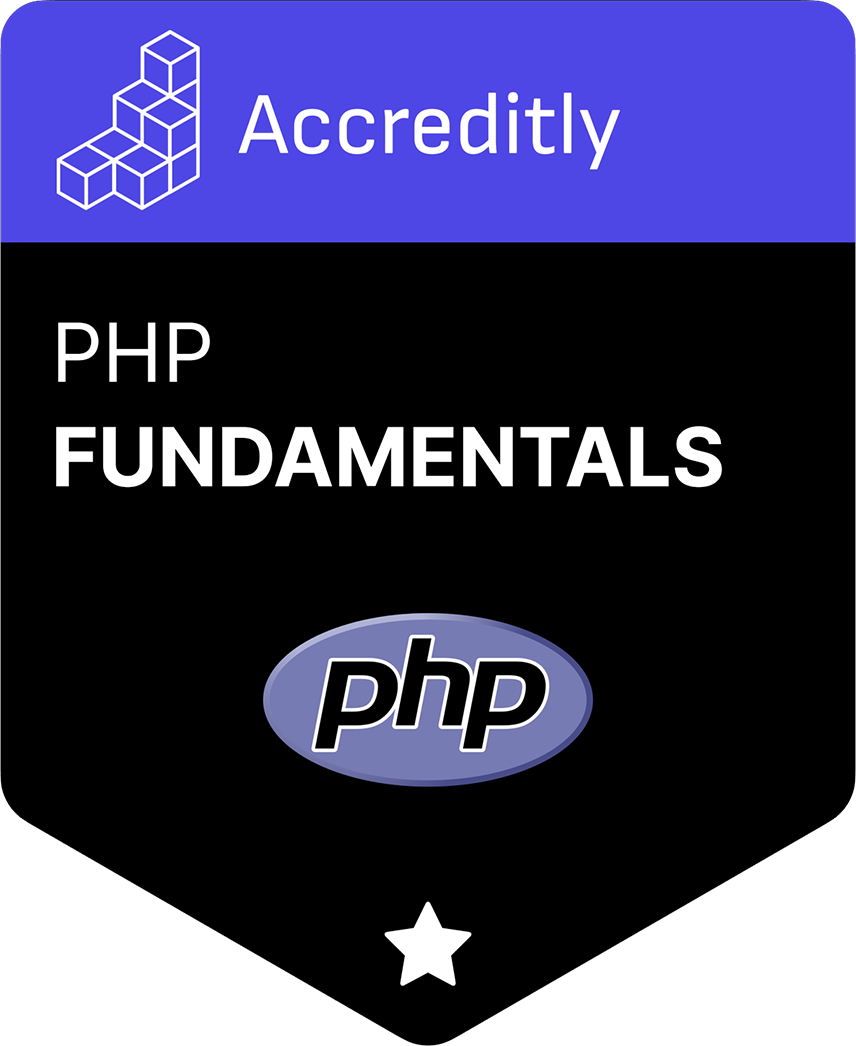