- Introduction to tsconfig.json
- Creating a tsconfig.json File
- Structure of tsconfig.json
- Compiler Options
- Files, Include, and Exclude
- References
- Advanced Configuration
- Real-World Examples
- Wrapping up
The tsconfig.json
file is a cornerstone of any TypeScript project. It allows developers to define how TypeScript should behave and how it should compile the code. This article delves into the tsconfig.json
file, covering its structure, key properties, and providing examples to help you configure it effectively for your TypeScript projects.
tsconfig.json
Introduction to The tsconfig.json
file is a JSON configuration file used by the TypeScript compiler (tsc
). It specifies the root files and the compiler options required to compile the project. By configuring the tsconfig.json
, you can control aspects such as the JavaScript version output, module system, strictness checks, and more.
tsconfig.json
File
Creating a You can create a tsconfig.json
file in the root directory of your TypeScript project. The simplest way to generate this file is to use the TypeScript compiler:
tsc --init
This command generates a tsconfig.json
file with default configurations.
tsconfig.json
Structure of A tsconfig.json
file typically consists of the following sections:
- Compiler Options: Configures the TypeScript compiler's behavior.
- Files: Specifies a list of files to be included in the compilation.
- Include: Specifies a list of glob patterns to include in the compilation.
- Exclude: Specifies a list of glob patterns to exclude from the compilation.
- References: Configures project references for multi-project setups.
Let's explore each section in detail.
Compiler Options
The compilerOptions
section allows you to define how the TypeScript compiler should process your code. This section is extensive, with numerous properties to control various aspects of the compilation process.
Basic Options
-
target
: Specifies the JavaScript language version for emitted JavaScript and includes compatible library declarations. Default ises3
."compilerOptions": { "target": "es6" }
-
module
: Determines the module system for the generated JavaScript code. Options includecommonjs
,amd
,es6
,esnext
, etc."compilerOptions": { "module": "commonjs" }
-
lib
: Specifies the library files to be included in the compilation."compilerOptions": { "lib": ["es6", "dom"] }
-
allowJs
: Allows JavaScript files to be compiled."compilerOptions": { "allowJs": true }
-
checkJs
: Report errors in.js
files."compilerOptions": { "checkJs": true }
-
jsx
: Determines how JSX code is compiled. Options includepreserve
,react
, andreact-native
."compilerOptions": { "jsx": "react" }
Strict Type-Checking Options
TypeScript provides several strict type-checking options to ensure the quality and correctness of your code. Enabling these options can help catch errors early in the development process.
-
strict
: Enables all strict type-checking options."compilerOptions": { "strict": true }
-
noImplicitAny
: Raises an error on expressions and declarations with an impliedany
type."compilerOptions": { "noImplicitAny": true }
-
strictNullChecks
: Ensures thatnull
andundefined
are only assignable to themselves and any, preventing many common errors."compilerOptions": { "strictNullChecks": true }
-
strictFunctionTypes
: Ensures that function types are checked more strictly."compilerOptions": { "strictFunctionTypes": true }
-
strictPropertyInitialization
: Ensures class properties are initialized in the constructor."compilerOptions": { "strictPropertyInitialization": true }
Module Resolution Options
Module resolution options control how TypeScript resolves modules.
-
baseUrl
: Sets the base directory for resolving non-relative module names."compilerOptions": { "baseUrl": "./" }
-
paths
: Configures a mapping of module paths to locations relative to thebaseUrl
."compilerOptions": { "paths": { "@app/*": ["src/app/*"], "@components/*": ["src/components/*"] } }
-
rootDirs
: Specifies a list of root directories whose combined content represents the structure of the project at runtime."compilerOptions": { "rootDirs": ["src", "generated"] }
-
moduleResolution
: Determines how modules are resolved. Options includenode
andclassic
."compilerOptions": { "moduleResolution": "node" }
Source Map Options
Source maps help in debugging by mapping the compiled JavaScript code back to the original TypeScript code.
-
sourceMap
: Generates corresponding.map
files for easier debugging."compilerOptions": { "sourceMap": true }
-
inlineSourceMap
: Embeds the source map as a data URL in the output file."compilerOptions": { "inlineSourceMap": true }
-
inlineSources
: Embed the original source files in the source map."compilerOptions": { "inlineSources": true }
Additional Options
TypeScript provides numerous other options to fine-tune the compilation process.
-
outDir
: Specifies the output directory for the compiled files."compilerOptions": { "outDir": "./dist" }
-
removeComments
: Removes all comments from the output files."compilerOptions": { "removeComments": true }
-
noEmitOnError
: Prevents the compiler from emitting files if there are any errors."compilerOptions": { "noEmitOnError": true }
Files, Include, and Exclude
Apart from compiler options, tsconfig.json
allows you to specify which files to include and exclude from the compilation process.
Files
The files
property specifies an array of filenames to be included in the compilation.
"files": [
"src/main.ts",
"src/helper.ts"
]
Include
The include
property specifies an array of glob patterns to include in the compilation.
"include": [
"src/**/*.ts"
]
Exclude
The exclude
property specifies an array of glob patterns to exclude from the compilation. By default, node_modules
is excluded.
"exclude": [
"node_modules",
"dist"
]
References
The references
property is used for project references in a monorepo or multi-project setup. This feature allows you to split a large TypeScript project into smaller, manageable projects.
Example of Using References
Suppose you have two projects: core
and app
. You want app
to reference core
.
-
Core Project
tsconfig.json
:{ "compilerOptions": { "composite": true, "outDir": "./dist" }, "include": ["src"] }
-
App Project
tsconfig.json
:{ "compilerOptions": { "outDir": "./dist" }, "references": [ { "path": "../core" } ], "include": ["src"] }
Advanced Configuration
For more advanced use cases, TypeScript allows deep customization of the tsconfig.json
file.
Using Extends
The extends
property is used to inherit configurations from another tsconfig.json
file. This is useful for sharing a common base configuration across multiple projects.
{
"extends": "./base-tsconfig.json",
"compilerOptions": {
"outDir": "./dist"
},
"include": ["src"]
}
Type Acquisition
The typeAcquisition
property allows you to configure automatic acquisition of type definitions for JavaScript projects.
{
"typeAcquisition": {
"enable": true,
"include": ["jest"],
"exclude": ["node"]
}
}
Plugins
The plugins
property allows you to configure language service plugins.
{
"compilerOptions": {
"plugins": [
{
"name": "typescript-styled-plugin",
"tags": ["styled"]
}
]
}
}
Real-World Examples
Example 1: Basic Project Setup
Here’s a basic example for a typical TypeScript project:
{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true
},
"include": ["src"]
}
Example 2: React Project Setup
For a React project using TypeScript, you might use:
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"noFallthroughCasesInSwitch": true,
"module": "esnext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"jsx": "react-jsx"
},
"include": ["src"]
}
Example 3: Node.js Project Setup
For a Node.js project, you might use:
{
"compilerOptions": {
"target": "es2017",
"module": "commonjs",
"outDir": "./dist",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true
},
"include": ["src"],
"exclude": ["node_modules", "**/*.spec.ts"]
}
Wrapping up
The tsconfig.json
file is a powerful tool for configuring your TypeScript projects. By understanding its structure and key properties, you can tailor your TypeScript environment to meet the specific needs of your project. Whether you're working on a simple single-file project or a complex monorepo, mastering the tsconfig.json
file will enhance your development workflow and improve the quality of your code.
For further details on each property, refer to the official TypeScript tsconfig.json
documentation.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
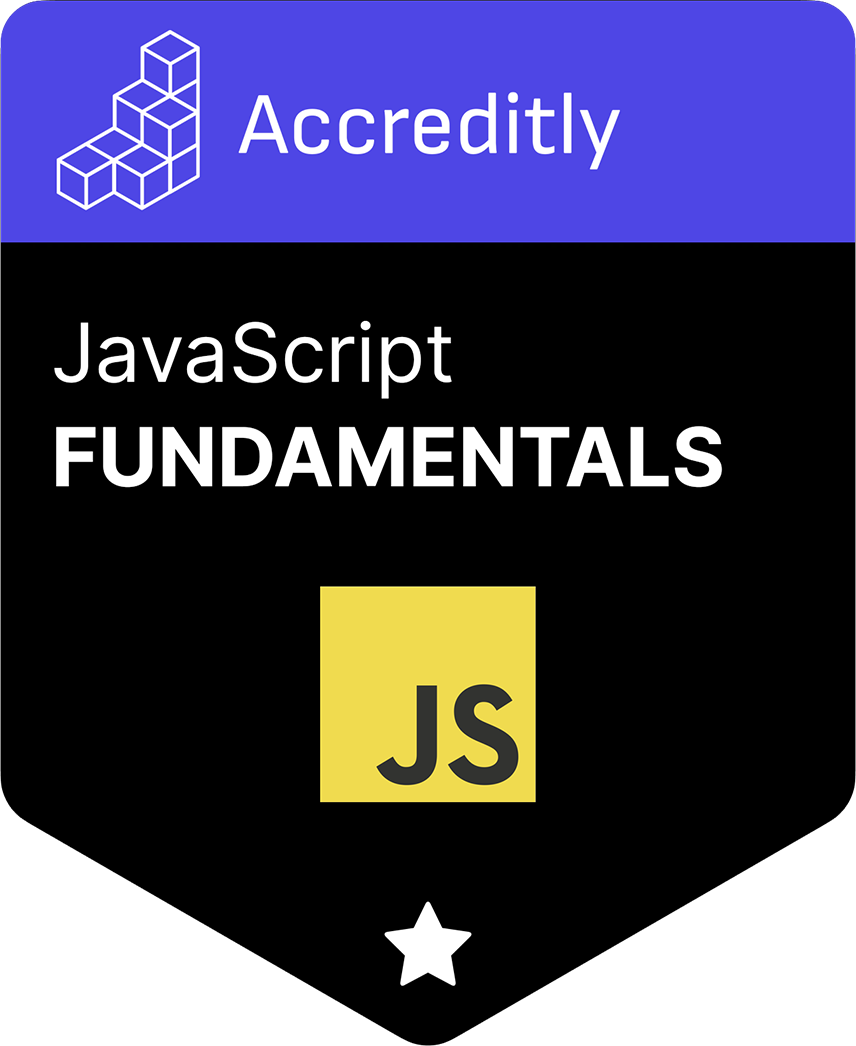