- Understanding Blade Directives
- Why Write Custom Directives?
- The Basics of Creating a Custom Directive
- More Complex Examples
- Best Practices
Laravel Blade, the powerful and elegant templating engine, offers a range of built-in directives for common tasks. However, sometimes you need something more tailored to your specific needs. That's where custom Blade directives come into play. Creating your own directives can make your templates cleaner, more expressive, and more maintainable. Let's explore how to craft these custom directives in Laravel.
Understanding Blade Directives
A Blade directive in Laravel is essentially a custom instruction that you can use in your Blade views. It allows you to define how data should be rendered in the view layer of your application. Common built-in directives include @if
, @foreach
, and @yield
.
Why Write Custom Directives?
Custom directives can simplify complex PHP logic in your Blade views, making them more readable and easier to maintain. For instance, you might frequently format dates in a specific way or include a complex HTML structure. By encapsulating this logic in a directive, you can reuse it effortlessly across your views.
The Basics of Creating a Custom Directive
Creating a custom directive involves two steps: defining the directive and using it in your views.
Step 1: Define Your Directive
Custom Blade directives are defined in the boot
method of a service provider, typically AppServiceProvider
. Use the Blade
facade's directive
method to define your custom directive.
Here's an example of a custom directive that formats a date:
use Illuminate\Support\Facades\Blade;
public function boot() {
Blade::directive('formatDate', function ($expression) {
return "<?php echo ($expression)->format('m/d/Y'); ?>";
});
}
This directive can now be used to format date objects in your Blade views.
Step 2: Use Your Directive in Views
Once defined, you can use your custom directive in any Blade view:
@formatDate($post->created_at)
This will output the created_at
date of a post formatted as m/d/Y
.
More Complex Examples
Your custom directives can be as simple or complex as you need. For example, a directive to include inline SVGs might look like this:
Blade::directive('inlineSvg', function ($expression) {
$path = public_path("svg/{$expression}.svg");
return file_exists($path) ? file_get_contents($path) : '';
});
In your Blade file:
@inlineSvg('logo')
Best Practices
-
Clear Naming: Name your directives clearly and descriptively. The name should give a good indication of what the directive does.
-
Avoid Overuse: While custom directives are powerful, overusing them can make your code harder to understand. Use them for repetitive and complex tasks that significantly benefit from abstraction.
-
Documentation: Document your custom directives, especially if you're working in a team. This ensures that everyone understands what each directive does and how to use it.
-
Abstract into files: Don't litter your
AppServiceProvider.php
file with lots of Blade directives, instead load them from separate files to try and keep your files clean and succinct. However, try not to over-abstract, so you only really need to do this if you have more than one or two Blade directives, or if they're particularly complex.
Writing your own Laravel Blade directives is a simple yet powerful way to extend the capabilities of your views. Custom directives can encapsulate complex logic, making your templates cleaner and more intuitive. Whether it's formatting data, injecting reusable HTML, or any other repetitive task, a well-crafted directive can be a game-changer in your Laravel development process.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
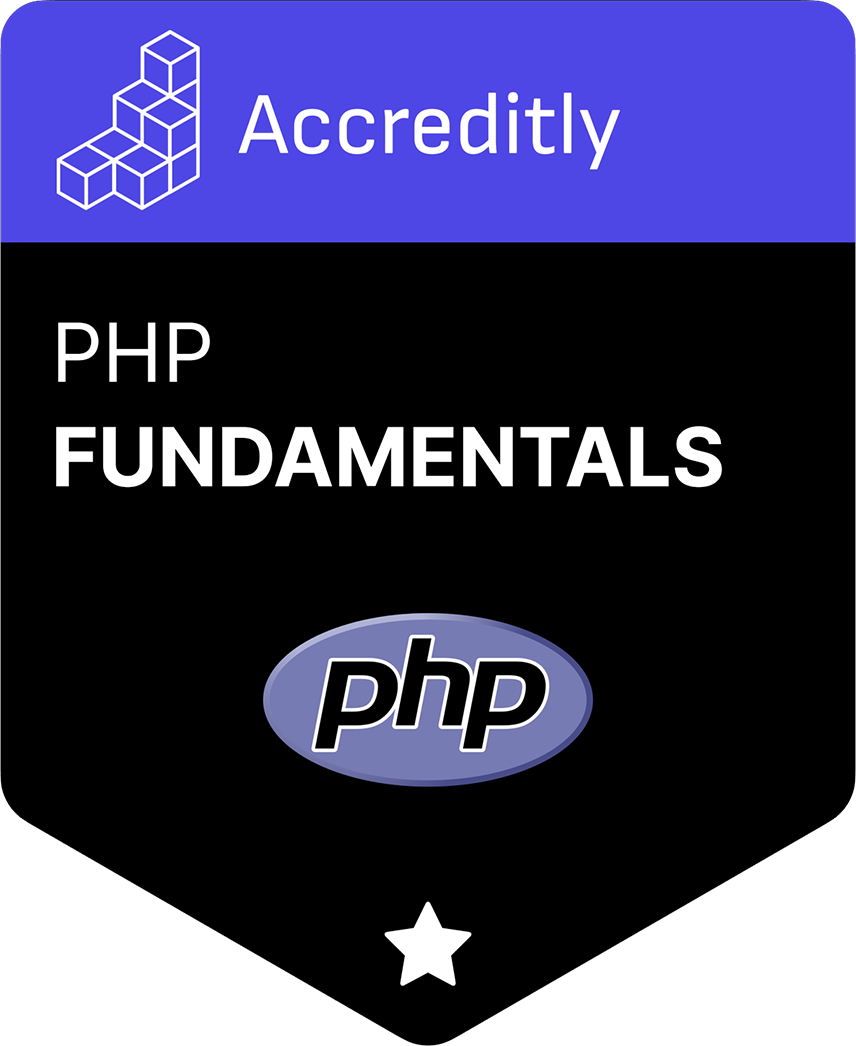