- Setting up Stripe
- Creating a Plan
- Creating a Customer
- Creating a Subscription
- Managing Subscriptions
- Implementing
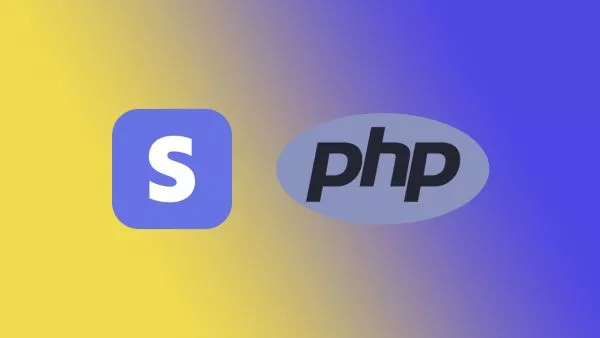
The Stripe PHP SDK provides a simple and robust solution for managing subscription payments in your web application. This article will guide you through setting up Stripe, creating subscription plans, and managing subscriptions.
Before you start, ensure you have installed the Stripe PHP SDK using composer: composer require stripe/stripe-php
Setting up Stripe
To use Stripe's PHP SDK, you need to set your secret key which is available from your Stripe dashboard.
\Stripe\Stripe::setApiKey('your-secret-key');
We always recommend storing keys in environment (.env
) variables, which are kept outside of source control.
Creating a Plan
Subscription billing requires a plan to which your customers can subscribe. You can create a plan as follows:
$plan = \Stripe\Plan::create([
'amount' => 2000,
'interval' => 'month',
'product' => ['name' => 'Monthly Premium Plan'],
'currency' => 'usd',
]);
In the above code, the amount is in the smallest currency unit (cents), and the interval is the frequency of billing.
You can also create Plans and Prices inside the Stripe dashboard. Stripe will give you the relevant IDs.
You'll need to store these somewhere so you can use them to create a subscription later on.
Creating a Customer
Before creating a subscription, you need to create a customer:
$customer = \Stripe\Customer::create([
'description' => 'customer for [email protected]',
'email' => '[email protected]',
'payment_method' => 'pm_card_visa',
]);
Here, a new customer is created with a description, email, and a payment method.
Creating a Subscription
With the plan and customer set up, you can create a subscription:
$subscription = \Stripe\Subscription::create([
'customer' => $customer->id,
'items' => [['plan' => $plan->id]],
'expand' => ['latest_invoice.payment_intent'],
]);
This creates a subscription for the customer to the specified plan.
Managing Subscriptions
You can retrieve a customer's subscriptions as follows:
$subscriptions = \Stripe\Subscription::all(['customer' => $customer->id]);
To cancel a subscription:
$subscription = \Stripe\Subscription::retrieve('replace_with_subscription_id');
$subscription->cancel();
Implementing
The below snippets show you how to create a plan, customer and then a subscription. You can then view all subscriptions associated with a customer and cancel them. You'll need to store all relevant IDs within your own database in order to follow through the process.
Additionally, you'll want to consider implementing webhooks for state changes in the subscription. Subscriptions, by their nature, will change without the customer being there. For example, if the renewal fails because of insufficient funds, you need to update your systems to prevent access. It's possible to do this by polling the API at each billing cycle, but the better way to do it is using webhooks, whereby Stripe will inform you of any changes.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
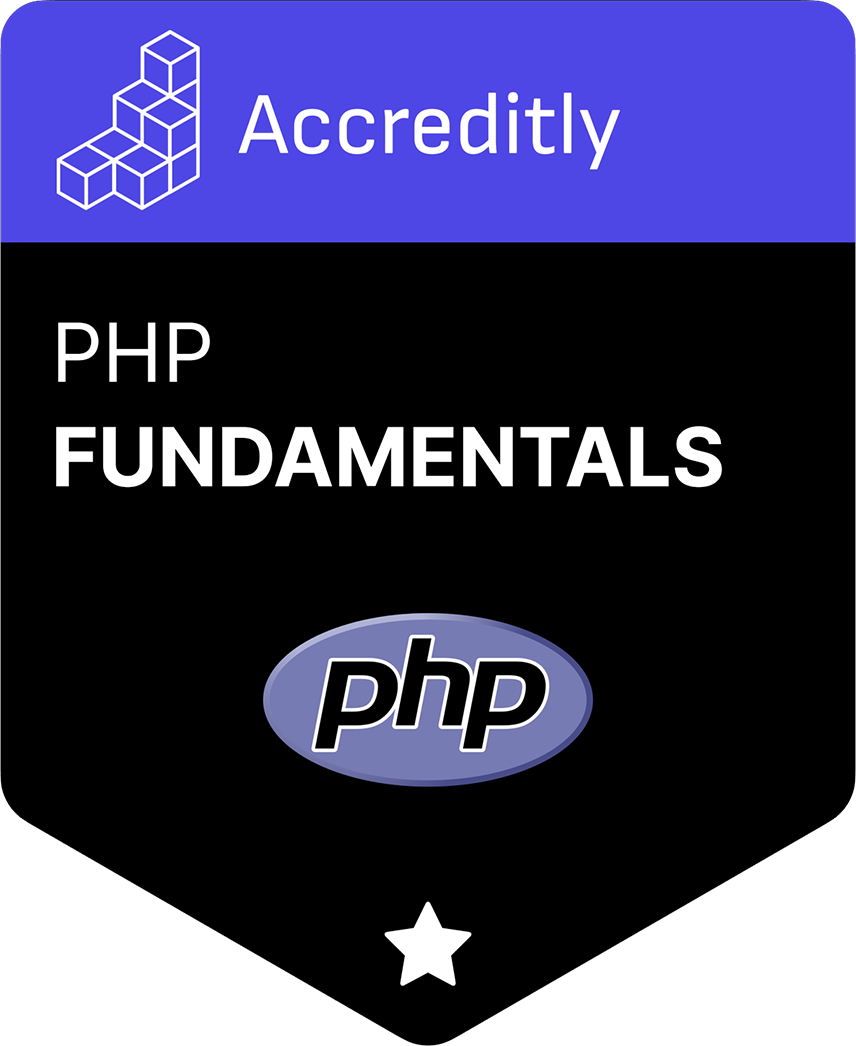
Related articles
Tutorials WordPress Database Design Tooling
How to store and output currency values in WordPress
Effectively manage currency values in WordPress, from storage best practices to displaying currency amounts on your website. This guide covers everything to ensure accurate and user-friendly currency handling in WordPress.