- Creating Temporary Files
- Working with Uploaded Files
- Cleaning Up Temporary Files
- Security Considerations
In the development of web applications with Laravel, handling temporary files is a common requirement. Temporary files can be used for various purposes such as generating on-the-fly reports, processing large data sets, or even storing files uploaded by users before moving them to a permanent location. Proper management of these files is crucial for maintaining application performance, ensuring security, and preventing disk space from being unnecessarily consumed. This article explores best practices for managing temporary files in Laravel, including creation, usage, and cleanup.
Creating Temporary Files
Laravel provides several ways to create and work with temporary files, leveraging PHP's native functions and Laravel's file storage capabilities.
Using PHP’s Native Functions
You can create a temporary file using PHP’s tmpfile()
function, which creates a temporary file in the system's temporary directory.
$tempFile = tmpfile();
fwrite($tempFile, 'Hello, Laravel!');
rewind($tempFile);
Remember, a file created with tmpfile()
is automatically removed when closed (using fclose()
), or when the script ends.
Laravel’s Storage Facade
For more flexibility, including specifying the directory for your temporary files within Laravel's filesystems, use the Storage facade. First, ensure you've configured a disk for temporary files in config/filesystems.php
.
'temp' => [
'driver' => 'local',
'root' => storage_path('app/temp'),
],
You can then use Laravel’s Storage facade to create and manage temporary files.
use Illuminate\Support\Facades\Storage;
Storage::disk('temp')->put('example.txt', 'Hello, Laravel!');
Working with Uploaded Files
When handling file uploads in Laravel, you might not immediately move the uploaded file to its permanent location. Laravel’s uploaded file class has a store()
method that can be used to move the file to a temporary disk.
$request->file('photo')->store('uploads', 'temp');
Cleaning Up Temporary Files
Managing the lifecycle of temporary files is critical. You should remove these files as soon as they're no longer needed to free up disk space and maintain application efficiency.
Manual Deletion
After processing a temporary file, you can delete it using Laravel’s Storage facade.
Storage::disk('temp')->delete('example.txt');
Automatic Cleanup
For a more automated approach to cleaning up old temporary files, consider scheduling a task in Laravel’s console kernel. This task can run at regular intervals, removing temporary files older than a certain threshold.
// app/Console/Kernel.php
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
collect(Storage::disk('temp')->allFiles())->each(function ($file) {
if (now()->diffInHours(Storage::disk('temp')->lastModified($file)) >= 24) {
Storage::disk('temp')->delete($file);
}
});
})->daily();
}
This example removes files in the temporary disk that are older than 24 hours.
Security Considerations
Managing temporary files securely is paramount. Ensure sensitive data is not exposed through temporary files, especially when handling user uploads or generating files with confidential information.
- Always store temporary files in a non-publicly accessible directory.
- Consider encrypting temporary files containing sensitive data.
- Regularly audit and monitor access to temporary files.
Efficiently managing temporary files in Laravel not only helps in maintaining application performance but also plays a critical role in ensuring data security. Whether you’re handling user uploads, generating reports, or processing data, Laravel provides a robust set of tools for managing the lifecycle of temporary files. Remember, the key to effective temporary file management lies in understanding when and how to create, use, and most importantly, clean up these files. Implementing automated cleanup tasks and adhering to security best practices will keep your Laravel application running smoothly and securely.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
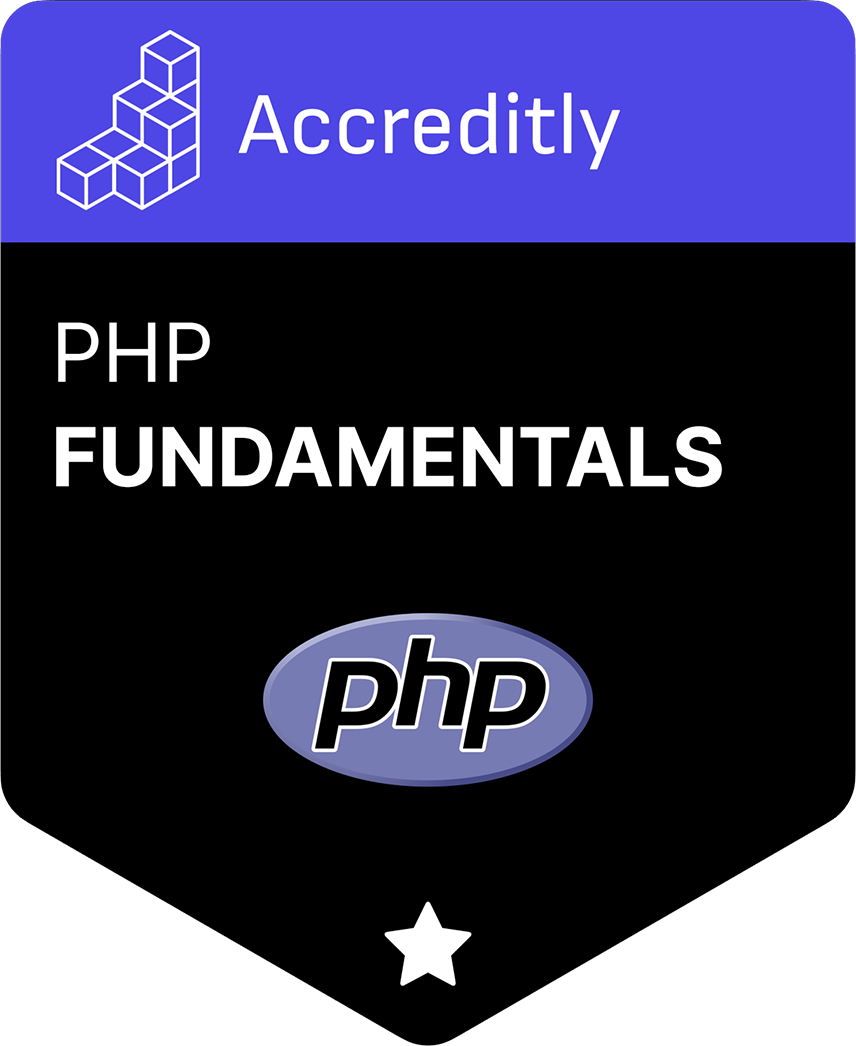