- 1. Why Visual Testing?
- 2. Visual Testing Tools Compatible with PHP
- 3. Setting Up Visual Testing
- 4. Best Practices
- 5. Challenges and Solutions
In an era where user experience reigns supreme, ensuring visual consistency across different devices and browsers is paramount. Visual testing, sometimes referred to as visual regression testing, verifies that the UI appears as expected to users. While PHP is predominantly a server-side language, it can be used in conjunction with various tools to facilitate visual testing for websites. Let's dive in.
1. Why Visual Testing?
Before the how, let's understand the why:
- Consistency: Ensure design and layout consistency across different screen sizes, resolutions, and browsers.
- Quick Feedback: Detect visual regressions early in the development cycle.
- Enhanced User Experience: A visually tested site guarantees optimal user experience by eliminating UI glitches.
2. Visual Testing Tools Compatible with PHP
2.1. Wraith
Wraith is a screenshot comparison tool that uses a headless browser to capture screenshots. It can be integrated with PHP using the command line. Wraith is a tool created and used by the BBC.
Pros:
- Supports multiple browsers.
- Integrates with responsive design testing.
Cons:
- Requires Ruby for setup.
2.2. PHPUnit + Selenium
PHPUnit, the popular PHP testing framework, can be combined with Selenium for browser automation to capture screenshots and conduct visual tests.
Pros:
- Robust and mature solution.
- Can be fully written in PHP.
Cons:
- Slightly steeper learning curve.
3. Setting Up Visual Testing
3.1. Installation
For this guide, we'll focus on the combination of PHPUnit and Selenium:
- Install PHPUnit via Composer:
composer require --dev phpunit/phpunit
- Install Selenium Server and ensure it's running.
3.2. Writing Your First Visual Test
- Create a PHPUnit test case.
- Use Selenium to navigate to the webpage.
- Capture a screenshot.
- Compare the screenshot to a baseline image.
class VisualTest extends PHPUnit\Framework\TestCase {
protected $webDriver;
public function setUp(): void {
$this->webDriver = RemoteWebDriver::create('http://localhost:4444/wd/hub', DesiredCapabilities::chrome());
}
public function testHomePageVisuals() {
$this->webDriver->get('http://yourwebsite.com');
$screenshot = $this->webDriver->takeScreenshot('/path/to/save/screenshot.png');
// Use an image comparison library to compare $screenshot to a baseline image.
}
public function tearDown(): void {
$this->webDriver->quit();
}
}
4. Best Practices
4.1. Establish a Baseline
Always keep a version of your site's visuals that you consider perfect as a baseline. Compare against this version in future tests.
4.2. Automate
Integrate visual testing into your Continuous Integration (CI) process. This ensures each push or pull request doesn't introduce visual regressions.
4.3. Regularly Update Baselines
As designs evolve, so should your baselines. Regularly update them to reflect the latest accurate version of your site.
5. Challenges and Solutions
-
False Positives: Minor changes can trigger test failures. Solution: Implement threshold-based comparisons, allowing minor pixel differences.
-
Dynamic Content: Pages with ever-changing content can cause tests to fail. Solution: Mask or ignore dynamic regions during tests.
Visual testing in PHP, though not commonplace, offers a robust solution to ensure the visual integrity of web applications. With the right tools and practices in place, you can confidently deliver visually stunning and consistent web experiences to your users.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
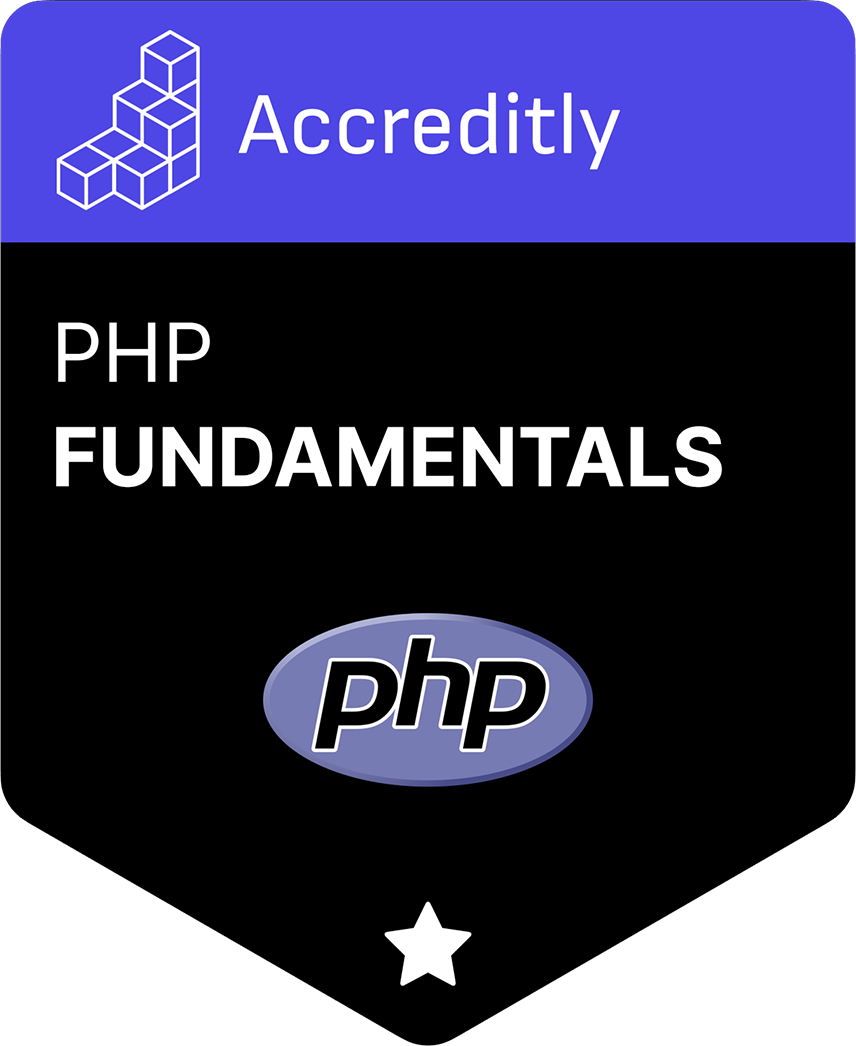