- 1. Array.prototype.reduce()
- 2. Object.entries()
- 3. Array.prototype.some() and Array.prototype.every()
- 4. String.prototype.padStart() and String.prototype.padEnd()
- 5. parseInt()
JavaScript is full of powerful features and functions. However, not all of them get the attention they deserve. Some of the most potent JavaScript tools are often overlooked, hidden under the shadow of their more well-known counterparts. In this article, we'll unveil five such functions, shedding light on their capabilities and showing how they can be used to improve your codebase.
Array.prototype.reduce()
1. The reduce()
function can transform an array into a single value, be it a number, an object, or another array. While map()
and filter()
get a lot of attention, reduce()
is often overlooked despite its powerful capabilities.
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue);
console.log(sum); // Outputs: 15
Object.entries()
2. Object.entries()
returns an array of a given object's enumerable string-keyed property [key, value]
pairs. It allows you to convert an object to an array and iterate over it, which can be incredibly useful in certain situations.
let obj = { 'a': 1, 'b': 2, 'c': 3 };
let entries = Object.entries(obj);
console.log(entries);
// Outputs: [['a', 1], ['b', 2], ['c', 3]]
Array.prototype.some()
and Array.prototype.every()
3. These two functions are used to test whether at least one element (for some()
) or all elements (for every()
) in the array pass the test implemented by the provided function.
let numbers = [1, 2, 3, 4, 5];
let areAllNumbersEven = numbers.every(number => number % 2 === 0);
let isThereAnEvenNumber = numbers.some(number => number % 2 === 0);
console.log(areAllNumbersEven); // Outputs: false
console.log(isThereAnEvenNumber); // Outputs: true
String.prototype.padStart()
and String.prototype.padEnd()
4. These two functions pad the start or end of a string with another string (multiple times, if needed) until the resulting string reaches the given length. This can be very useful when formatting output.
let str = '5';
console.log(str.padStart(3, '0')); // Outputs: '005'
console.log(str.padEnd(3, '!')); // Outputs: '5!!'
parseInt()
5. While parseInt()
is well-known, its radix parameter (which specifies the base in mathematical numeral systems) is often overlooked. By setting the radix, you can parse binary, octal, hexadecimal numbers and beyond.
console.log(parseInt('11', 2)); // Outputs: 3 - '11' is 3 in binary
console.log(parseInt('10', 8)); // Outputs: 8 - '10' is 8 in octal
console.log(parseInt('F', 16)); // Outputs: 15 - 'F' is 15 in hexadecimal
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
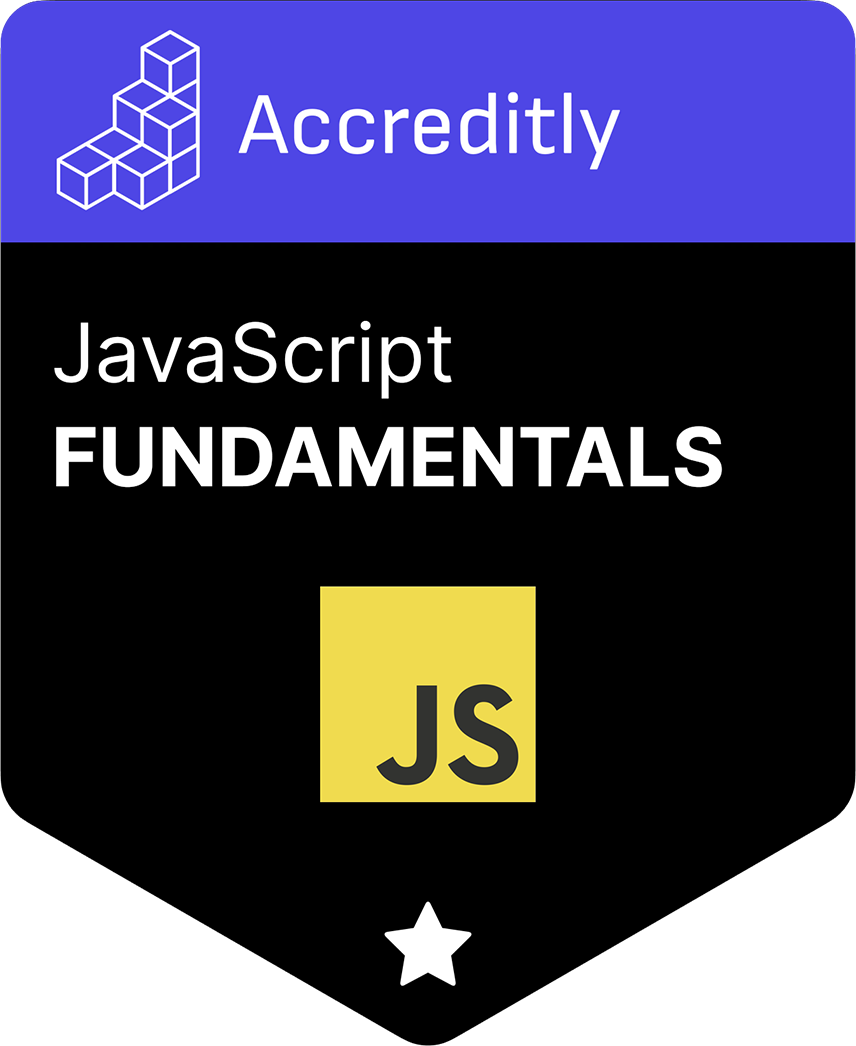