- Why Naming Conventions Matter
- Basics of Laravel Relationships
- Joining Tables in Laravel
- Naming Convention for Joining Tables
- 1. Table Name Composition
- 2. Column Names in the Joining Table
- 3. Timestamps and Additional Columns
- Customizing Table and Column Names
- Custom Table Name
- Custom Column Names
- Benefits of Following Laravel’s Naming Convention
Among its many features, Laravel’s Eloquent ORM (Object-Relational Mapping) shines in simplifying database interactions. However, to fully leverage Eloquent, it’s crucial to follow Laravel’s naming conventions, especially when dealing with joining tables in a relational database. This article explores Laravel's naming conventions for joining tables, ensuring that your application remains maintainable and consistent.
Why Naming Conventions Matter
Naming conventions in Laravel (or any framework) are more than just a matter of aesthetics—they play a significant role in the functionality and clarity of your code. Properly naming your tables and columns ensures that Laravel can automatically recognize relationships and generate SQL queries with minimal configuration. When you adhere to Laravel’s conventions, you reduce the risk of errors and make your codebase more intuitive for other developers.
Basics of Laravel Relationships
Before diving into naming conventions, it’s essential to understand how Laravel handles relationships between tables:
- One-to-One: A relationship where a single record in a table corresponds to a single record in another table.
- One-to-Many: A relationship where a single record in one table can relate to many records in another.
- Many-to-Many: A relationship where records in one table can relate to many records in another and vice versa. This is where joining tables, also known as pivot tables, come into play.
Joining Tables in Laravel
In Laravel, many-to-many relationships are typically managed through a joining table (also referred to as a pivot table). This table doesn’t need a primary key or a model class but serves as an intermediary to link two related tables.
Naming Convention for Joining Tables
Laravel follows a specific convention for naming joining tables in many-to-many relationships. This convention helps Laravel recognize and correctly map the relationship without additional configuration.
1. Table Name Composition
The name of the joining table should be a combination of the two related table names, ordered alphabetically and separated by an underscore (_
). For example:
- If you have two tables named
users
androles
, the joining table should be namedrole_user
. - If your tables are
posts
andtags
, the joining table should bepost_tag
.
The alphabetical order is crucial. Laravel expects the tables to be named in this manner, so a joining table named user_role
instead of role_user
would require you to manually specify the table name in the relationship method, which is unnecessary overhead.
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class);
}
}
class Role extends Model
{
public function users()
{
return $this->belongsToMany(User::class);
}
}
In the above example, Laravel automatically assumes the existence of a role_user
table to manage the relationship between users and roles. There’s no need to specify the table name, as Laravel adheres to its naming convention.
2. Column Names in the Joining Table
The joining table should consist of two foreign key columns, each named after the corresponding table’s singular form, followed by _id
. For instance:
- In the
role_user
table, the columns should berole_id
anduser_id
. - In the
post_tag
table, the columns should bepost_id
andtag_id
.
This naming pattern allows Laravel to identify the correct keys when establishing the relationship.
CREATE TABLE role_user (
role_id BIGINT UNSIGNED NOT NULL,
user_id BIGINT UNSIGNED NOT NULL,
FOREIGN KEY (role_id) REFERENCES roles(id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
3. Timestamps and Additional Columns
Although not mandatory, it’s often useful to include created_at
and updated_at
timestamps in your joining tables. This is especially helpful if you need to track when a relationship was established.
CREATE TABLE role_user (
role_id BIGINT UNSIGNED NOT NULL,
user_id BIGINT UNSIGNED NOT NULL,
created_at TIMESTAMP NULL,
updated_at TIMESTAMP NULL,
FOREIGN KEY (role_id) REFERENCES roles(id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
If your joining table requires additional attributes beyond the foreign keys (such as status
or order
), you might need to create a dedicated model for it. This model can then be used like any other Eloquent model.
class RoleUser extends Model
{
protected $table = 'role_user';
protected $fillable = ['role_id', 'user_id', 'status'];
}
Customizing Table and Column Names
While following Laravel’s conventions simplifies the process, there are scenarios where you might need to customize the table or column names. Laravel allows you to specify these in your Eloquent models.
Custom Table Name
If your joining table doesn’t follow the naming convention, you can specify the table name using the ->withPivot()
method in your relationship definition.
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class, 'user_role');
}
}
In this example, Laravel is explicitly told to use the user_role
table instead of the default role_user
.
Custom Column Names
Similarly, if your joining table’s columns don’t follow the convention, you can specify them in the relationship method.
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class, 'user_role', 'custom_user_id', 'custom_role_id');
}
}
Here, Laravel is informed that the foreign key columns in the user_role
table are custom_user_id
and custom_role_id
.
Benefits of Following Laravel’s Naming Convention
Adhering to Laravel’s naming convention for joining tables offers several advantages:
-
Automatic Relationship Mapping: Laravel can automatically detect and manage relationships, reducing the need for manual configuration.
-
Consistency: Following a consistent naming convention makes your codebase easier to read and maintain, particularly in teams or for future developers.
-
Error Reduction: Proper naming conventions minimize the risk of errors in your database queries and migrations.
-
Code Simplicity: By adhering to conventions, you avoid cluttering your models with unnecessary custom configurations, keeping your code cleaner.
Laravel’s naming conventions for joining tables are not just recommendations—they’re a key part of what makes the framework so powerful and developer-friendly. By following these conventions, you enable Laravel to handle much of the heavy lifting in managing relationships, allowing you to focus on building the core features of your application.
Remember, while Laravel provides the flexibility to customize table and column names when necessary, sticking to the conventions wherever possible will save you time and headaches down the road. As with many things in programming, consistency is key, and Laravel’s naming conventions are a tried-and-true path to consistent, maintainable code.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
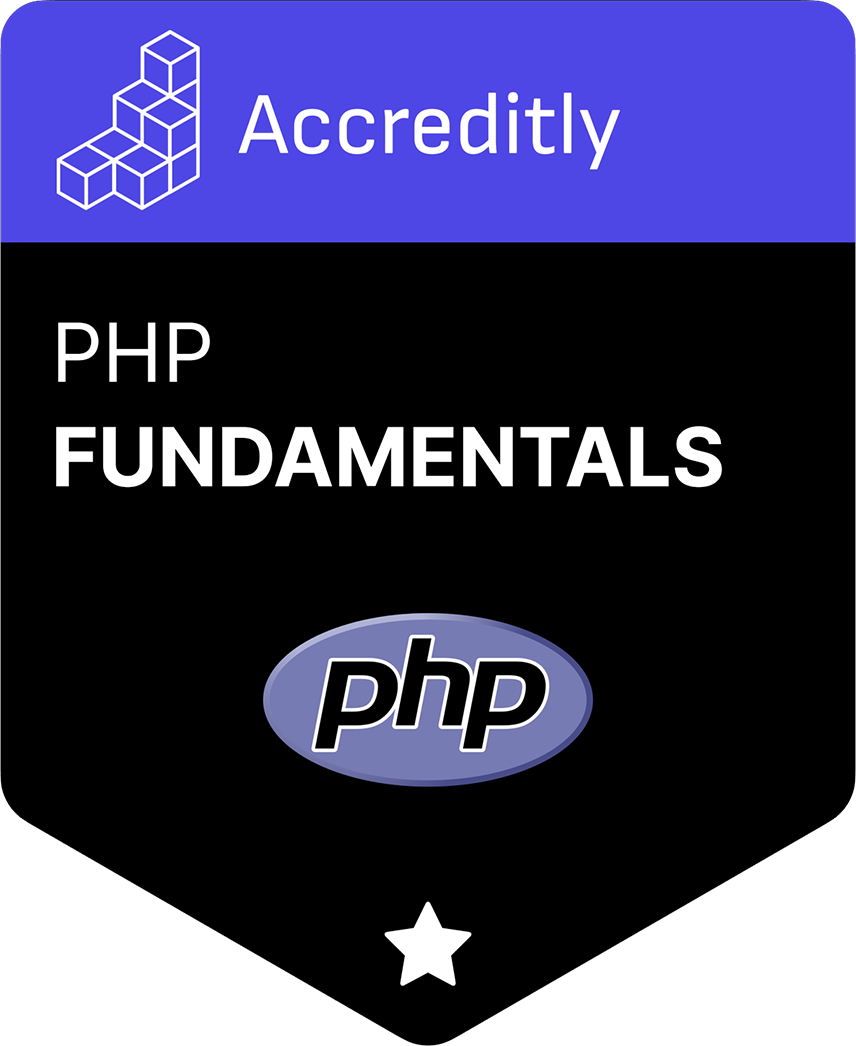