- What is GROUP BY?
- Simple Example of GROUP BY
- Using GROUP BY with Multiple Columns
- Common Aggregate Functions Used with GROUP BY
- GROUP BY and HAVING
- GROUP BY with ORDER BY
- Common Pitfalls with GROUP BY
- Best Practices for Using GROUP BY
- Advanced GROUP BY Techniques
- Conclusion: Mastering GROUP BY
MySQL's GROUP BY
clause is one of the most powerful tools in SQL for data aggregation. It allows you to group rows that share a common field value and perform aggregate functions such as SUM()
, COUNT()
, AVG()
, MAX()
, and MIN()
on those groups. Despite its simplicity, GROUP BY
can be challenging for newcomers to SQL and even seasoned developers due to various nuances and potential pitfalls. This article will explore GROUP BY
in MySQL, providing a comprehensive explanation, numerous examples, common mistakes, and best practices to ensure you're using it effectively.
What is GROUP BY?
In MySQL, the GROUP BY
clause is used in SQL queries to arrange identical data into groups. It’s commonly used with aggregate functions to perform operations on each group of data. The basic syntax is as follows:
SELECT column_name, aggregate_function(column_name)
FROM table_name
WHERE condition
GROUP BY column_name;
Here’s a breakdown:
-
column_name
: The column or columns you want to group by. -
aggregate_function
: A function likeSUM()
,COUNT()
,AVG()
, etc., that performs calculations on each group.
Simple Example of GROUP BY
Let’s start with a simple example. Consider a table sales
that records daily sales for different products:
CREATE TABLE sales (
id INT AUTO_INCREMENT PRIMARY KEY,
product_name VARCHAR(100),
sale_date DATE,
amount DECIMAL(10, 2)
);
INSERT INTO sales (product_name, sale_date, amount) VALUES
('Product A', '2024-08-01', 150.00),
('Product B', '2024-08-01', 200.00),
('Product A', '2024-08-02', 170.00),
('Product B', '2024-08-02', 220.00),
('Product A', '2024-08-03', 180.00);
Now, to calculate the total sales for each product, you would use GROUP BY
:
SELECT product_name, SUM(amount) AS total_sales
FROM sales
GROUP BY product_name;
Result:
product_name | total_sales |
---|---|
Product A | 500.00 |
Product B | 420.00 |
Using GROUP BY with Multiple Columns
GROUP BY
can also be used with multiple columns. For example, if you wanted to find out the total sales per product for each day, you would modify the query as follows:
SELECT product_name, sale_date, SUM(amount) AS total_sales
FROM sales
GROUP BY product_name, sale_date;
Result:
product_name | sale_date | total_sales |
---|---|---|
Product A | 2024-08-01 | 150.00 |
Product A | 2024-08-02 | 170.00 |
Product A | 2024-08-03 | 180.00 |
Product B | 2024-08-01 | 200.00 |
Product B | 2024-08-02 | 220.00 |
In this query, GROUP BY
creates a unique combination of product_name
and sale_date
, and the SUM()
function is applied to each group.
Common Aggregate Functions Used with GROUP BY
- COUNT(): Counts the number of rows in each group.
- SUM(): Adds up the values in each group.
- AVG(): Calculates the average value of the group.
- MAX(): Returns the maximum value in each group.
- MIN(): Returns the minimum value in each group.
Let’s take a look at each of these in action.
COUNT()
Suppose you want to know how many sales entries exist for each product:
SELECT product_name, COUNT(*) AS sales_count
FROM sales
GROUP BY product_name;
Result:
product_name | sales_count |
---|---|
Product A | 3 |
Product B | 2 |
AVG()
If you’re interested in finding out the average sale amount for each product:
SELECT product_name, AVG(amount) AS average_sales
FROM sales
GROUP BY product_name;
Result:
product_name | average_sales |
---|---|
Product A | 166.67 |
Product B | 210.00 |
MAX() and MIN()
To determine the highest and lowest sales for each product:
SELECT product_name, MAX(amount) AS highest_sale, MIN(amount) AS lowest_sale
FROM sales
GROUP BY product_name;
Result:
product_name | highest_sale | lowest_sale |
---|---|---|
Product A | 180.00 | 150.00 |
Product B | 220.00 | 200.00 |
GROUP BY and HAVING
The HAVING
clause is often used with GROUP BY
to filter groups based on aggregate functions, similar to how the WHERE
clause filters rows.
For example, if you wanted to find products with total sales greater than $450:
SELECT product_name, SUM(amount) AS total_sales
FROM sales
GROUP BY product_name
HAVING total_sales > 450;
Result:
product_name | total_sales |
---|---|
Product A | 500.00 |
GROUP BY with ORDER BY
You can use the ORDER BY
clause in conjunction with GROUP BY
to sort the grouped results. For instance, to order the total sales from highest to lowest:
SELECT product_name, SUM(amount) AS total_sales
FROM sales
GROUP BY product_name
ORDER BY total_sales DESC;
Result:
product_name | total_sales |
---|---|
Product A | 500.00 |
Product B | 420.00 |
Common Pitfalls with GROUP BY
While GROUP BY
is powerful, it can be tricky, leading to potential pitfalls. Let’s discuss some common mistakes and how to avoid them.
1. Using Non-Aggregate Columns in SELECT
One of the most common mistakes is using columns in the SELECT
statement that are not part of the GROUP BY
clause and are not used with aggregate functions. This can lead to unexpected results or SQL errors.
For example:
SELECT id, product_name, SUM(amount)
FROM sales
GROUP BY product_name;
This query will throw an error because id
is neither in the GROUP BY
clause nor used in an aggregate function. MySQL requires that every column in the SELECT
clause must either be in the GROUP BY
clause or used with an aggregate function.
To fix this, you should either add id
to the GROUP BY
clause (if it makes logical sense) or remove it from the SELECT
statement.
2. Misusing GROUP BY with DISTINCT
Sometimes, developers mistakenly use DISTINCT
with GROUP BY
, not realizing that GROUP BY
already provides unique grouping. Using DISTINCT
in such cases is redundant and can affect performance.
SELECT DISTINCT product_name, SUM(amount)
FROM sales
GROUP BY product_name;
This query is unnecessary; the DISTINCT
keyword does nothing here. The correct approach is to remove DISTINCT
:
SELECT product_name, SUM(amount)
FROM sales
GROUP BY product_name;
3. Ignoring NULL Values
When grouping data, be aware of how NULL
values are handled. MySQL considers all NULL
values as equal for grouping purposes. If your data contains NULL
values, they will be grouped together in a single group.
For example, if the product_name
column has NULL
values:
INSERT INTO sales (product_name, sale_date, amount) VALUES
(NULL, '2024-08-04', 300.00);
And you run the following query:
SELECT product_name, COUNT(*) AS count_sales
FROM sales
GROUP BY product_name;
Result:
product_name | count_sales |
---|---|
Product A | 3 |
Product B | 2 |
NULL | 1 |
The NULL
values are grouped into their own category. If you want to exclude NULL
values from the result, add a WHERE
clause:
SELECT product_name, COUNT(*) AS count_sales
FROM sales
WHERE product_name IS NOT NULL
GROUP BY product_name;
Best Practices for Using GROUP BY
To make the most out of GROUP BY
and avoid common issues, follow these best practices:
1. Use Explicit Column Names
Always specify the exact columns you want to include in the GROUP BY
clause, rather than relying on implicit grouping. This makes your queries clearer and easier to understand.
2. Avoid Redundant GROUP BY Clauses
If your query doesn’t require grouping, avoid using GROUP BY
. Unnecessary grouping can lead to performance degradation, especially with large datasets.
3. Optimize with Indexes
If you frequently use `
GROUP BY` on certain columns, consider adding indexes to those columns. Indexes can significantly improve query performance by reducing the amount of data MySQL needs to scan.
CREATE INDEX idx_product_name ON sales(product_name);
4. Be Cautious with Large Datasets
When working with large datasets, be aware that GROUP BY
operations can be resource-intensive. Use LIMIT
or paginate results if you're dealing with a significant amount of data.
SELECT product_name, SUM(amount)
FROM sales
GROUP BY product_name
LIMIT 10;
5. Consider Using Subqueries
Sometimes, using a subquery can simplify your GROUP BY
logic, especially if you need to perform additional calculations or filtering on the grouped data.
SELECT product_name, total_sales
FROM (
SELECT product_name, SUM(amount) AS total_sales
FROM sales
GROUP BY product_name
) AS grouped_sales
WHERE total_sales > 450;
Advanced GROUP BY Techniques
Now that we’ve covered the basics and some intermediate concepts, let’s dive into more advanced techniques for using GROUP BY
in MySQL.
GROUP BY with ROLLUP
The ROLLUP
modifier is an extension to GROUP BY
that allows you to calculate subtotals and grand totals in your result set. This is particularly useful for financial reports or hierarchical data.
For example, let’s calculate the total sales per product, along with the overall total:
SELECT product_name, SUM(amount) AS total_sales
FROM sales
GROUP BY product_name WITH ROLLUP;
Result:
product_name | total_sales |
---|---|
Product A | 500.00 |
Product B | 420.00 |
NULL | 920.00 |
The NULL
in the result represents the grand total of all sales.
GROUP BY with CASE Statements
You can combine GROUP BY
with CASE
statements to create more complex groupings based on conditional logic.
Suppose you want to group sales into categories: “High Sales” for amounts over $200 and “Low Sales” for amounts under $200:
SELECT
CASE
WHEN amount > 200 THEN 'High Sales'
ELSE 'Low Sales'
END AS sale_category,
COUNT(*) AS count_sales
FROM sales
GROUP BY sale_category;
Result:
sale_category | count_sales |
---|---|
High Sales | 3 |
Low Sales | 2 |
Conclusion: Mastering GROUP BY
The GROUP BY
clause in MySQL is indispensable for data aggregation and reporting. By grouping data, applying aggregate functions, and using techniques like ROLLUP
and CASE
statements, you can derive meaningful insights from your datasets.
While powerful, GROUP BY
also comes with potential pitfalls, especially regarding non-aggregate columns, redundant grouping, and handling NULL
values. Following best practices—such as using explicit column names, optimizing with indexes, and considering subqueries—will help you avoid these issues and make your queries more efficient.
Understanding and mastering GROUP BY
in MySQL is essential for anyone working with relational databases. Whether you’re analyzing sales data, generating reports, or simply summarizing information, GROUP BY
allows you to perform complex queries with ease and efficiency.
For further reading, the MySQL documentation on GROUP BY is an excellent resource, offering more detailed explanations and examples to help deepen your understanding.
Interested in proving your knowledge of this topic? Take the Database Fundamentals certification.
Database Fundamentals
Focusing on SQL and database design, this exam will test your knowledge of database fundamentals.
$99
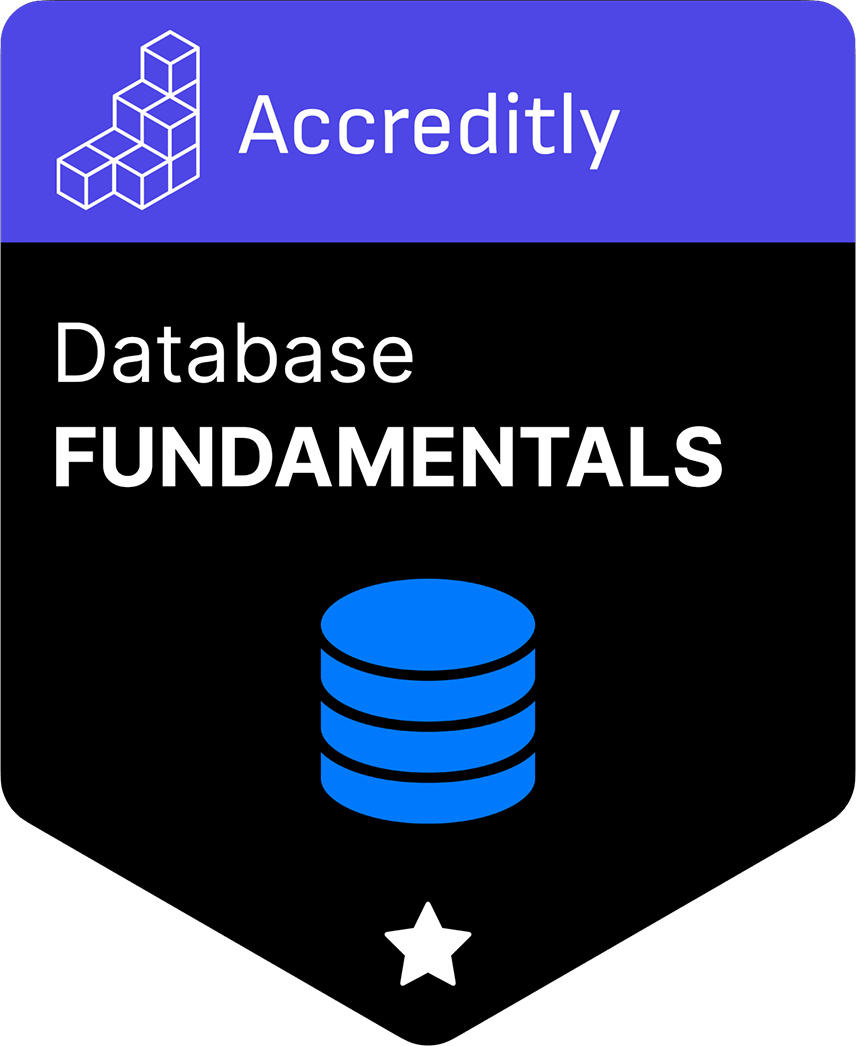