- Understanding Zero-Based Month Indexing
- Why Zero-Based Indexing?
- Working with Months in JavaScript
- Handling Month Display for Users
- Conclusion
When it comes to date handling in JavaScript, one of the first stumbling blocks developers encounter is the zero-based indexing of months. In JavaScript's Date
object, months are indexed starting from 0, meaning January is represented as 0, February as 1, all the way to December, which is represented as 11. This convention can be counterintuitive, especially for those coming from other programming languages or backgrounds where January is traditionally represented as the first month of the year. This article explores how to handle months in JavaScript, with a focus on navigating the '0' month of January.
Understanding Zero-Based Month Indexing
JavaScript's Date
object follows a zero-based indexing system for months as part of its design. This means when you're creating a new date or retrieving the month from a Date
object, you'll need to adjust your thinking (and calculations) to accommodate this setup.
Creating a Date in January:
const january = new Date(2023, 0, 1);
console.log(january); // Logs the date corresponding to January 1st, 2023
In this example, when specifying 0
as the second argument to the Date
constructor, you're effectively creating a date in January.
Why Zero-Based Indexing?
The zero-based indexing for months in JavaScript is consistent with other programming languages and systems where array indexes start at 0. This approach provides some mathematical advantages in computations and array manipulations, making it a common practice in computer science. However, it does require developers to make a mental shift when dealing with human-friendly date representations.
Working with Months in JavaScript
Getting the Month from a Date:
When retrieving the month from a Date
object, remember that the returned value will range from 0 (January) to 11 (December).
const now = new Date();
const month = now.getMonth();
console.log(month); // Could log '0' for January
Setting the Month of a Date:
Similarly, when setting the month of a Date
object using the setMonth()
method, you'll use the zero-based index.
const date = new Date();
date.setMonth(0); // Sets the month to January
Handling Month Display for Users
When displaying dates to users, you'll likely want to show them in a more familiar, human-readable format. JavaScript provides several ways to format dates, including methods like toLocaleDateString()
which respects the local setting of the user's browser, automatically adjusting the month display accordingly.
const date = new Date();
console.log(date.toLocaleDateString('en-US', { month: 'long', year: 'numeric', day: 'numeric' }));
// Outputs "January 1, 2023" in the US locale
For more customized date formatting, especially in applications where you need full control over the output, consider using a library like date-fns
or Day.js
. These libraries offer straightforward APIs for formatting dates and can simplify handling zero-based month indexing.
import { format } from 'date-fns';
const date = new Date();
console.log(format(date, 'MMMM dd, yyyy'));
// Outputs "January 01, 2023"
Conclusion
The zero-based month indexing in JavaScript's Date
object is a quirk that can catch many developers off guard. By understanding and anticipating this behavior, you can effectively manipulate and display dates in your JavaScript applications. Whether you're directly manipulating Date
objects or leveraging powerful third-party libraries, handling dates, and specifically the '0' month of January, becomes a manageable task with practice and familiarity. Remember, the key to smooth date handling in JavaScript lies in recognizing the system's design and applying consistent logic throughout your date-related code.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
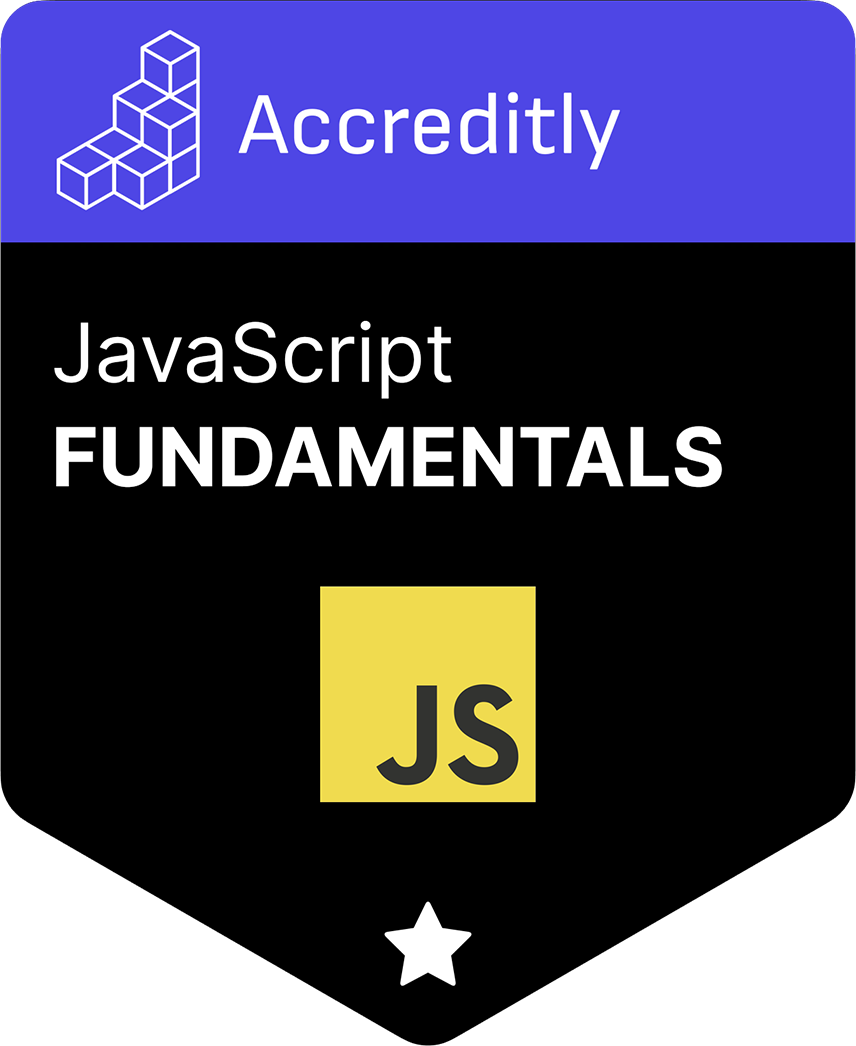
Related articles
Tutorials WordPress PHP Cyber Security
How to check your WordPress core installation for unauthorised changes
Safeguard your WordPress site by learning how to check your WordPress core installation for unauthorised changes. This article provides a step-by-step approach to maintaining the security and integrity of your WordPress core files.